Selection Sort in Detail
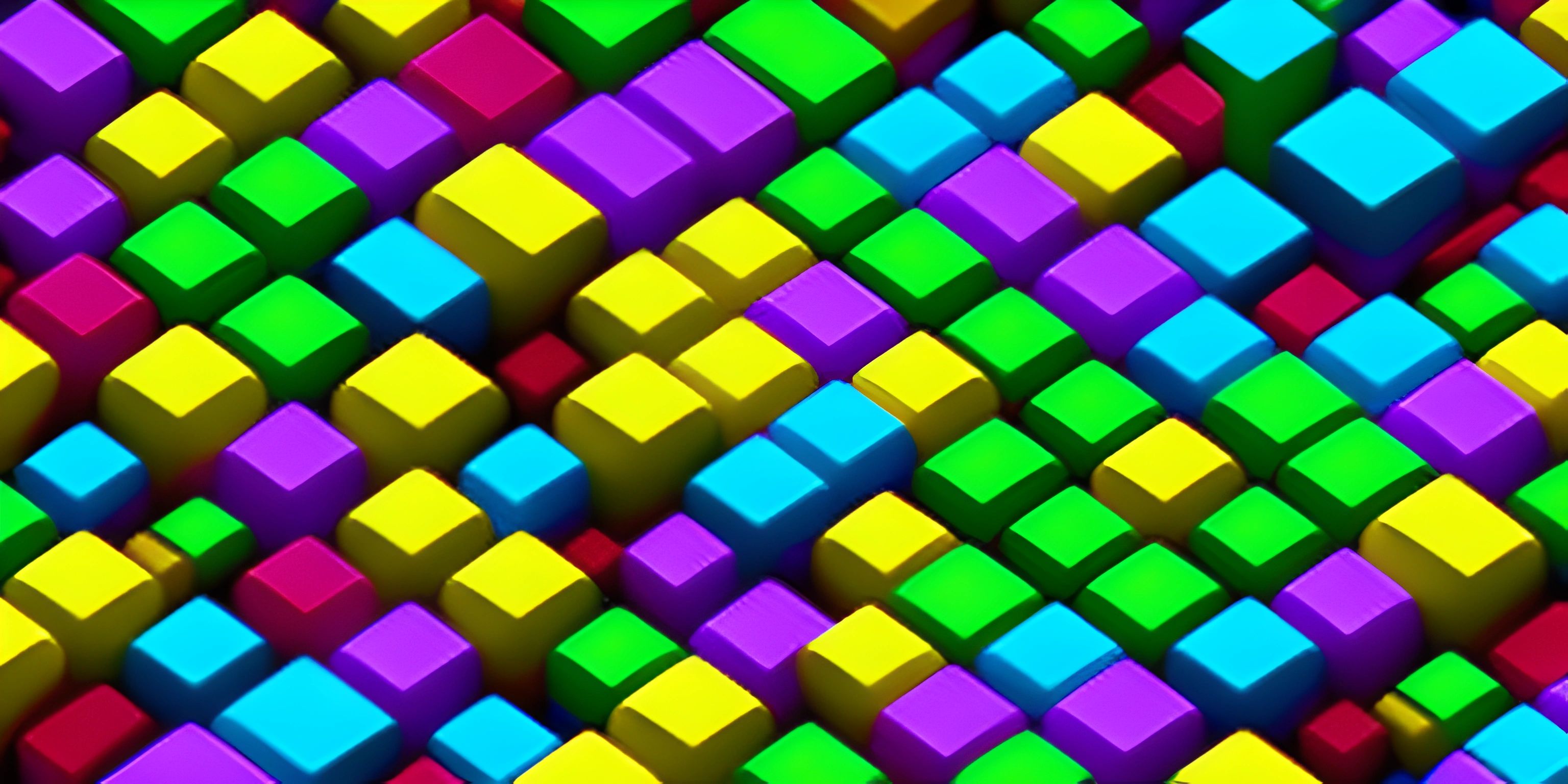
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ah, selection sort! It's like picking the shortest straw among many, then doing it again and again until you have a nice, neat, sorted pile of straws. In this article, we'll dive deep into the world of selection sort, exploring how it works, how to implement it, and when it can be useful.
How Does Selection Sort Work?
