Data Types
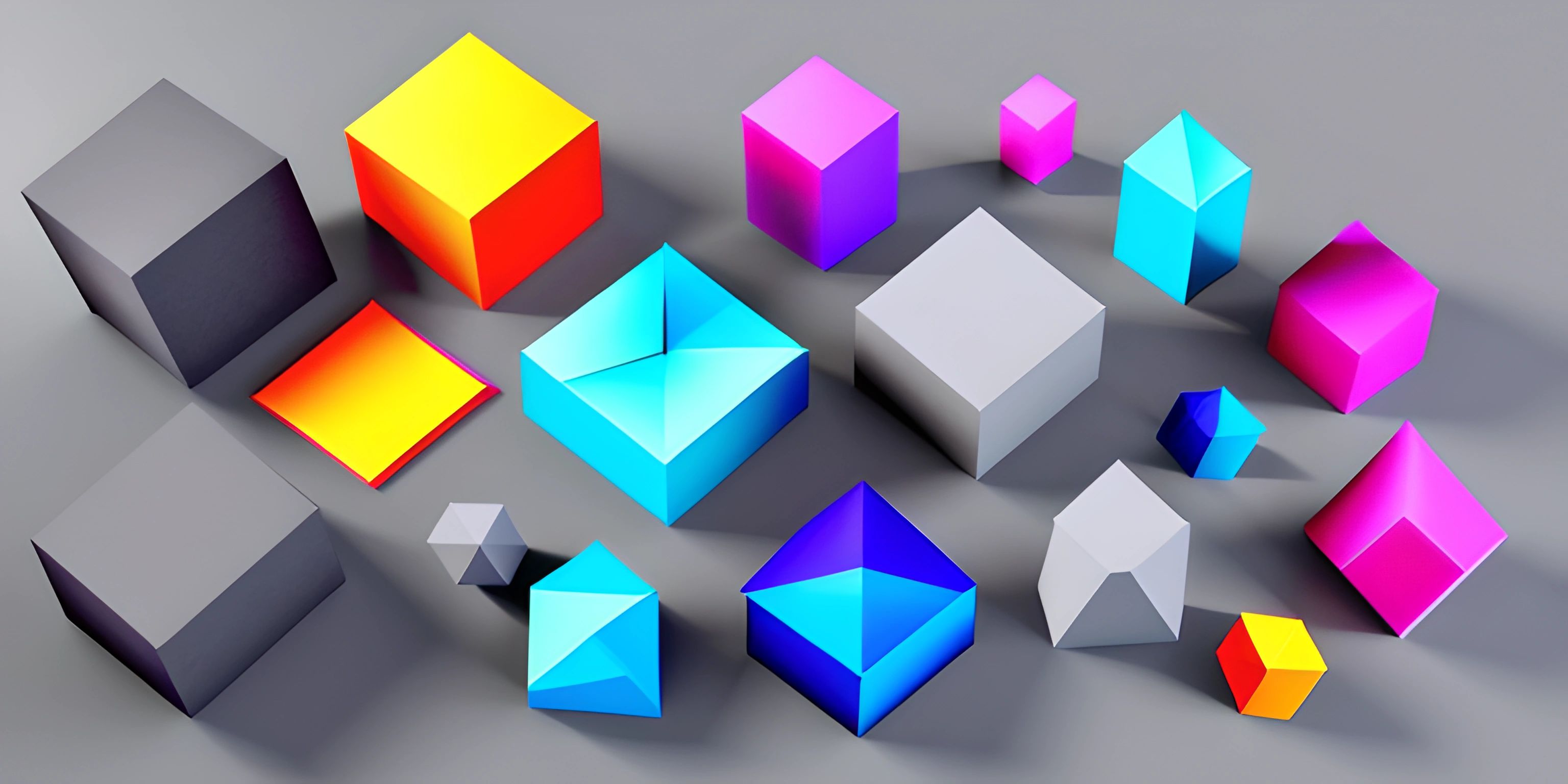
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Every programming language comes with a variety of data types, which are simply different ways of representing information. Just as we use different containers to hold various things in real life (like a glass for water and a bowl for soup), data types allow us to store and manipulate all sorts of data in our programs.
