Understanding CPU Registers
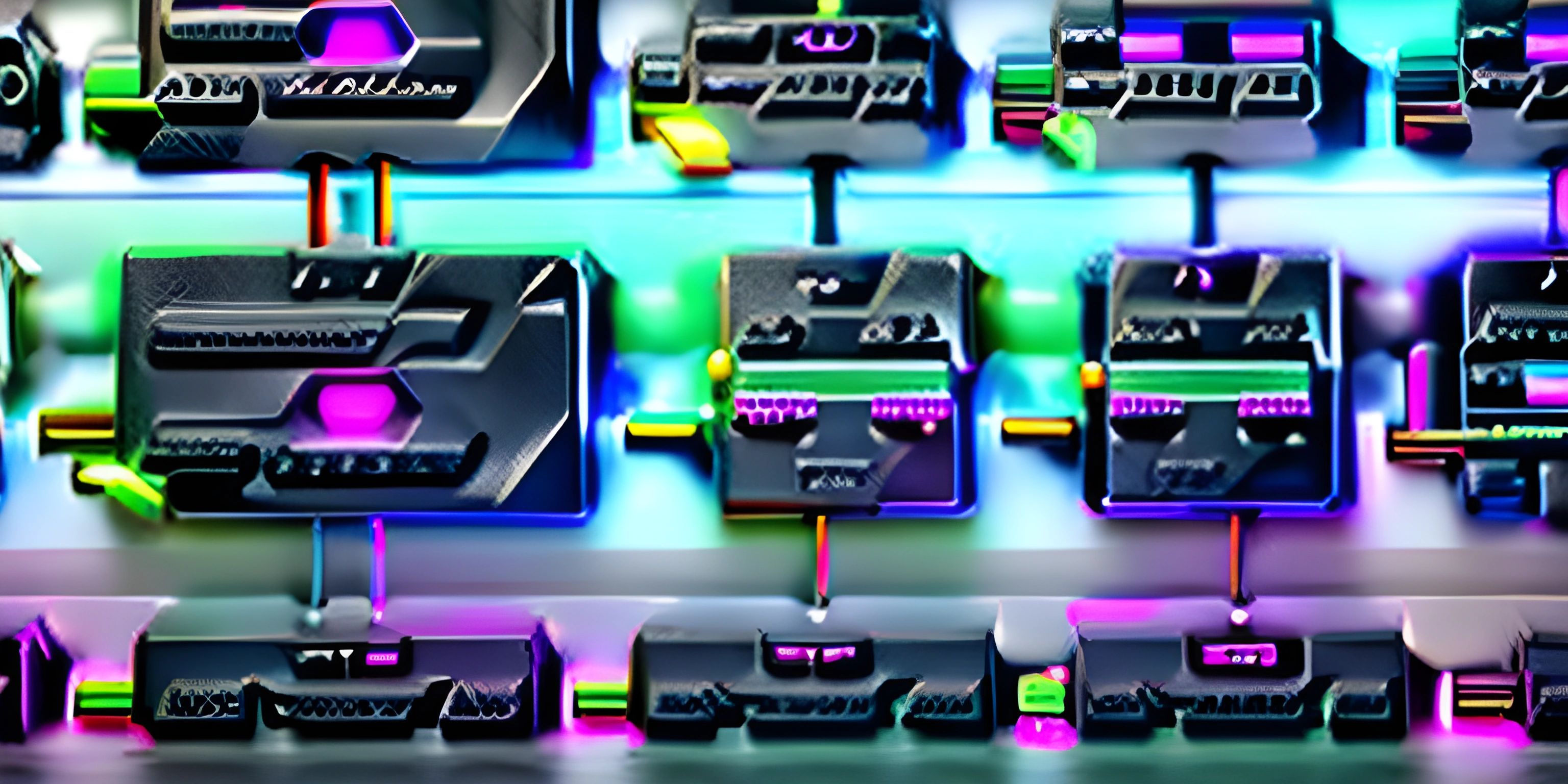
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine a chef in a busy kitchen. They have a vast pantry of ingredients (main memory) but only a limited number of hands and pockets (registers) to hold the essentials while cooking. Similarly, a CPU has a vast memory but relies on registers to hold data that it needs immediately. Let's dive into the world of CPU registers to see how they hold the kitchen of computing together.
