Understanding Chaos Theory in Programming
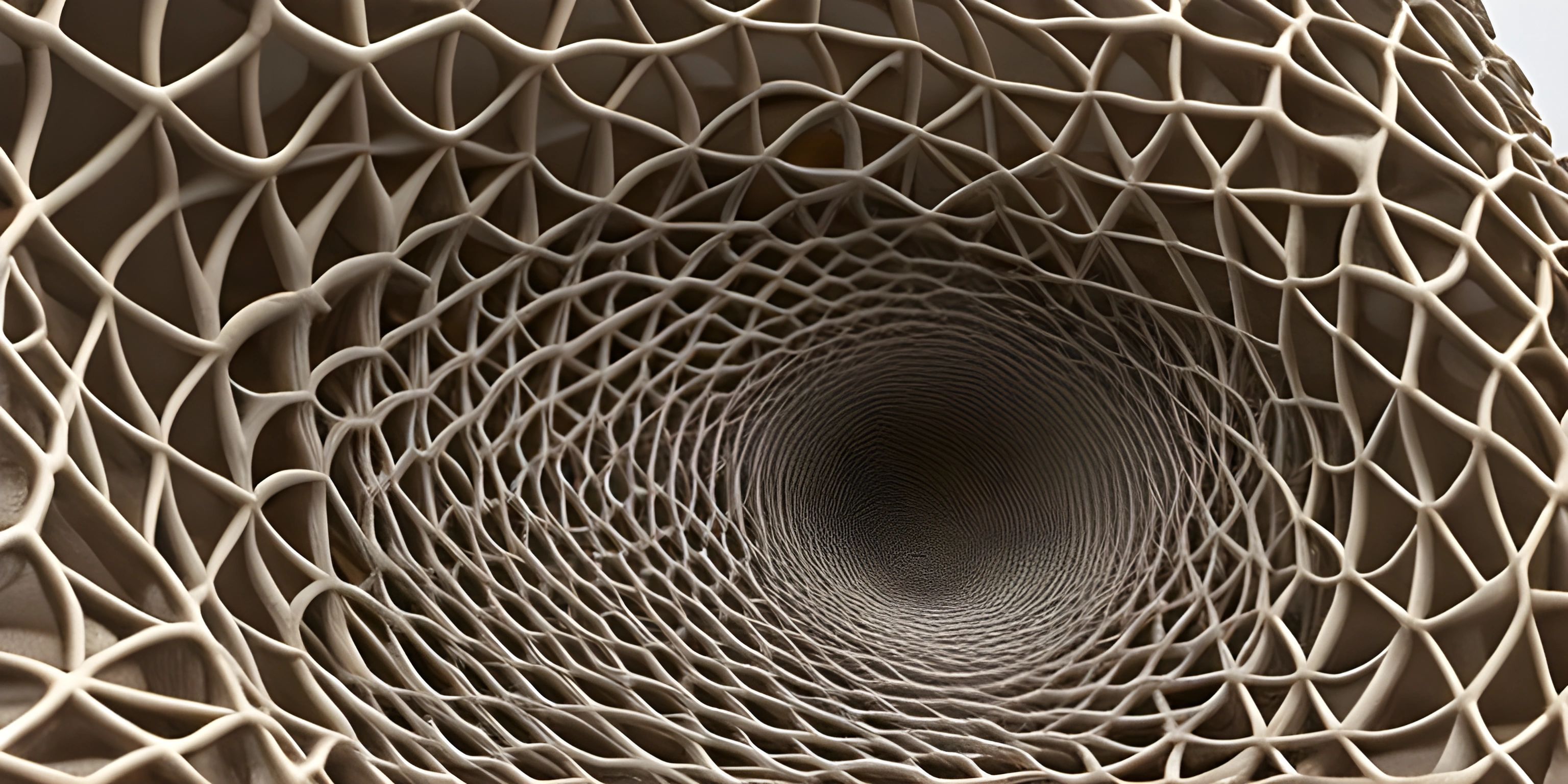
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Have you ever wondered how a butterfly flapping its wings in Brazil could potentially cause a tornado in Texas? Welcome to the wonderfully unpredictable world of chaos theory! This seemingly paradoxical idea is a fundamental concept in various fields, including programming and computer science. In this article, we'll explore chaos theory, its implications, and how it can impact your code like an unexpected plot twist in a thrilling mystery novel.
What is Chaos Theory?