Selection sort is an easy-to-understand, yet not-so-efficient sorting algorithm. It is a comparison-based algorithm that works by repeatedly finding the minimum element from the unsorted portion of the array and placing it at the beginning of the sorted portion.
Here's a step-by-step breakdown of how selection sort works:
- Find the smallest element in the array and swap it with the first element.
- Find the smallest element in the remaining unsorted portion of the array and swap it with the second element.
- Continue this process until the entire array is sorted.
Let's see an example using a simple array of numbers: [5, 3, 8, 1, 2]
- The smallest element is
1
. Swap it with the first element:[1, 3, 8, 5, 2]
- The smallest element in the remaining unsorted portion (
[3, 8, 5, 2]
) is2
. Swap it with the second element:[1, 2, 8, 5, 3]
- Repeat the process until the entire array is sorted:
[1, 2, 3, 5, 8]
Implementing Selection Sort
Now that we understand the concept, let's implement selection sort in Python.
def selection_sort(arr): for i in range(len(arr)): min_index = i for j in range(i + 1, len(arr)): if arr[j] < arr[min_index]: min_index = j arr[i], arr[min_index] = arr[min_index], arr[i] # Example usage: arr = [5, 3, 8, 1, 2] selection_sort(arr) print(arr) # Output: [1, 2, 3, 5, 8]
This implementation uses nested loops to traverse the array and find the minimum element. The outer loop keeps track of the sorted portion, while the inner loop finds the minimum element in the unsorted portion. Once the minimum element is found, we swap it with the current element in the outer loop.
Applications and Limitations
Selection sort is best suited for small arrays or when you need a simple, easy-to-understand algorithm. However, it does have some limitations:
- Efficiency: With a time complexity of O(n²), selection sort is inefficient for larger arrays compared to other sorting algorithms like merge sort or quick sort.
- Stability: Selection sort is not a stable sort, which means that equal elements might not retain their relative order after sorting. This can be important for some applications.
Despite these limitations, selection sort can be a good choice when simplicity is more important than performance or when working with smaller data sets.
In conclusion, selection sort is like the humble, easy-going friend among sorting algorithms. It might not be the fastest or the most sophisticated, but it's reliable and easy to understand, making it a great starting point for those new to sorting algorithms.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Smiley Face (psst, it's free!).
FAQ
What is the selection sort algorithm?
The selection sort algorithm is a simple comparison-based sorting technique that works by dividing the input list into two parts: the sorted part and the unsorted part. Initially, the sorted part is empty, and the unsorted part contains all the elements. The algorithm repeatedly selects the minimum (or maximum) element from the unsorted part and moves it to the end of the sorted part. This process continues until the entire list is sorted.
How does the selection sort algorithm work?
The selection sort algorithm works in the following steps:
- Find the minimum (or maximum) element in the unsorted part of the list and note its index.
- Swap the minimum (or maximum) element with the first element in the unsorted part.
- Move the boundary between the sorted and unsorted parts one element to the right.
- Repeat steps 1-3 until the entire list is sorted.
Can you provide a code example of selection sort in Python?
Sure! Here's a simple implementation of the selection sort algorithm in Python:
def selection_sort(arr): for i in range(len(arr)): min_index = i for j in range(i+1, len(arr)): if arr[j] < arr[min_index]: min_index = j arr[i], arr[min_index] = arr[min_index], arr[i] example_list = [64, 34, 25, 12, 22, 11, 90] selection_sort(example_list) print("Sorted array is:", example_list)
What is the time complexity of the selection sort algorithm?
The selection sort algorithm has a time complexity of O(n^2) for both average and worst-case scenarios, where n is the number of elements in the list. This is because the algorithm performs n-1 comparisons for the first element, n-2 for the second element, and so on until 1 comparison for the last element, resulting in a total of (n-1) + (n-2) + ... + 1 = n(n-1)/2 comparisons. This makes selection sort inefficient for large lists.
When should we use the selection sort algorithm?
Selection sort is best suited for small lists or lists that are already partially sorted because of its simplicity and quadratic time complexity. In cases where the data set is small or the computational resources are limited, selection sort can be a viable option. However, for larger lists or when efficiency is crucial, other more efficient sorting algorithms like quicksort or merge sort should be considered.