Sorting Algorithms
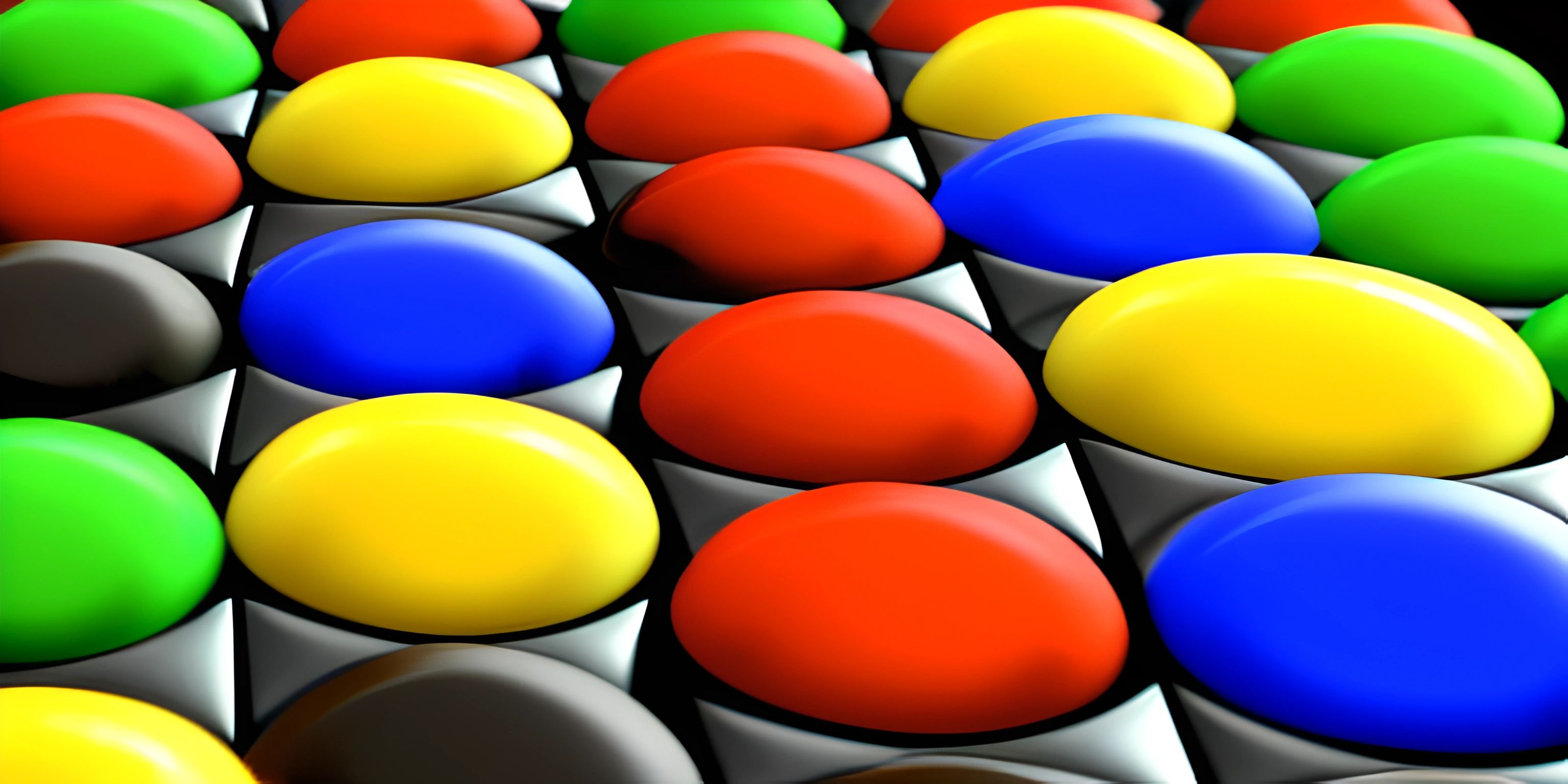
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting data is a fundamental task in programming, and there are a variety of algorithms available to help you do just that. In this article, we'll explore some popular sorting algorithms, comparing their efficiency, and discussing their applications.
Bubble Sort
Bubble sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The process is repeated until no swaps are needed. Here's an example of bubble sort in action:
def bubble_sort(arr): n = len(arr) for i in range(n): for j in range(0, n-i-1): if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j]
Bubble sort has a worst-case and average-case time complexity of O(n^2), making it inefficient for large datasets.
Selection Sort
Selection sort is another simple sorting algorithm that sorts an array by repeatedly finding the minimum element from the unsorted part and moving it to the beginning. Here's an example of selection sort:
def selection_sort(arr): n = len(arr) for i in range(n): min_index = i for j in range(i+1, n): if arr[j] < arr[min_index]: min_index = j arr[i], arr[min_index] = arr[min_index], arr[i]
Like bubble sort, selection sort also has a worst-case and average-case time complexity of O(n^2), making it inefficient for large datasets.
Quick Sort
Quick sort is a divide-and-conquer sorting algorithm that works by selecting a 'pivot' element from the array and partitioning the other elements into two groups, those less than the pivot and those greater than the pivot. The process is then applied recursively to the two groups. Here's an example of quick sort:
def partition(arr, low, high): pivot = arr[high] i = low - 1 for j in range(low, high): if arr[j] <= pivot: i += 1 arr[i], arr[j] = arr[j], arr[i] arr[i+1], arr[high] = arr[high], arr[i+1] return i + 1 def quick_sort(arr, low, high): if low < high: pivot_index = partition(arr, low, high) quick_sort(arr, low, pivot_index-1) quick_sort(arr, pivot_index+1, high)
Quick sort has an average-case time complexity of O(n * log(n)), making it more efficient than bubble sort and selection sort, especially for larger datasets.
Conclusion
Bubble sort, selection sort, and quick sort are just a few of the many sorting algorithms used in programming. Each algorithm has its own advantages and disadvantages, depending on factors such as the size and nature of the dataset. Understanding the principles and trade-offs of each algorithm will help you choose the best one for your specific use case. Additionally, exploring other algorithms like merge sort and heap sort can further expand your knowledge and problem-solving capabilities in programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).