Assembly Registers and Memory
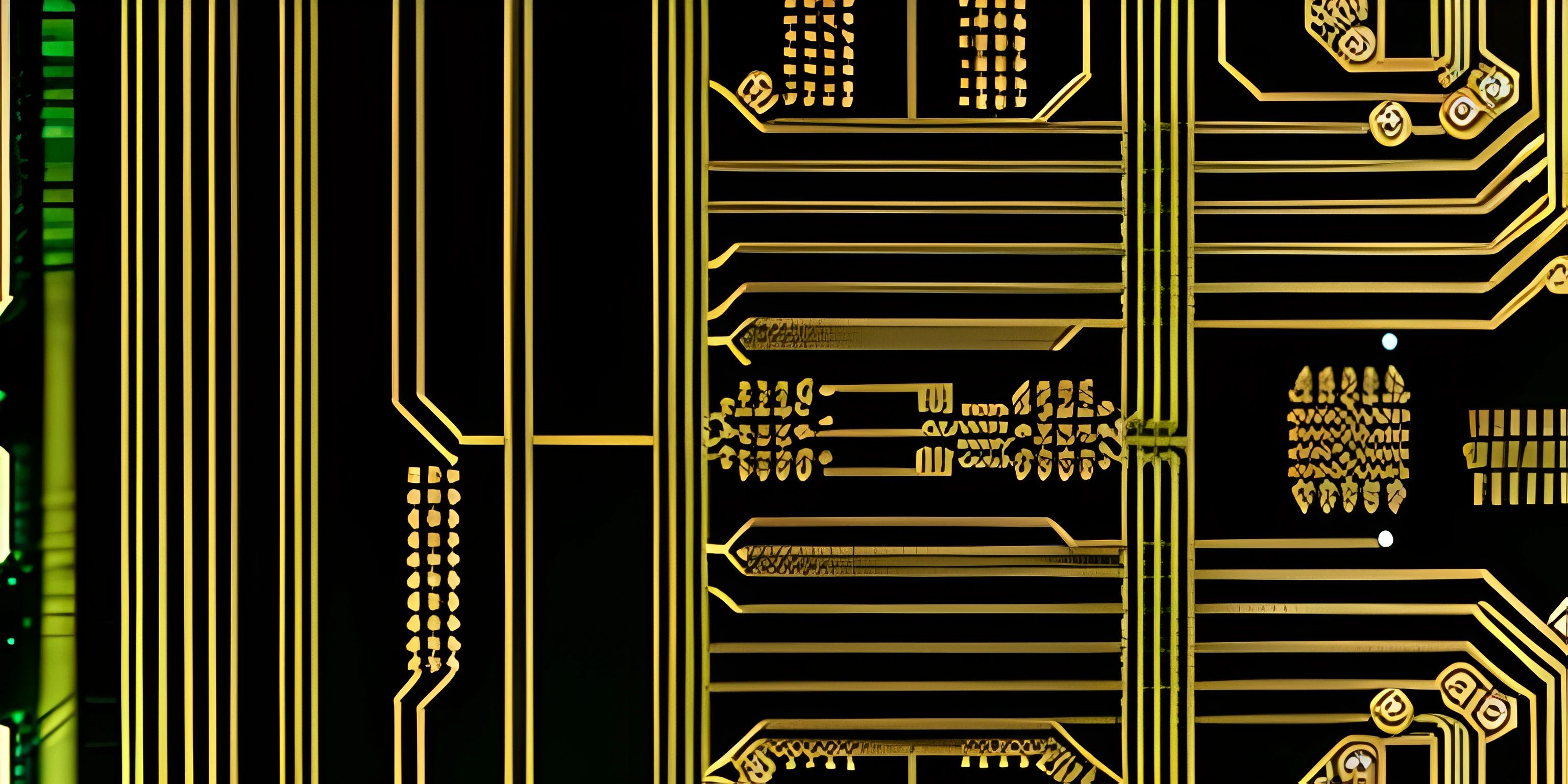
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When diving into the world of assembly language programming, you'll soon come across two crucial concepts: registers and memory. These two elements are the building blocks of data management and manipulation in low-level programming languages like assembly. Before we can execute complex operations, it's essential to know how these components work together.
