Quick Sort Explained
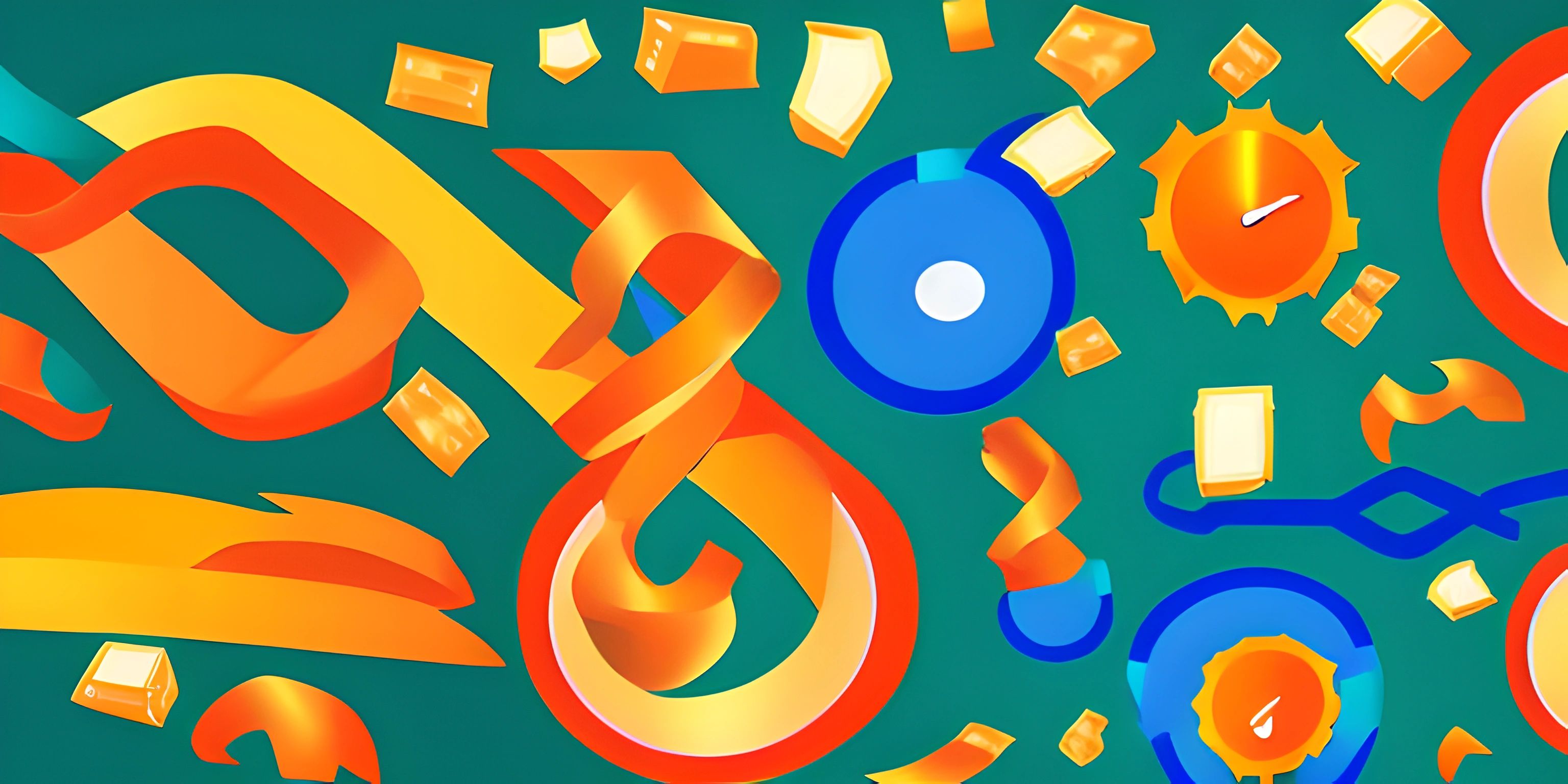
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting plays a crucial role in many programming tasks, and Quick Sort is one of the most popular algorithms for this job. It's known for its efficiency, simplicity, and is often the go-to choice when sorting is needed. So let's dive into the world of Quick Sort and see what makes it tick!
Quick Sort Basics
Quick Sort, also called partition-exchange sort, is a comparison-based sorting algorithm. It works by selecting an element called the pivot and partitioning the array around this pivot. The goal is to rearrange the elements so that all items less than the pivot come before it, and all items greater than the pivot come after it. This process is recursively applied to the sub-arrays on both sides of the pivot until the entire array is sorted.
Partitioning
The crux of the Quick Sort algorithm lies in the partitioning step. This is where the magic happens! Let's take a closer look at it.
- Choose a pivot: This can be done in various ways, such as picking the first, last, or middle element, or selecting a random element. The choice of pivot can impact the performance of the algorithm.
- Partition the array: Iterate through the array and compare each element against the pivot. If an element is less than the pivot, swap it with the first unpartitioned element, effectively moving it to the left side of the pivot.
- Update the pivot position: After the partitioning step, swap the pivot with the first element greater than it. This positions the pivot in its correct sorted position.
Here's a quick example using Python:
def partition(arr, low, high): pivot = arr[low] i = low + 1 j = high while True: while i <= j and arr[i] < pivot: i += 1 while i <= j and arr[j] > pivot: j -= 1 if i <= j: arr[i], arr[j] = arr[j], arr[i] else: break arr[low], arr[j] = arr[j], arr[low] return j def quick_sort(arr, low, high): if low < high: pivot_index = partition(arr, low, high) quick_sort(arr, low, pivot_index - 1) quick_sort(arr, pivot_index + 1, high) # Example usage arr = [3, 1, 4, 1, 5, 9, 2, 6, 5] quick_sort(arr, 0, len(arr) - 1) print(arr) # Output: [1, 1, 2, 3, 4, 5, 5, 6, 9]
Performance
Quick Sort's average-case time complexity is O(n log n), making it an efficient sorting algorithm. However, its worst-case time complexity is O(n^2), which can occur when the pivot selection is poor, leading to unbalanced partitions. Choosing a good pivot can help avoid this pitfall and ensure optimal performance.
Conclusion
Quick Sort is a versatile and powerful sorting algorithm, favored for its average-case performance and ease of implementation. With a good understanding of the partitioning process and careful pivot selection, you can harness the power of Quick Sort to sort your data efficiently and effectively.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).