10 Ideas for Beginner JavaScript Projects
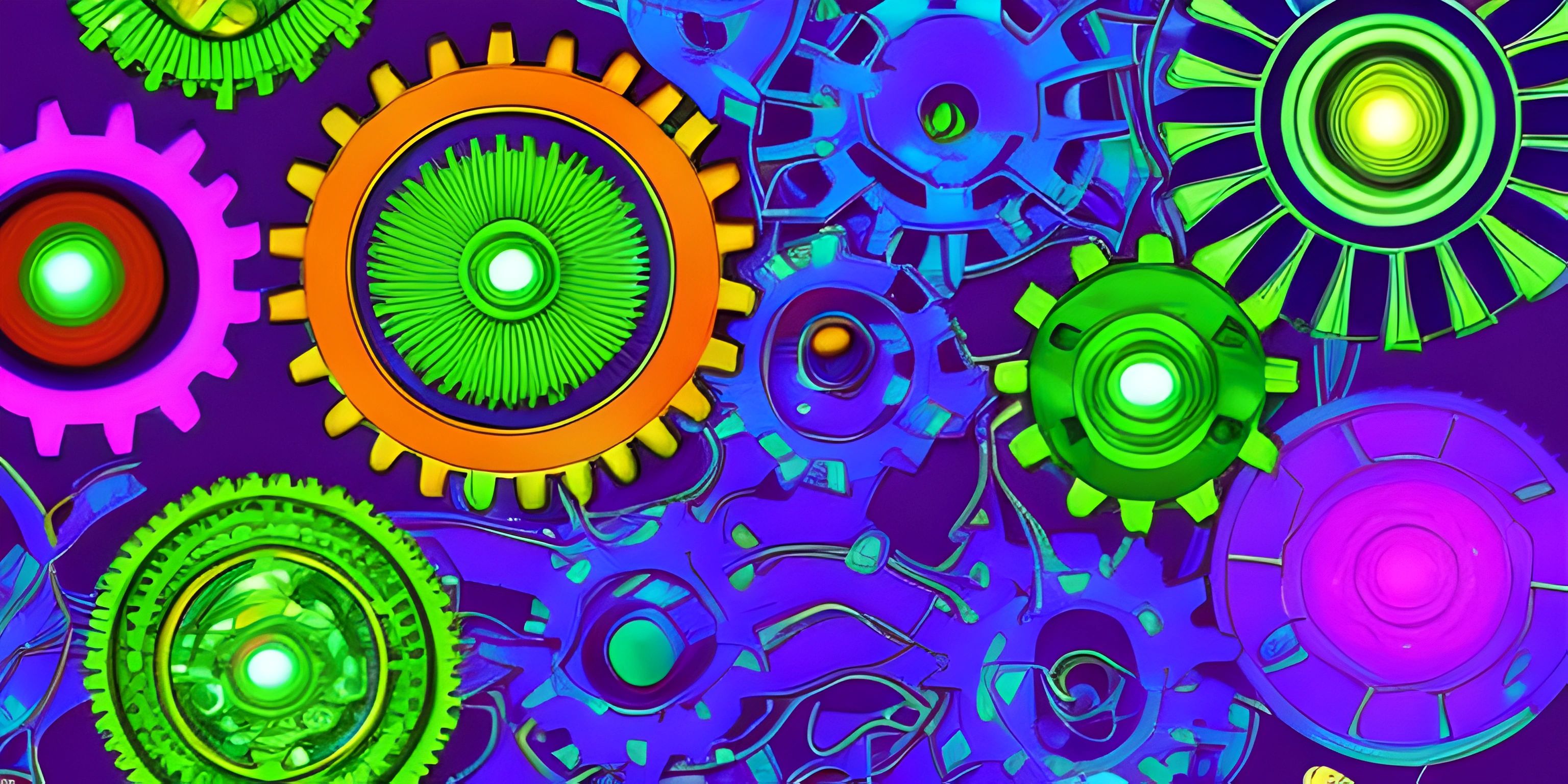
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
So you've learned the basics of JavaScript, and now you're itching to put your newfound skills to the test? Excellent choice, young padawan! It's time to embark on some programming adventures with these 10 brilliant beginner JavaScript project ideas. Fear not, for we've also included hints and tips to guide you on your quest.
1. Digital Clock
Create a digital clock that displays the current time on the screen. The clock should update every second.