What are CPU Registers?
Registers are small, fast storage locations within the CPU used to temporarily hold data and instructions. They are crucial for the CPU's efficiency because they provide quick access to frequently used values, much like the chef’s pockets in our kitchen analogy.
Here's a brief overview of a few common types of registers:
- General-Purpose Registers (GPRs): These are versatile registers used to store temporary data during execution of programs.
- Special-Purpose Registers: These registers have specific functions, such as the instruction register (IR), which holds the current instruction being executed.
- Status Registers: Also known as flag registers, these hold information about the state of the CPU, such as overflow, zero, and carry flags.
How Registers Enhance Performance
Just as a chef with more pockets can cook more efficiently, a CPU with more registers can perform tasks more swiftly. Let's explore how:
- Speed: Accessing registers is significantly faster than accessing main memory. Registers are usually located on the same chip as the CPU, reducing the time required to fetch or store data.
- Simplicity: Registers simplify the CPU's design. Operations performed on registers are straightforward and require fewer cycles compared to those involving memory.
- Reduced Bottlenecks: By storing intermediate results directly in registers, CPUs reduce the need to frequently access slower main memory, thereby reducing performance bottlenecks.
Types of CPU Registers
Let's take a closer look at some specific types of CPU registers and their roles:
1. General-Purpose Registers
These registers can be used for a variety of tasks, from arithmetic operations to temporary data storage during program execution. In x86 architecture, common GPRs include EAX, EBX, ECX, and EDX.
Here's an example in assembly language:
MOV EAX, 5 ; Move the value 5 into the EAX register MOV EBX, 10 ; Move the value 10 into the EBX register ADD EAX, EBX ; Add the value in EBX to EAX (EAX now contains 15)
2. Special-Purpose Registers
These are used for specific tasks essential to the CPU's operation. For example:
- Instruction Register (IR): Holds the current instruction being executed.
- Program Counter (PC): Keeps track of the next instruction to be executed.
3. Status Registers
Status registers hold condition flags that reflect the outcome of operations. Common flags include:
- Zero Flag (ZF): Set if the result of an operation is zero.
- Carry Flag (CF): Set if an arithmetic operation generates a carry out or borrow into the higher-order bit.
- Overflow Flag (OF): Set if an arithmetic operation results in an overflow.
Here's how status registers might be used in assembly:
CMP EAX, EBX ; Compare EAX and EBX JZ equal_label ; Jump to 'equal_label' if the Zero Flag is set ... equal_label: MOV ECX, 1 ; Execute this if EAX equals EBX
Register Allocation
Efficient register allocation is a critical aspect of compiler design. Compilers must decide which variables to keep in registers and which to spill to memory, balancing between the limited number of registers and the need for fast access.
Registers in Different Architectures
Different CPU architectures can have varying numbers and types of registers. For example:
- x86 Architecture: Has a relatively small number of registers (e.g., 16 GPRs in x86-64).
- ARM Architecture: Known for its large number of registers (e.g., 31 GPRs in ARMv8).
Understanding the architecture-specific register set is crucial for low-level programming and optimization.
Conclusion
CPU registers play an indispensable role in the efficient functioning of computer systems. They allow the CPU to quickly access and manipulate data, significantly boosting performance. Just like a well-organized kitchen helps a chef work efficiently, well-utilized registers enable a CPU to perform complex tasks swiftly and effectively.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are CPU registers used for?
CPU registers are used to hold data and instructions temporarily, allowing the CPU to access frequently used values quickly and efficiently.
Why are registers faster than memory?
Registers are located on the same chip as the CPU, reducing the time required to fetch or store data compared to accessing main memory.
What is a general-purpose register?
A general-purpose register can be used for various tasks, including arithmetic operations and temporary data storage during program execution.
How do status registers work?
Status registers hold condition flags that reflect the outcome of operations, such as whether the result of an operation is zero or if an arithmetic operation generated a carry.
Why is register allocation important in compilers?
Efficient register allocation is crucial in compilers to balance the limited number of registers and the need for fast data access, optimizing the performance of the generated code.