Getting Started with Lists
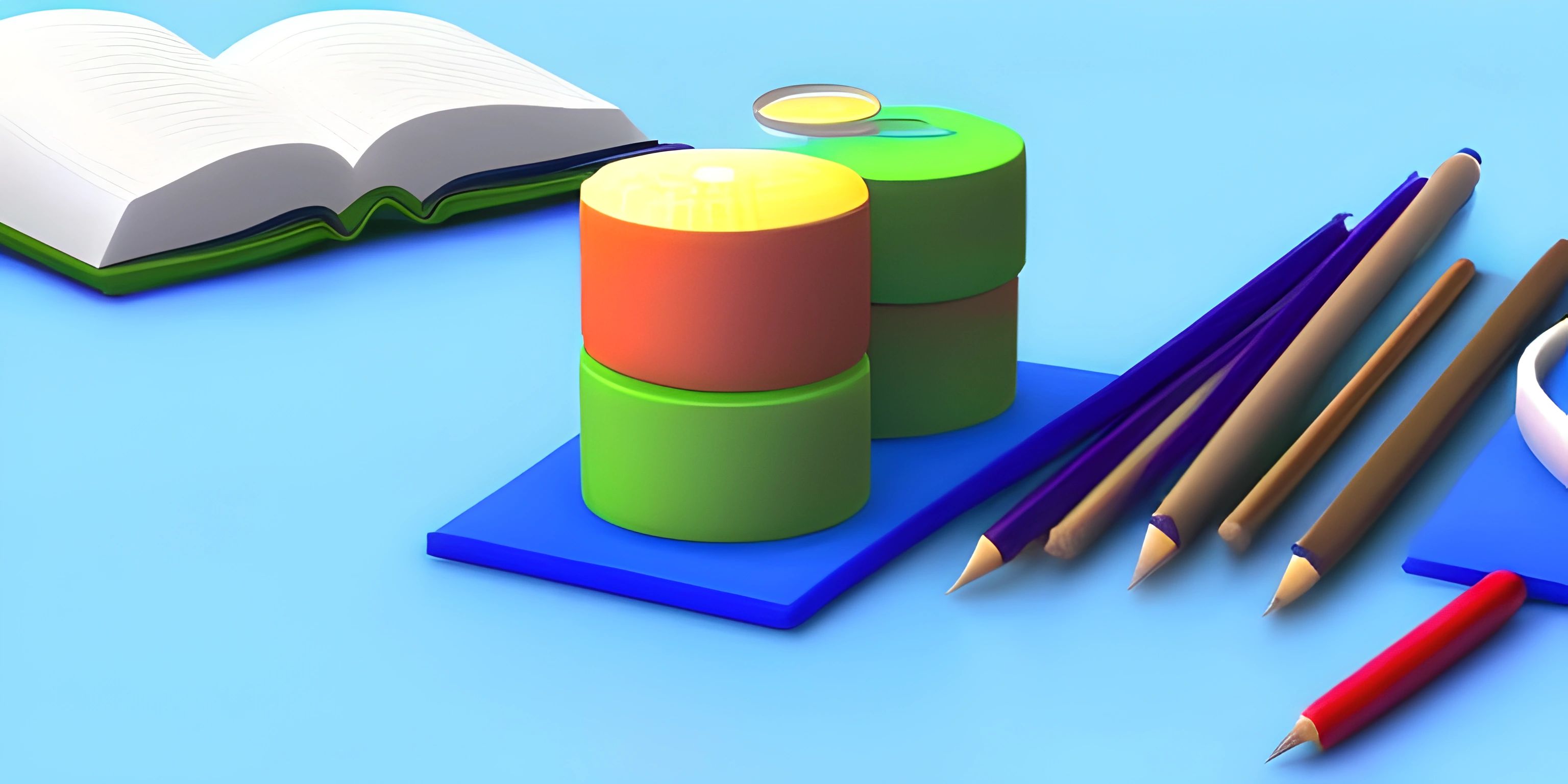
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every programmer must inevitably deal with a collection of items at some point. Whether you're managing an inventory in a game or storing names of people attending a party, having a way to organize and manipulate groups of data is crucial. This is where lists come to the rescue!
What is a list?
A list, also known as an array in some languages, is an ordered collection of elements. Elements within a list are stored in a specific order, and each element can be accessed using its index (position) within the list.
For instance, imagine a shopping list:
1. Apples 2. Bananas 3. Cereal 4. Milk
Here, each item is an element of the list, and they're ordered by their position (index) in the list.
Creating a list
In most programming languages, creating a list is straightforward. Here's an example in Python:
my_shopping_list = ["Apples", "Bananas", "Cereal", "Milk"]
And here's the same list in JavaScript:
let myShoppingList = ["Apples", "Bananas", "Cereal", "Milk"];
As you can see, the syntax for creating a list varies between languages, but the concept remains the same: a collection of items enclosed in brackets and separated by commas.
Accessing elements
To access an element in a list, we'll use its index within the list. Keep in mind that most programming languages use zero-based indexing, meaning the first element is at index 0, the second at index 1, and so on.
Here's how we can access the first element of our shopping list in Python:
first_item = my_shopping_list[0] # Access the first element, "Apples"
And the same action in JavaScript:
let firstItem = myShoppingList[0]; // Access the first element, "Apples"
Modifying elements
Changing an element in a list is similar to accessing it. We just need to use the index of the element we want to change and assign it a new value. Here's how we can change the first element of our shopping list to "Oranges" in Python:
my_shopping_list[0] = "Oranges" # Change the first element to "Oranges"
And the same modification in JavaScript:
myShoppingList[0] = "Oranges"; // Change the first element to "Oranges"
Common list operations
Programming languages offer a wide range of operations to manipulate lists. Some common operations include:
- Appending elements: Add a new element to the end of the list.
- Inserting elements: Add a new element at a specific index within the list.
- Removing elements: Remove an element from the list.
- Finding elements: Search for an element in the list and retrieve its index.
- Sorting elements: Rearrange the elements in the list in a specific order (e.g., ascending or descending).
The exact syntax for these operations varies between languages, but understanding their purpose and usage is fundamental to working with lists.
Now that you're familiar with the basics of lists, you're ready to embark on your programming journey with a powerful tool by your side. Remember to consult your chosen programming language's documentation for specific list implementations and operations. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What are lists and why are they important in programming?
Lists are a fundamental data structure in programming that allow you to store and manage multiple items in an ordered and mutable collection. They are important because they enable you to handle a dynamic number of elements, simplify code, and perform complex operations with ease.
How do I create a list in Python?
To create a list in Python, you can use square brackets []
and separate the elements with commas. Here's an example:
my_list = [1, 2, 3, 4, 5]
Can a list contain different data types?
Yes, a list can contain elements of different data types, including other lists! Here's an example:
mixed_list = [1, "hello", 3.14, [1, 2, 3]]
How do I access elements in a list?
You can access elements in a list by their index, starting from 0 for the first element. For example, to access the second element in a list, you would use:
my_list = [1, 2, 3, 4, 5] second_element = my_list[1]
How do I add or remove elements from a list?
You can use the append()
method to add an element to the end of a list, and the remove()
method to remove an element by its value. Here's an example:
my_list = [1, 2, 3, 4, 5] # Add an element my_list.append(6) # Remove an element my_list.remove(3)