Hints & Tips:
- Use the
Date
object to get the current time. - Create a function to update the time displayed on the screen.
- Use
setInterval()
to call the update function every second.
2. Temperature Converter
Build a simple temperature converter that allows users to switch between Celsius and Fahrenheit.
Hints & Tips:
- Create a function that takes a temperature value and converts it to the other unit.
- Use event listeners to detect when the user wants to convert the temperature.
3. Tip Calculator
Develop a tip calculator that takes the bill amount, tip percentage, and number of people, then calculates the tip per person.
Hints & Tips:
- Use input fields to collect user data.
- Create a function to calculate the tip per person.
- Display the result on the screen.
4. Simple To-Do List
Design a to-do list app where users can add, remove, and mark tasks as completed.
Hints & Tips:
- Use an array to store the to-do items.
- Create functions to add, remove, and toggle the completion status of tasks.
- Use
localStorage
to save and load the to-do list.
5. Random Quote Generator
Create a random quote generator that displays a new quote when the user clicks a button.
Hints & Tips:
- Store an array of quotes.
- Create a function to randomly select a quote from the array.
- Use event listeners to detect when the user clicks the button.
6. Color Flipper
Develop a color flipper that changes the background color of the page when the user clicks a button.
Hints & Tips:
- Use an array of colors.
- Create a function to randomly select a color from the array and apply it as the background color.
- Listen for button clicks to trigger the color change.
7. Countdown Timer
Build a countdown timer that allows users to set a time and then counts down to zero.
Hints & Tips:
- Use input fields for users to set the countdown time.
- Create a function to update the time displayed and decrease the remaining time.
- Use
setInterval()
to call the update function every second.
8. Simple Calculator
Design a simple calculator that performs basic arithmetic operations like addition, subtraction, multiplication, and division.
Hints & Tips:
- Create functions for each of the basic arithmetic operations.
- Use input fields to collect the numbers to be operated on.
- Use buttons and event listeners to trigger the corresponding functions.
9. Text-Based Adventure Game
Develop a text-based adventure game where the user makes choices to progress through the story.
Hints & Tips:
- Write a simple storyline with multiple paths and endings.
- Use
prompt()
andalert()
to interact with the user. - Create functions to handle different story paths based on user choices.
10. Quiz App
Create a quiz app with multiple-choice questions that tracks the user's score.
Hints & Tips:
- Store an array of question objects, each containing a question, possible answers, and the correct answer.
- Create functions to display the questions, handle user input, and update the score.
- Use event listeners to detect when the user submits their answer.
Now, take a deep breath and dive into these projects. Remember, practice makes perfect! Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are some beginner-friendly JavaScript project ideas?
Here are 10 beginner-friendly JavaScript project ideas:
- To-do List App
- Calculator
- Stopwatch / Timer
- Simple Quiz Game
- Weather App
- Random Quote Generator
- Image Carousel
- Tic-Tac-Toe Game
- Password Generator
- Currency Converter
How can I approach building a Calculator project in JavaScript?
To build a calculator in JavaScript, follow these steps:
- Design a simple UI with an input field and buttons for numbers and operations.
- Add event listeners to the buttons.
- Perform the math operations using JavaScript functions.
- Output the result in the input field.
- Add error handling to ensure valid inputs.
What should I consider when creating a Weather App in JavaScript?
When creating a Weather App, consider the following:
- Choose a reliable weather API to fetch data from.
- Create a functional UI to display the weather information.
- Handle user input (e.g., city, zip code) to fetch location-specific data.
- Use JavaScript to parse and display the weather data.
- Ensure error handling for invalid locations.
Can you provide tips for creating a Tic-Tac-Toe game in JavaScript?
Here are some tips for creating a Tic-Tac-Toe game:
- Design a 3x3 grid for the game board.
- Implement a function to handle player moves and update the board.
- Create a function to check for winning conditions (rows, columns, diagonals).
- Add a function to handle a draw scenario.
- Implement game reset and score tracking features.
How can I create a Random Quote Generator in JavaScript?
To create a Random Quote Generator, follow these steps:
- Collect a list of quotes to display.
- Design a simple UI to display the quote and an action button.
- Create a function to generate a random index within the quote list.
- Use JavaScript to update the UI with the selected quote.
- Add an event listener to the action button to trigger the random quote function.