Creating Artwork with Java and Processing
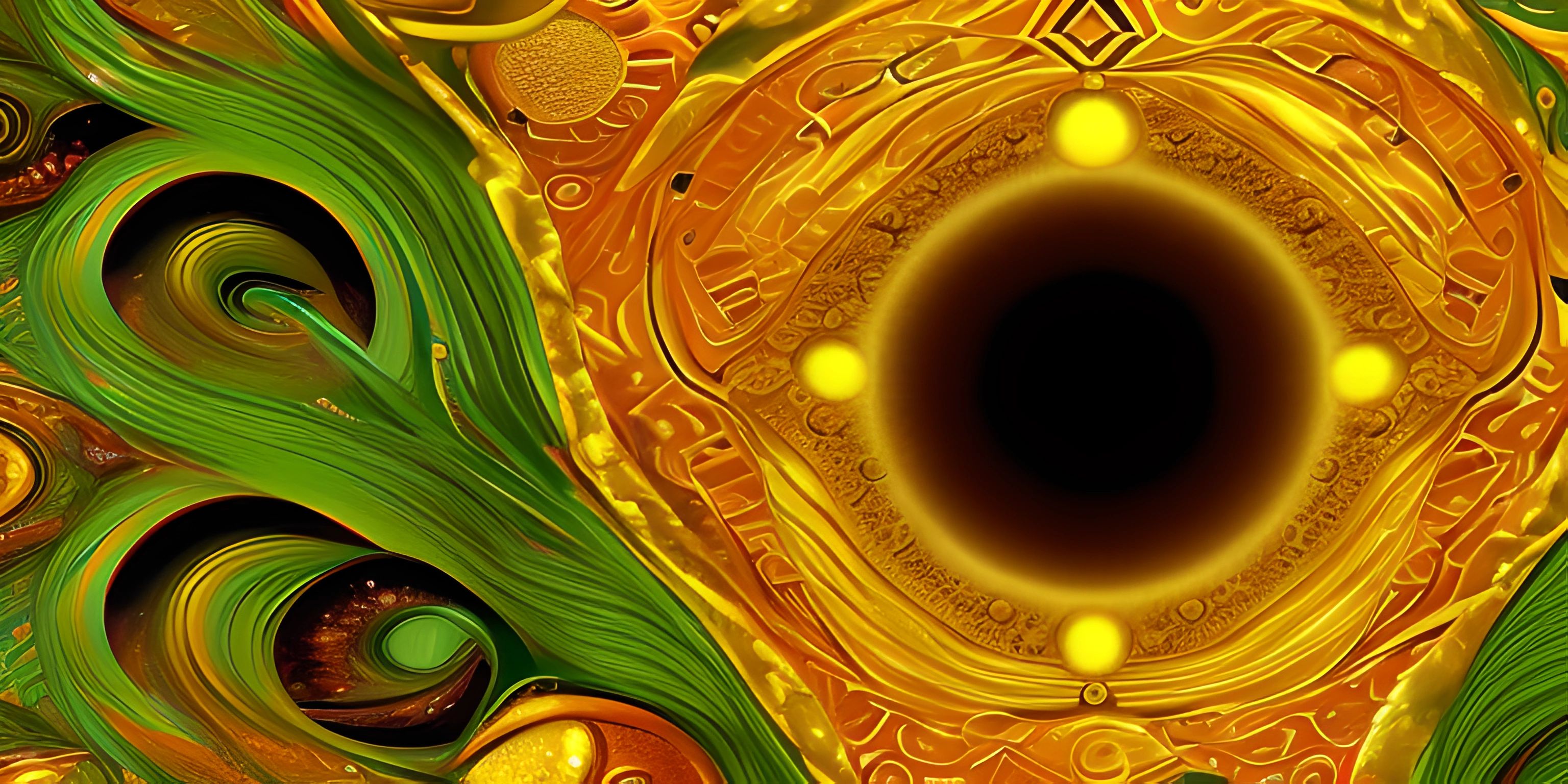
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming isn't just about writing complex algorithms or building web applications. It can also be a medium for artistic expression! By using the Processing library in Java, we can create visually stunning artwork with ease.