Basic Data Types
Let's take a look at some of the most common data types you'll encounter while programming, along with examples in various languages.
Integer
An integer is a whole number, without any decimal points or fractions. It can be positive, negative, or zero. In many languages, integers are represented using the keyword int
. Here's an example in Python:
age = 25
Float
A float, short for "floating-point number," is a number with a decimal point. It can be used to represent fractions and other non-integer values. In many languages, floats are represented using the keyword float
. Here's an example in JavaScript:
temperature = 72.5
String
A string is a sequence of characters, like words or sentences. Strings are typically enclosed in double quotes ("
) or single quotes ('
). Here's an example in Ruby:
greeting = "Hello, world!"
Boolean
A boolean is a data type that can only have two values: true
or false
. Booleans are used to represent conditions, like whether a light is on or off. Here's an example in Java:
boolean isOn = false;
Collection Data Types
Sometimes, we need to group multiple values together. That's where collection data types come in!
Array
An array is an ordered collection of elements, which can be of any data type. Arrays are indexed, meaning each element is assigned a unique number called the index. Here's an example in PHP:
$fruits = array("apple", "banana", "cherry");
Object
An object is a collection of key-value pairs, where each key is associated with a value. Objects are useful for organizing and structuring data. Here's an example in JavaScript:
let person = { "name": "Alice", "age": 30, "city": "New York" };
Choosing the Right Data Type
When deciding which data type to use, consider the nature of the data and how you plan to use it. For example, if you need to store a person's age, an integer would be the best choice. If you need to store their name, a string would be more appropriate.
As you delve deeper into the world of programming, you'll encounter more complex data types and custom data structures tailored to specific needs. But for now, understanding these basic data types will serve as a solid foundation for your coding adventures!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are the most common data types in programming?
The most common data types in programming include:
- Integers: Whole numbers like -3, 0, and 42.
- Floating-point numbers: Decimal numbers like 3.14, -0.5, and 2.71828.
- Strings: Sequences of characters like "hello", "world", and "Cratecode".
- Booleans: True or false values, often used for conditional statements.
- Arrays: Collections of elements, which can be of any data type, like [1, 2, 3] or ["apple", "banana", "cherry"]. Different programming languages may have additional types or variations of these basic types, but these are the most common across many languages.
How do I declare a variable with a specific data type?
In many programming languages, you can declare a variable and its data type using a specific syntax. Here's an example in a few different languages:
- Python:
age = 25 # Integer weight = 70.5 # Float name = "John" # String is_student = True # Boolean
- JavaScript:
let age = 25; // Number (integer) let weight = 70.5; // Number (float) let name = "John"; // String let is_student = true; // Boolean
- Java:
int age = 25; float weight = 70.5f; String name = "John"; boolean is_student = true;
Remember that the syntax and rules for declaring variables may vary between programming languages.
Can I change the data type of a variable after it has been declared?
In most programming languages, you can change the data type of a variable after it has been declared, either implicitly or explicitly. Here's a simple example in Python:
number = 42 # Integer print(type(number)) # Output: <class 'int'> number = 42.0 # Float print(type(number)) # Output: <class 'float'>
However, some languages, like Java and C++, use static typing, which means you cannot change the data type of a variable after it has been declared.
How do I convert a variable's data type to another type?
In many programming languages, you can convert a variable's data type using built-in functions or casting. Here's an example in Python, JavaScript, and Java:
- Python:
number = 42 floating_number = float(number) # Convert integer to float string_number = str(number) # Convert integer to string
- JavaScript:
let number = 42; let floating_number = parseFloat(number); // Convert integer to float let string_number = number.toString(); // Convert integer to string
- Java:
int number = 42; float floating_number = (float) number; // Cast integer to float String string_number = Integer.toString(number); // Convert integer to string
Remember to check the documentation of the programming language you're using for the correct method of converting data types.