Arrays in Programming
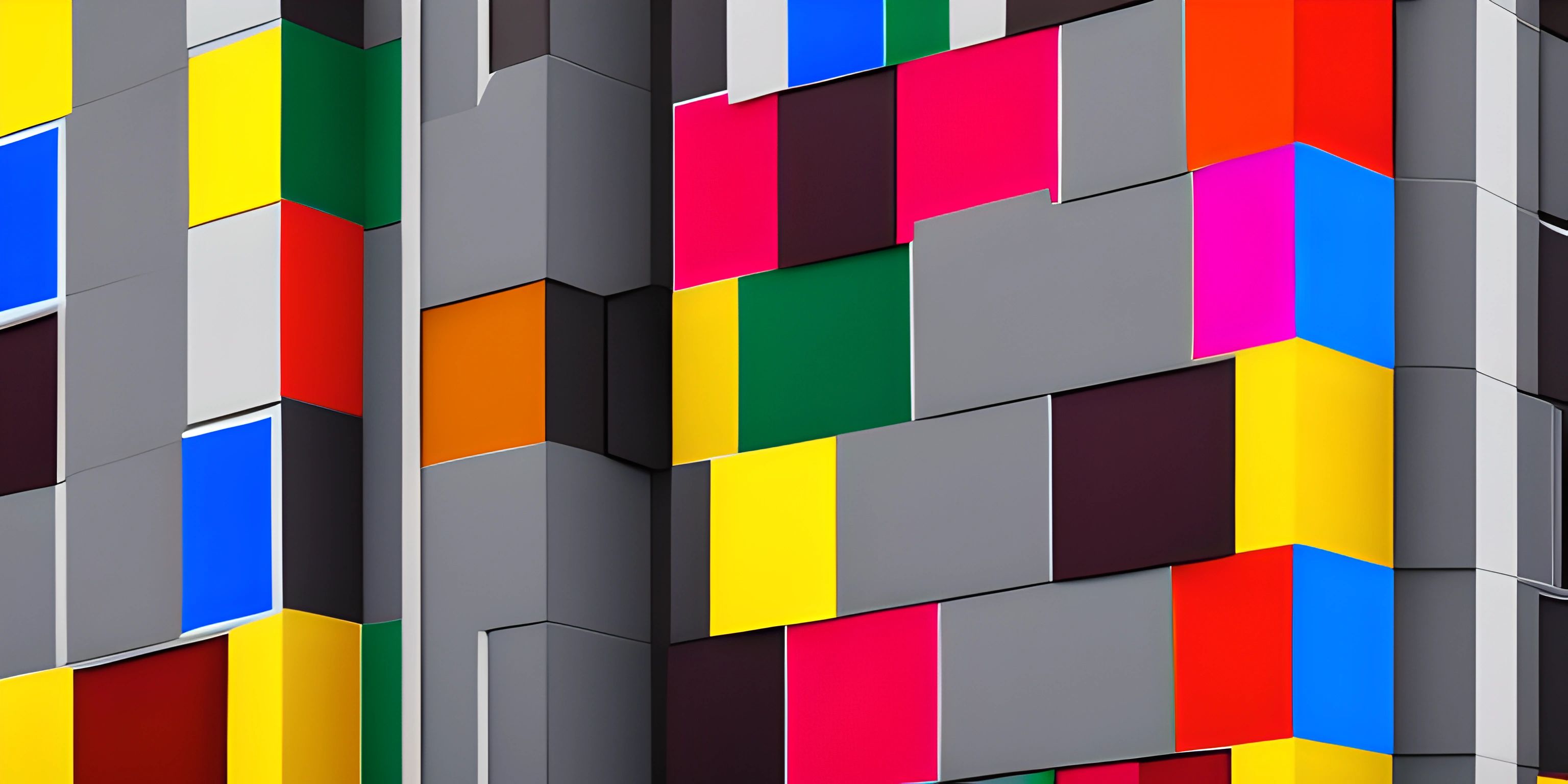
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Arrays are like the utility drawers in your kitchen. They hold multiple items in an organized manner, and you can easily access and manipulate those items when needed. In programming, arrays are essential data structures that can store multiple values in a single, ordered collection. Let's dive into arrays, their properties, and how they're used in different programming languages.
What is an Array?
