Debugging Tips and Tools
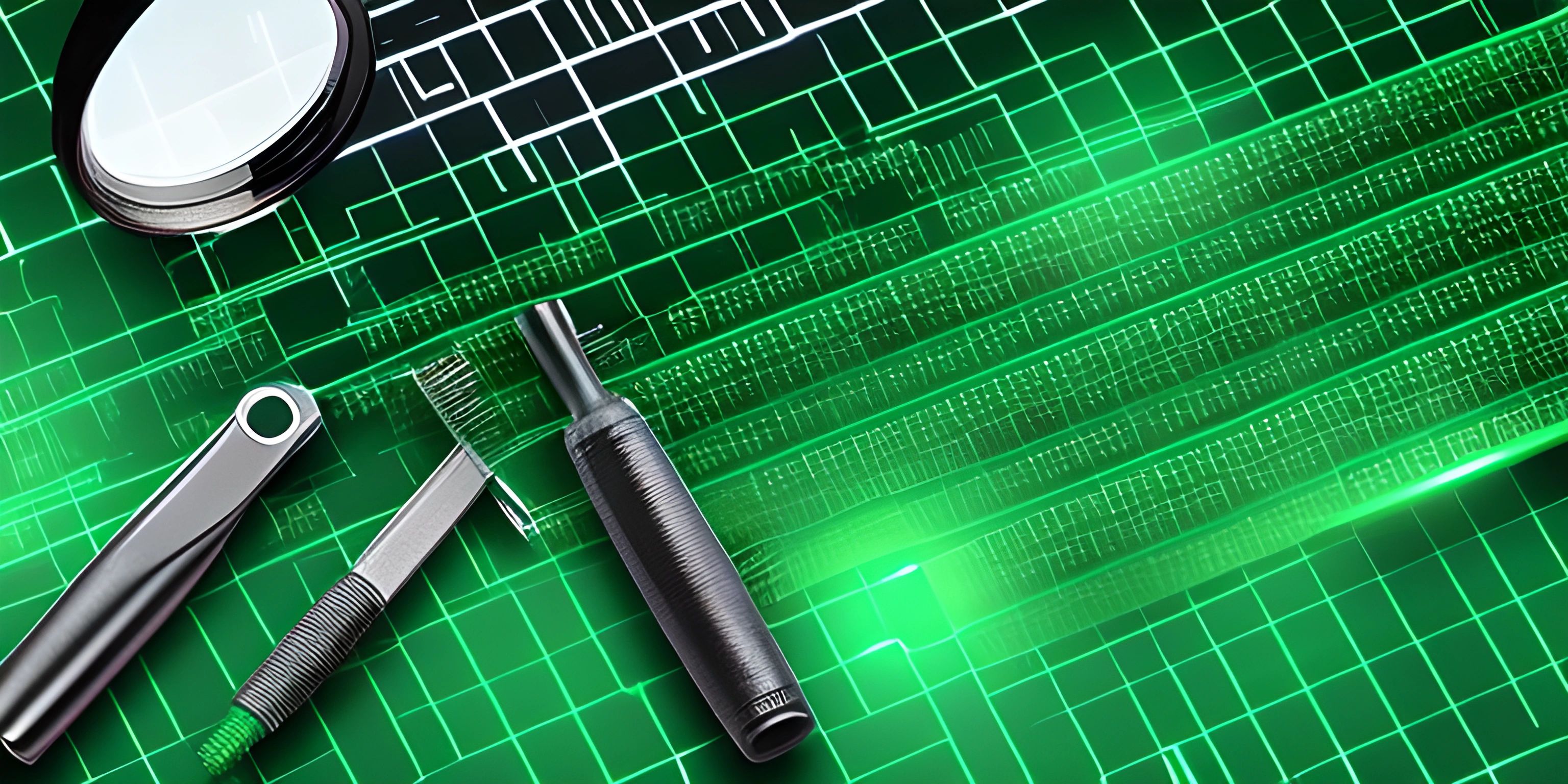
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Debugging is an essential skill for any programmer. It involves finding and fixing errors in your code, also known as bugs. In this article, we will discuss some tips and tools to help you debug your code efficiently.
1. Understand the Problem
Before diving into the code, make sure you understand the problem you are trying to solve. Read the error message carefully and try to identify the root cause of the issue. If you are not familiar with the error message, search for it online to get more information.
2. Use a Debugger
A debugger is a tool that allows you to execute your code step-by-step, inspect variables, and set breakpoints. Breakpoints are points in the code where the debugger will pause execution so you can examine the state of the program.
In-browser Debugger (for JavaScript)
Most modern web browsers come with built-in developer tools, including a debugger for JavaScript. To access the debugger, right-click on the webpage and select "Inspect" or "Inspect Element". Then, navigate to the "Sources" or "Debugger" tab.
Node.js Debugger
For debugging Node.js applications, you can use the built-in debugger by running the command node inspect your-script.js
. This will start a debugging session in your terminal. You can also use IDEs like Visual Studio Code, which have integrated debuggers for Node.js.
3. Add Debugging Statements
Adding debugging statements, such as console.log()
(in JavaScript) or print()
(in Python), can help you track the flow of your code and the value of variables at different points in time. Make sure to remove these statements once you've fixed the issue.
4. Break Down the Problem
If you are having trouble understanding a complex piece of code, break it down into smaller, more manageable pieces. This will make it easier to identify the source of the problem and debug it.
5. Rubber Duck Debugging
Rubber duck debugging is a technique where you explain your code line-by-line to a rubber duck or any inanimate object. This forces you to think critically about your code and often helps you find the issue.
6. Use Version Control
Using version control systems like Git can help you track changes in your code and revert to a previous working state if you introduce a bug.
7. Ask for Help
If you are still struggling to find and fix the issue, don't hesitate to ask for help from your peers, mentors, or online communities like Stack Overflow.
Remember, debugging is an essential part of programming, and with practice, you will become more efficient at finding and fixing bugs in your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What are some common debugging techniques?
There are several common debugging techniques that can be used to find and fix errors in your code:
- Rubber Duck Debugging: This involves explaining your code line by line to a rubber duck (or any other inanimate object). It helps you to think more deeply about your code and often leads to the discovery of errors.
- Print Debugging: Inserting print statements in your code to display the values of variables at specific points, which can help you track the flow of data and identify issues.
- Code Review: Having a fellow programmer review your code can help find issues you might have missed.
- Breakpoints: Using a debugger tool, you can set breakpoints in your code to pause execution at specific points and examine the state of your program.
What tools can I use to help me with debugging?
There are various debugging tools available depending on the programming language you're using. Some popular ones include:
- Chrome DevTools: For debugging JavaScript, HTML, and CSS in web development.
- Visual Studio Debugger: Supports debugging in various languages, including C++, C#, and Python, when using the Visual Studio IDE.
- GDB (GNU Debugger): A powerful command-line debugger for C, C++, and other languages.
- PDB (Python Debugger): A built-in debugger for Python scripts.
How can I set breakpoints in my code using a debugger?
Setting breakpoints in your code using a debugger depends on the tool you're using. Here are examples for popular debuggers:
- Chrome DevTools: Open the DevTools in your browser, navigate to the "Sources" tab, find the relevant JavaScript file, and click on the line number where you want to set a breakpoint.
- Visual Studio Debugger: Open your code in Visual Studio, click in the margin to the left of the line number where you want to set a breakpoint, or press F9.
- GDB: In the command line, use the "break" command followed by the file name and line number, like this:
break file.c:10
. - PDB: Add the following line in your Python script where you want the breakpoint:
import pdb; pdb.set_trace()
.