Creating Art with Python and Processing
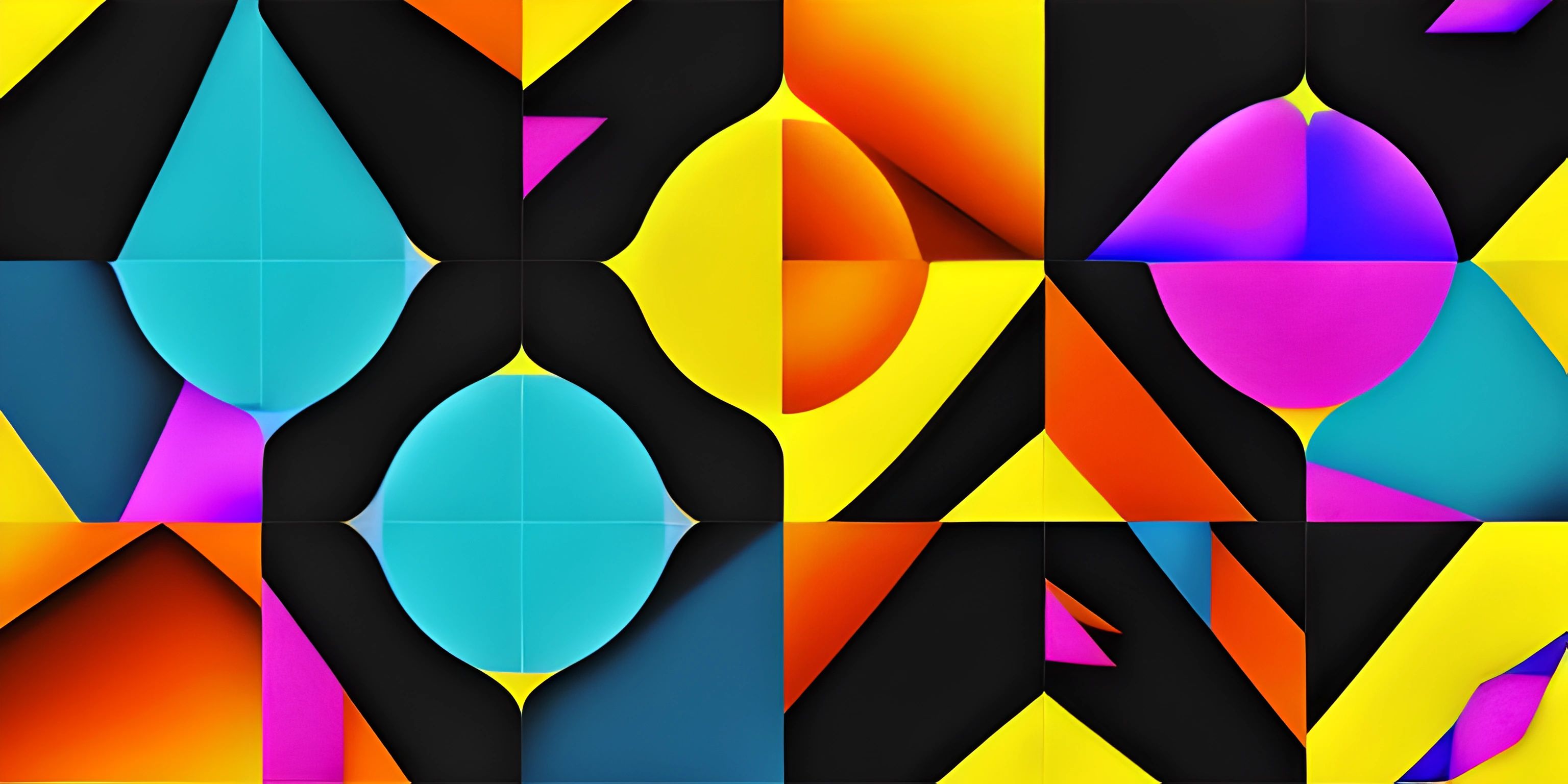
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Visual art and programming may seem like an unlikely pair, but with the help of Python and the Processing library, you can create stunning pieces of art with code. In this guide, we'll explore how to use Python and Processing to unleash your creative potential and generate eye-catching visuals.
What is Processing?
Processing is an open-source programming library and development environment for creating visual art, interactive graphics, and animations. It is designed to make it easy for artists, designers, and programmers to create visually rich, interactive applications. The Processing library simplifies complex graphics programming tasks and offers a vast collection of built-in functions to create and manipulate visuals.
Setting up Python and Processing
Before diving into the world of artistic coding, we need to set up our environment. In this guide, we will use the Python mode in Processing, which allows us to write our code using the Python language.
- Download and install Processing.
- Open Processing and go to
Tools
>Add Tool
>Modes
>Python Mode for Processing
. - Click on "Install" and wait for it to complete.
- Select Python Mode from the drop-down menu in the top right corner of the Processing IDE.
Now that our environment is set up, let's start exploring the capabilities of Processing.
Drawing Shapes
Processing provides a straightforward way to draw shapes on the screen. Let's begin with a simple example – drawing a circle.
def setup(): size(800, 800) def draw(): background(255) fill(200, 0, 0) ellipse(400, 400, 200, 200)
In this code, we define two essential functions: setup()
and draw()
. The setup()
function is called once when the program starts, and we use it to set the size of the canvas to 800x800 pixels. The draw()
function is executed repeatedly, and we use it to draw our shapes.
We start by setting the background color to white using background(255)
. We then set the fill color of the circle using the fill()
function. The ellipse()
function draws a circle with a diameter of 200 pixels at the coordinates (400, 400).
Animating Shapes
To create an animation, we can update the position, size, or color of our shapes within the draw()
function. Let's move the circle horizontally by incrementing its x-coordinate.
pos_x = 400 def setup(): size(800, 800) def draw(): global pos_x background(255) fill(200, 0, 0) ellipse(pos_x, 400, 200, 200) pos_x += 1
We declare a global variable pos_x
to store the x-coordinate of the circle. Inside the draw()
function, we increment pos_x
by 1 on each iteration, making the circle move to the right.
Using Loops and Arrays
To create more complex visuals, we can use loops and arrays to draw multiple shapes with different properties. Let's draw a grid of squares with random colors.
from random import randint def setup(): size(800, 800) def draw(): background(255) for i in range(8): for j in range(8): fill(randint(0, 255), randint(0, 255), randint(0, 255)) rect(100 * i, 100 * j, 100, 100)
Using nested for
loops, we iterate over a grid of 8x8 squares. We set a random color for each square using the fill()
function and the randint()
function from the random
module. Finally, we draw each square using the rect()
function.
Conclusion
This guide has just scratched the surface of what you can do with Python and Processing. The possibilities are endless, and you can create mesmerizing animations, interactive art, or even games using these tools. As you continue to explore and experiment, you'll unlock the full potential of creative coding and bring your artistic vision to life.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).