C++ Compiler Errors Explained
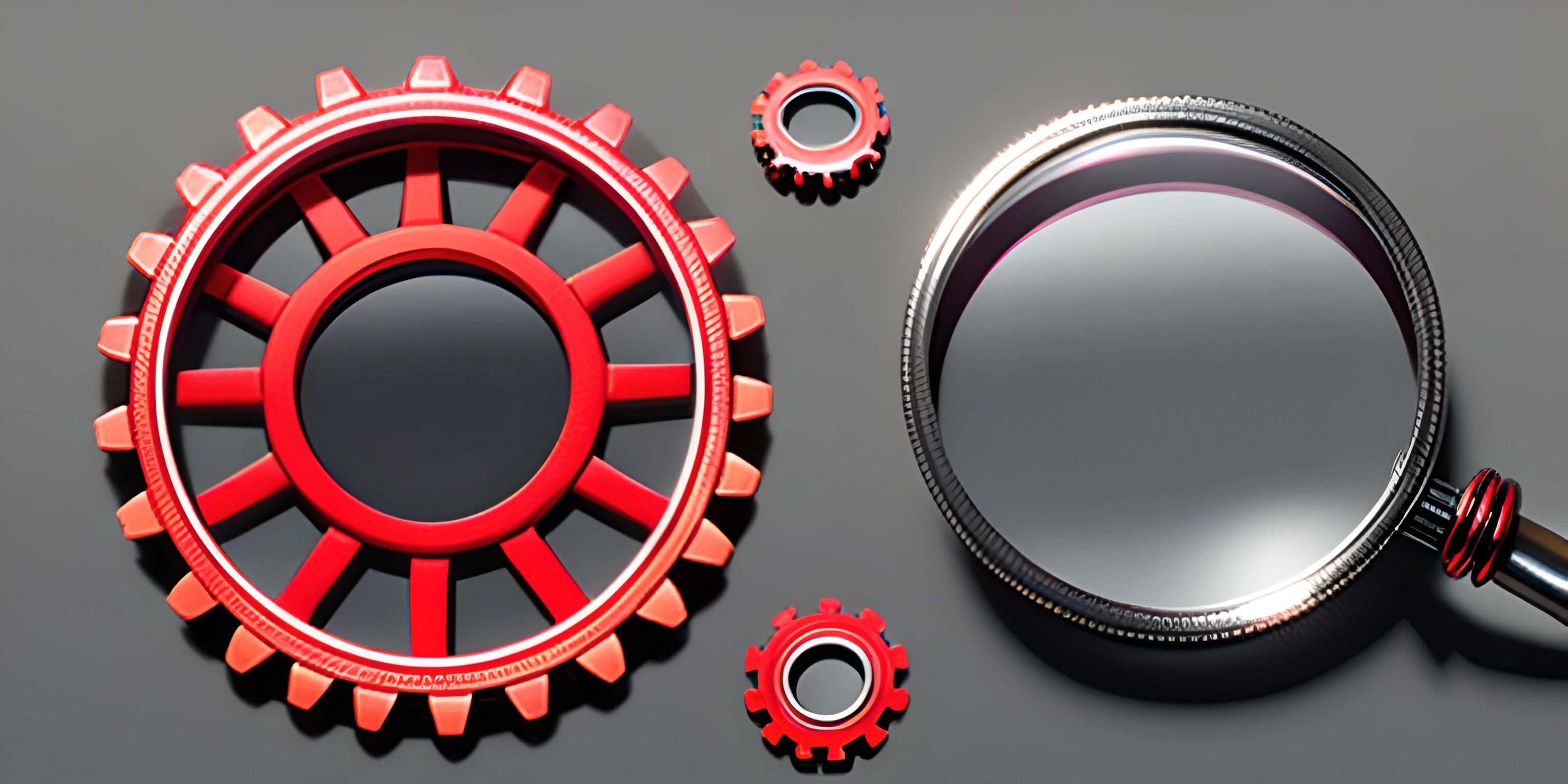
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When you're first learning C++, it's natural to come across compiler errors. These error messages can be confusing and frustrating, but don't worry – we'll break down some common errors, explain what they mean, and share some tips on how to fix them.
Syntax Errors
Syntax errors occur when the compiler encounters code that doesn't follow the rules of the C++ language. Some common syntax errors include:
Missing Semicolons
Each statement in C++ must end with a semicolon (;
). When you forget a semicolon, the compiler might get confused and report an error.
int main() { int x = 5 return 0; }
This code will produce an error because there's a missing semicolon after int x = 5
. To fix it, add the semicolon:
int main() { int x = 5; return 0; }
Mismatched Braces
Braces {}
are used in C++ to define the scope of functions, loops, and conditional statements. If you have an opening brace but forget the closing brace, you'll get an error.
int main() { if (true) { int x = 5; // Missing closing brace return 0; }
To fix this, make sure all opening braces have corresponding closing braces.
int main() { if (true) { int x = 5; } return 0; }
Semantic Errors
Semantic errors occur when your code is syntactically correct but doesn't make sense in the context of the C++ language. Examples include:
Undeclared Variables
If you attempt to use a variable that hasn't been declared, the compiler will report an error.
int main() { x = 5; return 0; }
This code will produce an error because x
hasn't been declared. To fix it, declare x
before using it:
int main() { int x = 5; return 0; }
Type Mismatch
When you try to assign a value of one type to a variable of a different type, you'll get a type mismatch error.
int main() { int x; x = "hello"; return 0; }
This code will produce an error because x
is an int
and we're trying to assign a const char*
to it. To fix it, make sure the variable type matches the value type:
int main() { const char* x; x = "hello"; return 0; }
Linker Errors
Linker errors occur when the compiler can't find the definitions of functions or variables referenced in your code. This often happens when you include a header file but forget to link the corresponding library.
For example, the following code uses the pow()
function from the <cmath>
library:
#include <iostream> #include <cmath> int main() { double x = pow(2, 3); std::cout << "2 cubed is: " << x << std::endl; return 0; }
If you're using g++
to compile, you may need to link the math library explicitly:
g++ -o my_program my_program.cpp -lm
The -lm
flag tells the linker to include the math library.
FAQ
What are some common syntax errors in C++?
Common syntax errors in C++ include missing semicolons, mismatched braces, and incorrect use of operators.
What are semantic errors in C++?
Semantic errors occur when your code is syntactically correct but doesn't make sense in the context of the C++ language. Examples include undeclared variables and type mismatches.
How can I resolve linker errors in C++?
To resolve linker errors, ensure that you've included the necessary header files and linked the corresponding libraries when compiling your code. For example, when using the pow()
function from the <cmath>
library, you may need to link the math library explicitly with the -lm
flag when compiling using g++
.