Setting Up Processing
Before we dive into creating our masterpiece, let's set up Processing in our Java project. You can download the library from the official Processing website. Once you have it, add it as a dependency in your Java project. If you're using an IDE like Eclipse or IntelliJ, this should be as simple as adding the Processing JAR file to your project's classpath.
Creating a Basic Sketch
In Processing, a piece of artwork is called a "sketch." To create a sketch, you'll need to extend the PApplet
class, which contains all the methods and functionality you'll need to create your visual art. Here's a basic example:
import processing.core.*; public class MySketch extends PApplet { public static void main(String[] args) { PApplet.main("MySketch"); } public void settings() { size(800, 600); } public void setup() { background(255); } public void draw() { // Your artwork code goes here } }
This basic setup gives us a blank canvas of 800 by 600 pixels to work with. The settings()
method is where we set the dimensions of our canvas, and the setup()
method initializes the background color. The draw()
method is where the magic happens – this is where we'll write the code to create our artwork.
Drawing Shapes
Processing provides a wide range of methods for drawing shapes, such as ellipse()
, rect()
, and line()
. Let's start by drawing a simple circle in the middle of our canvas:
public void draw() { fill(0, 0, 255); // Set the fill color to blue ellipse(width / 2, height / 2, 100, 100); // Draw a circle with a diameter of 100 pixels }
This will draw a blue circle in the center of our canvas. The fill()
method sets the color that will be used to fill in the shapes we draw. The ellipse()
method is used to draw a circle or an ellipse, with the first two parameters specifying the center coordinates and the last two parameters defining the width and height.
Adding Interactivity
One of the coolest things about Processing is how easy it is to add interactivity to your sketches. Let's say we want the circle's color to change when we click on it. We can do that by overriding the mousePressed()
method:
public void mousePressed() { if (dist(mouseX, mouseY, width / 2, height / 2) < 50) { fill(random(255), random(255), random(255)); } }
Here, we're using the dist()
method to calculate the distance between the mouse cursor and the center of the circle. If the distance is less than 50 pixels (meaning we've clicked inside the circle), we change the fill color to a random color using the random()
method.
Embrace Your Inner Artist
We've just scratched the surface of what's possible with Java and Processing. There's a whole world of possibilities waiting for you to explore, from creating generative art and animations to building interactive installations. So, unleash your creativity and start experimenting with this powerful library to create your own unique and captivating artwork. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reading Data Files (psst, it's free!).
FAQ
What is the Processing library in Java?
The Processing library is an open-source graphics library and integrated development environment (IDE) built for the visual arts and electronic arts communities. It simplifies programming and enhances visual creativity by providing a set of powerful tools and functions for creating visually rich and interactive digital art pieces using Java.
How do I get started with Processing in Java?
To get started with Processing in Java, you'll need to follow these steps:
- Download and install the Processing IDE from the official website (https://processing.org/download/).
- Open the Processing IDE and create a new project.
- Add the necessary import statements for using the Processing library, such as
import processing.core.PApplet;
. - Extend your main class with
PApplet
. - Implement the
settings()
anddraw()
methods, which are essential for creating artwork with Processing. - Run your project and observe the results.
What are the main methods used in creating artwork with Processing and Java?
The two main methods used in creating artwork with Processing and Java are:
settings()
: This method is used to set up the canvas size, rendering mode, and other initial configurations for your project.draw()
: This method is where all the magic happens. It contains the code responsible for rendering the shapes, colors, and animations on the canvas.
Can I use Processing with other programming languages?
Yes! Although Processing was initially designed to work with Java, it has since expanded to include support for other programming languages, such as Python and JavaScript. These adaptations of the Processing library are called "Processing.py" and "p5.js" respectively. Each version retains the core concepts and functionality, allowing artists and developers to create visually stunning artwork across multiple programming languages.
What are some common Processing functions for creating shapes and colors?
Some common Processing functions for creating shapes and colors include:
background()
: Sets the background color of the canvas.fill()
: Sets the color used to fill shapes.stroke()
: Sets the color used for drawing lines and borders around shapes.rect()
: Draws a rectangle on the canvas.ellipse()
: Draws an ellipse (circle or oval) on the canvas.line()
: Draws a line between two points on the canvas.color()
: Creates a color value based on the input parameters (RGB, HSB, etc.). These are just a few examples of the many functions available in the Processing library. You can mix and match these functions to create a wide variety of shapes, colors, and patterns for your digital artwork.