Registers
Registers are small, fast storage locations within the CPU (Central Processing Unit) that hold data temporarily. They are like the CPU's personal notepad, allowing it to quickly jot down and access data as it processes instructions. Registers come in various sizes, such as 8-bit, 16-bit, 32-bit, and 64-bit, depending on the CPU architecture.
In assembly language programming, you'll often find yourself working with registers to store, move, and manipulate data. They play a vital role in arithmetic operations, data transfer, and decision-making.
Types of Registers
There are several types of registers, each with its purpose:
- General-purpose registers (GPRs): Stored data for arithmetic and logic operations.
- Stack pointer registers: Point to the top of the stack memory, used for managing the call stack.
- Instruction pointer registers: Keep track of the next instruction to be executed in the program.
- Segment registers: Store pointers to specific memory segments for memory management.
- Flag registers: Hold status bits that indicate the results of operations or the state of the CPU.
Memory
Memory is where the computer stores data and instructions outside of the CPU. The memory used in assembly language programming is usually RAM, which stands for Random Access Memory. Unlike registers, memory is a much larger storage space but slower to access.
Addressing Memory
When you want to work with data in memory, you need to know its location. This is done through memory addressing, where each memory location has a unique address, much like houses on a street. In assembly language, you'll often use pointers to store and manipulate these memory addresses.
Memory Segments
To better organize memory, it is divided into segments. Each segment has a specific purpose and size. Some common memory segments include:
- Code segment: Holds the program instructions.
- Data segment: Contains global and static variables.
- Stack segment: Manages the call stack.
- Heap segment: Stores dynamically allocated memory.
Interplay between Registers and Memory
Registers and memory work together to manage data in assembly language programming. In general, data is loaded from memory into registers for processing, and the results are then stored back into memory.
Here's a simple example in assembly language that demonstrates how registers and memory interact:
mov eax, [myVar] ; Load the value of myVar from memory into the EAX register add eax, 5 ; Add 5 to the value in the EAX register mov [myVar], eax ; Store the updated value back into memory at the location of myVar
In this example, the data stored at the memory address of myVar
is loaded into the EAX
register. The value in EAX
is then incremented by 5. Finally, the updated value is stored back in memory at the location of myVar
.
By understanding how registers and memory work in assembly language programming, you'll unlock the power to manipulate data at a low level, giving you greater control and efficiency in your programs.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are registers in assembly language programming?
Registers are small, fast storage spaces within a computer's CPU that hold data and instructions for quick access during assembly language programming. They play an essential role in managing data by storing and manipulating values, holding memory addresses, and assisting with arithmetic and logical operations.
How is memory used in assembly language programming?
Memory in assembly language programming is used to store data and instructions that cannot fit in the CPU's limited register space. It is organized into a large array of bytes or words, each with a unique address. Assembly programmers can read from and write to memory addresses using specific instructions, such as loading a value from memory into a register or storing a value from a register into memory.
What is the difference between registers and memory in assembly language?
The main difference between registers and memory in assembly language is their location and speed. Registers are located within the CPU and are much faster than memory, as they can be accessed directly during program execution. Memory, on the other hand, is a separate component from the CPU and requires more time to access. Additionally, registers have limited storage capacity, while memory can store much larger amounts of data.
How are registers named in assembly language?
Registers are typically named according to their purpose or function in assembly language. General-purpose registers can be used for various tasks, such as holding values or memory addresses, and are usually named with a letter followed by a number (e.g., R0, R1, R2). Some registers have specific functions, like the program counter (PC) or the stack pointer (SP), and are named accordingly.
Can you provide an example of using registers and memory in assembly language?
Certainly! Here's a simple example using ARM assembly language, where we add two numbers stored in memory and save the result in a register.
LDR R0, [R1] ; Load the value at memory address in R1 into R0 LDR R2, [R3] ; Load the value at memory address in R3 into R2 ADD R4, R0, R2 ; Add the values in R0 and R2, and store the result in R4
In this example, R1 and R3 hold memory addresses, and R0 and R2 are used to load values from memory. R4 holds the result of the addition operation.