Chaos theory is a branch of mathematics that deals with systems that appear to be disordered and random but are, in fact, following deterministic laws. In simpler terms, it's about finding the underlying order in seemingly chaotic systems. It's the science of surprises, the nonlinear and the unpredictable. Think of it as the Sherlock Holmes of mathematics, always finding the hidden connections that others overlook.
The Butterfly Effect
The butterfly effect is a key concept in chaos theory, describing how small changes in initial conditions can lead to vastly different outcomes. This phenomenon was popularized by meteorologist Edward Lorenz when he discovered that minute variations in his weather model's initial conditions resulted in drastically different weather patterns. It's like baking a cake where a pinch more of sugar could turn your dessert into an unrecognizable confection.
In programming, the butterfly effect can be seen in how tiny bugs or changes in code can lead to unexpected and sometimes catastrophic outcomes. A single misplaced semicolon or an off-by-one error can ripple through your program like a stone causing ripples in a pond.
Chaos Theory in Action
Random Number Generators
One practical application of chaos theory in programming is in random number generators (RNGs). While these generators are designed to produce sequences of numbers that lack any pattern, they're actually deterministic algorithms. This means that if you know the initial state (or seed), you can predict the entire sequence. However, small changes to the seed can result in vastly different sequences, a perfect example of the butterfly effect.
Here's a simple Python example to illustrate this:
import random # Set a seed random.seed(10) print(random.random()) # Output will always be the same for seed 10 # Change the seed slightly random.seed(11) print(random.random()) # Output will be different with seed 11
Fractals and Graphics
Fractals are another area where chaos theory shines. These complex geometric shapes exhibit self-similarity, meaning they look similar at any scale. Fractals are used in computer graphics to create realistic textures and landscapes. Think of them as the never-ending Russian dolls of the graphical world.
Here's an example of generating a simple fractal using Python:
import turtle def draw_fractal(length, depth): if depth == 0: turtle.forward(length) return length /= 3.0 draw_fractal(length, depth-1) turtle.left(60) draw_fractal(length, depth-1) turtle.right(120) draw_fractal(length, depth-1) turtle.left(60) draw_fractal(length, depth-1) # Initialize turtle turtle.speed(0) turtle.penup() turtle.goto(-200, 100) turtle.pendown() # Draw a fractal draw_fractal(400, 4) turtle.done()
This code will use the turtle
module to draw a Koch snowflake, a classic fractal pattern.
Chaos in Algorithms
Algorithms can also exhibit chaotic behavior. For example, some sorting algorithms may behave unpredictably with certain types of input data. The quicksort algorithm, for instance, can degrade to O(n^2) complexity if the pivot selection is poor, which is a case of chaotic behavior in deterministic systems.
Implications of Chaos Theory in Programming
Understanding chaos theory can help developers become more aware of the potential for small changes to have large impacts. This awareness is crucial for debugging, optimizing, and maintaining code. Here are a few implications:
- Sensitivity to Initial Conditions: Be mindful of how small changes in code or input data can lead to significant differences in program behavior.
- Testing and Debugging: Thorough testing is essential to catch and manage the butterfly effects in your code. Unit tests, integration tests, and stress tests can help identify and mitigate chaotic behavior.
- Optimization: When optimizing code, keep in mind that minor tweaks can have disproportionate effects on performance and stability. Use profiling tools to understand the impact of changes.
- Resilience and Robustness: Design systems to be resilient to small changes and errors. Implement fail-safes and redundancy to handle unexpected behavior gracefully.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What is chaos theory in simple terms?
Chaos theory is the study of systems that appear to be random and disordered but are actually following deterministic rules. It's about finding the hidden order in seemingly chaotic systems.
How does the butterfly effect relate to programming?
The butterfly effect illustrates how small changes in initial conditions can lead to vastly different outcomes. In programming, this means that tiny bugs or code changes can have significant and unpredictable impacts on your program's behavior.
Can you give an example of chaos theory in computer graphics?
Yes! Fractals are a great example. These complex geometric shapes show self-similarity and are used in computer graphics to create realistic textures and landscapes.
Why is understanding chaos theory important for programmers?
Understanding chaos theory helps programmers be more aware of how small changes can lead to large impacts, which is crucial for debugging, optimizing, and maintaining robust code.
How can chaos theory affect algorithms?
Some algorithms, like quicksort, can exhibit chaotic behavior with certain input data, leading to significantly different performance outcomes. Understanding these behaviors helps in designing more efficient and reliable algorithms.