An array is a collection of elements, where each element is identified by an index (a numerical position within the array). The elements within an array can be of the same data type or different data types, depending on the programming language being used.
Here's a simple analogy to help you grasp the concept of arrays: Imagine a row of lockers in a school. Each locker can store an item, and each locker has a number (index) to identify its position in the row.
One-Dimensional Arrays
A one-dimensional array is like the row of lockers mentioned earlier – it has a single set of elements with an index for each element. Here's an example of a one-dimensional array that stores five integers:
numbers = [10, 20, 30, 40, 50]
Multi-Dimensional Arrays
A multi-dimensional array is like a matrix or a grid of elements, where each element can be accessed using multiple indices. Essentially, it's an array of arrays. Here's an example of a two-dimensional array that stores a 3x3 grid of integers:
matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]
Operations on Arrays
Arrays can be manipulated in various ways, such as adding, removing, or updating elements. Some common operations on arrays are:
- Access: Retrieve the value at a specific index.
- Insert: Add an element at a specific index.
- Update: Modify the value of an element at a specific index.
- Delete: Remove an element at a specific index.
- Length: Determine the number of elements stored in the array.
- Search: Find the index of an element with a specific value.
Accessing Elements in an Array
To access an element in an array, you simply use its index. Remember, array indices start at 0, so the first element is at index 0, the second at index 1, and so on. Here's an example in Python:
numbers = [10, 20, 30, 40, 50] # Access the second element (index 1) second_number = numbers[1] print(second_number) # Output: 20
Modifying Elements in an Array
To change the value of an element in an array, you assign a new value to the element using its index. Here's an example:
numbers = [10, 20, 30, 40, 50] # Change the value of the third element (index 2) numbers[2] = 100 print(numbers) # Output: [10, 20, 100, 40, 50]
Arrays in Different Programming Languages
The syntax and features of arrays may vary slightly between programming languages. Let's take a look at arrays in some popular languages:
Python
In Python, arrays are called lists. They can store elements of different data types and are dynamically resizable. You can learn more about Python lists in this guide.
JavaScript
JavaScript uses arrays to store elements of different data types. They are also dynamically resizable. Check out this article to learn more about arrays in JavaScript.
Java
In Java, arrays are fixed in size and can only store elements of the same data type. To create dynamic arrays, you can use the ArrayList
class. Here's a tutorial on arrays and ArrayLists in Java.
Now that you have a good understanding of arrays, you can confidently start using them in your programming projects. Keep experimenting and exploring the various operations and features of arrays in different languages to become a master of this essential data structure. Good luck, and happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What is an array in programming?
An array is a data structure in programming that allows you to store multiple values of the same data type in a single variable. It's like a container that holds a fixed number of elements, making it easier to manage and access data. Arrays are particularly useful when you need to work with a collection of similar data items, such as a list of names, numbers, or colors.
How do I declare and initialize an array in programming languages?
Declaring and initializing an array can vary depending on the programming language you're using. Here are some examples in different languages:
// JavaScript let numbers = [1, 2, 3, 4, 5];
# Python numbers = [1, 2, 3, 4, 5]
// Java int[] numbers = {1, 2, 3, 4, 5};
// C# int[] numbers = new int[] {1, 2, 3, 4, 5};
How do I access elements in an array?
To access elements in an array, you use the index of the element you want to access. Array indices usually start at 0, so the first element is at index 0, the second element is at index 1, and so on. Here's an example in JavaScript:
let names = ["Alice", "Bob", "Charlie"]; console.log(names[0]); // Output: "Alice" console.log(names[1]); // Output: "Bob" console.log(names[2]); // Output: "Charlie"
How do I loop through the elements of an array?
You can use various types of loops to iterate through the elements of an array. Here's an example using a for
loop in Python:
colors = ["red", "green", "blue"] for color in colors: print(color)
In this example, the for
loop iterates through each element in the colors
array and prints it out.
Can arrays have different data types within the same array?
In most programming languages, arrays are designed to store elements of the same data type. However, some languages, like JavaScript and Python, allow you to store elements of different data types in the same array. For example:
// JavaScript let mixedArray = [1, "two", 3.0, true];
# Python mixed_array = [1, "two", 3.0, True]
Keep in mind that arrays with mixed data types can be harder to work with and may lead to unexpected behavior. It's generally recommended to use arrays to store elements of the same data type.