Python Debugging Tips
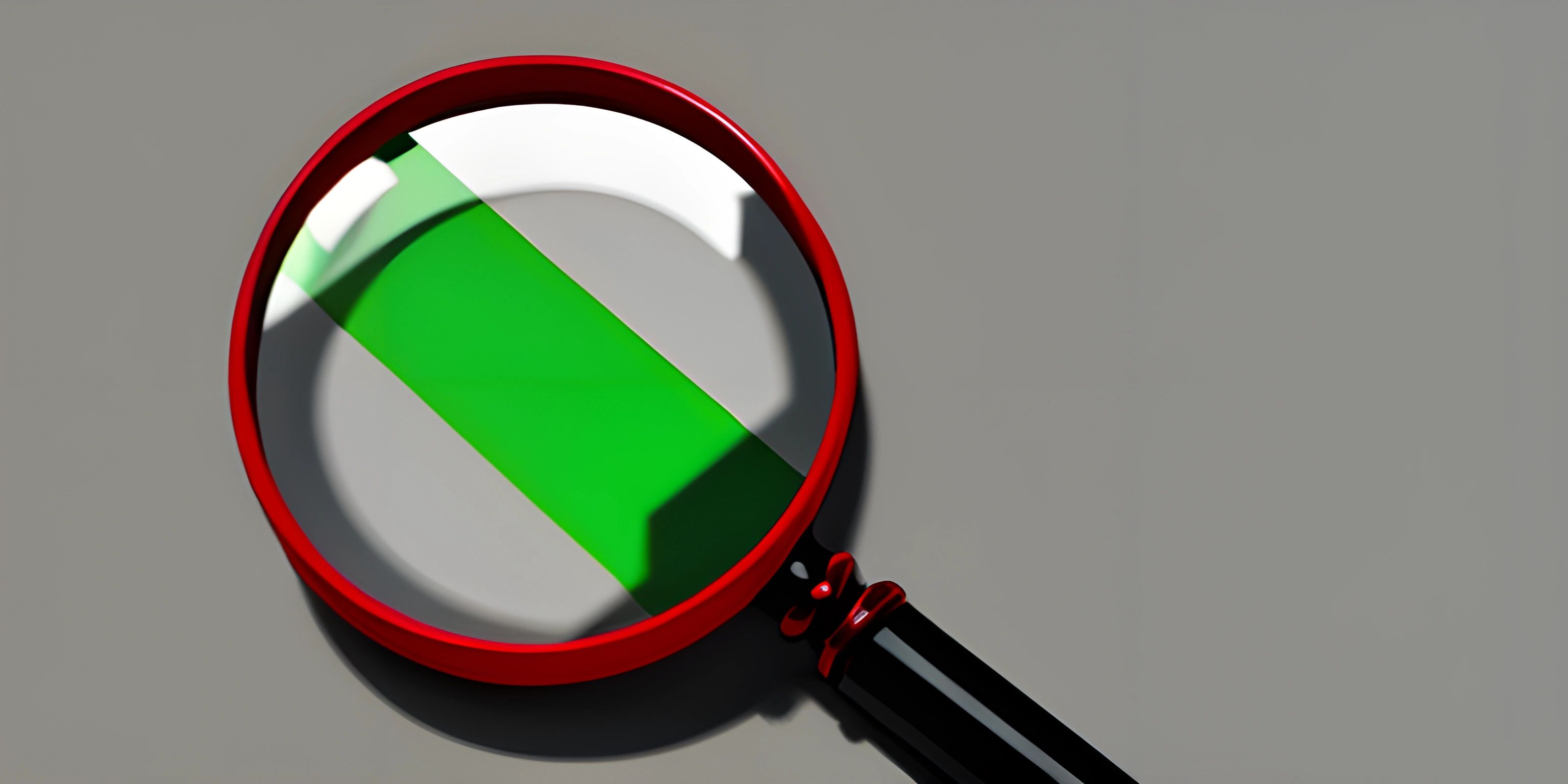
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Debugging is an essential skill for any programmer. When your Python code runs into an issue, knowing how to identify and fix the problem is crucial. This article will cover some tips and tricks to help you debug your Python code more effectively.
Understand the Error Message
Python error messages can be intimidating at first, but they provide valuable information about what went wrong in your code. When you encounter an error, read the message carefully and try to understand what it is telling you. Here's an example of a common error message:
Traceback (most recent call last): File "example.py", line 3, in <module> result = 5 / 0 ZeroDivisionError: division by zero
In this case, the error message indicates that there's a ZeroDivisionError
on line 3 of the example.py
file. The message even tells us the specific issue: "division by zero." Understanding the error message is the first step in resolving the problem.
Use Print Statements
Sometimes, the best way to debug your code is to add print statements throughout your code to check the values of variables and the flow of execution. This can help you identify where things go awry. For example, if you're trying to find out why a variable doesn't have the expected value, you can add a print statement before and after the line where the variable is modified:
print("Before:", my_variable) my_variable = my_variable * 2 print("After:", my_variable)
By reviewing the output, you can get a better sense of what is happening in your code.
Debugging with pdb
The Python Debugger (pdb
) is a powerful tool for debugging your code. You can set breakpoints in your code, step through the execution, and examine the values of variables at any point. To use pdb
, add the following line to your code where you want to set a breakpoint:
import pdb; pdb.set_trace()
When your code reaches this line, it will pause and enter the interactive debugger, allowing you to inspect the current state of your program, step through the code line by line, and even execute arbitrary Python code. This can be extremely helpful in identifying issues in your code.
Use an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) can help streamline the debugging process. Most IDEs offer built-in debugging tools, such as breakpoints, variable watches, and step-through execution. Popular Python IDEs like PyCharm and Visual Studio Code come with powerful debugging features that can save you time and effort during the debugging process.
Divide and Conquer
If you're unsure where the issue lies in your code, try breaking it down into smaller, more manageable parts. By isolating specific sections of your code and testing them independently, you can more easily pinpoint the source of the problem. This technique, known as "divide and conquer" debugging, can be an effective way to narrow down the scope of the issue and make it easier to resolve.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is the importance of understanding error messages in Python?
Understanding error messages is crucial because they provide valuable information about what went wrong in your code. By carefully reading and interpreting error messages, you can identify the cause of the issue and begin the process of resolving it.
What is the Python Debugger (`pdb`) and how is it useful for debugging?
The Python Debugger (pdb
) is a powerful tool for debugging your code. It allows you to set breakpoints, step through the execution of your code, and examine the values of variables at any point. This can help you identify issues in your code and understand the flow of execution more effectively.
How can an Integrated Development Environment (IDE) help with debugging?
An Integrated Development Environment (IDE) can streamline the debugging process by offering built-in debugging tools, such as breakpoints, variable watches, and step-through execution. These features can save you time and effort during the debugging process and make it easier to identify and resolve issues in your code.
What is the "divide and conquer" debugging technique?
The "divide and conquer" debugging technique involves breaking your code down into smaller, more manageable parts to isolate specific sections and test them independently. By doing this, you can more easily pinpoint the source of the problem and make it easier to resolve.