Assembly Language
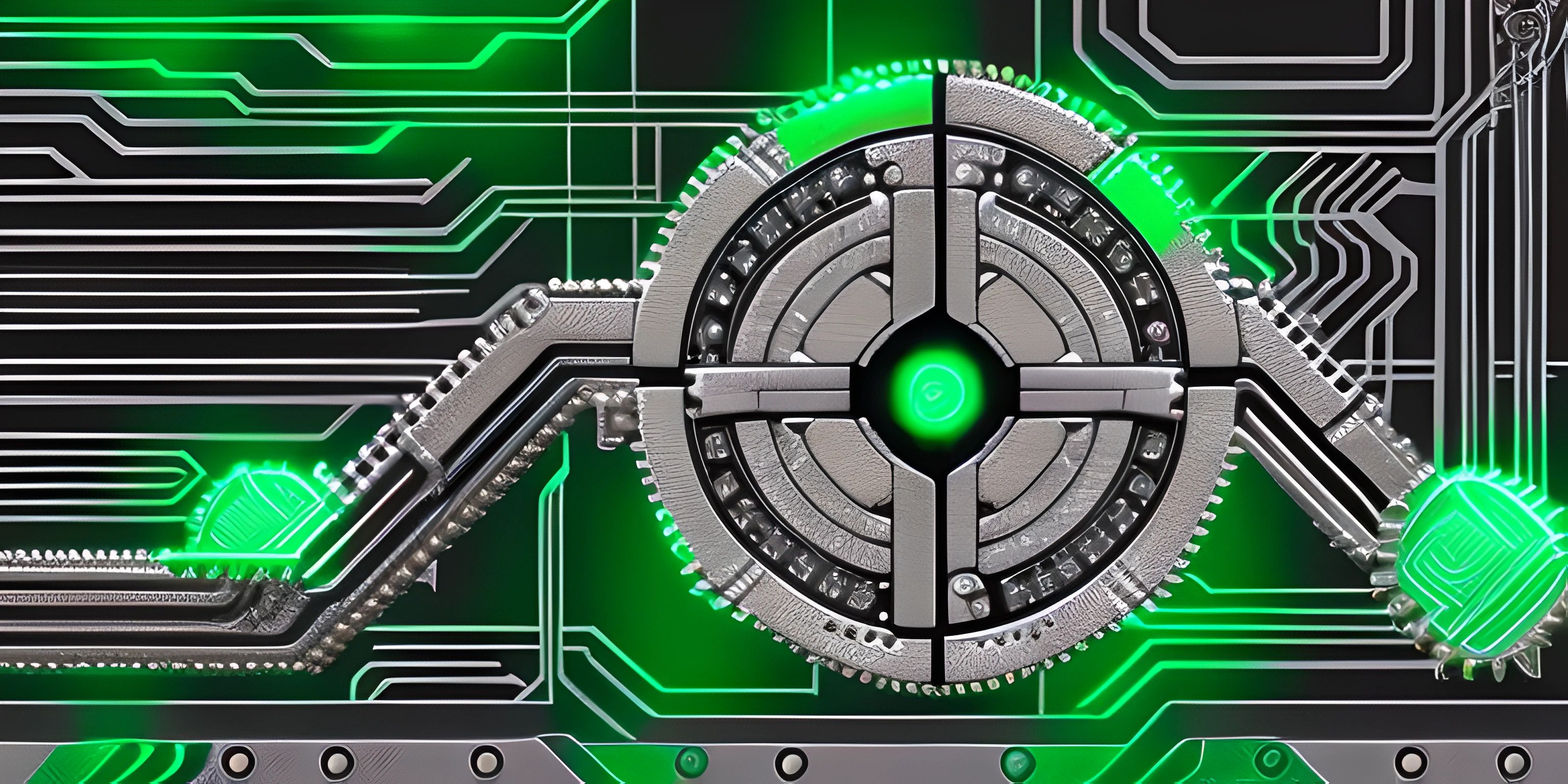
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Assembly language is a fascinating creature in the programming world, lurking in the dark depths of computer systems. It's a low-level programming language that is designed to be easily translated into machine code (the 1s and 0s that computers really understand). Since it's closer to the computer hardware, assembly language is often used when performance is critical or for tasks that require direct control over the hardware.
Architecture-Specific Nature
Unlike high-level programming languages like Python or Java, assembly language is architecture-specific. This means that an assembly language program written for one CPU architecture (e.g., x86) would not work on another architecture (e.g., ARM) without being rewritten or translated. This is because each CPU architecture has its own unique set of instructions, and assembly language is simply a human-readable representation of those instructions.
Instruction Set
Each CPU architecture has a set of instructions that can be executed. These are called the instruction set. In assembly language, each instruction typically corresponds to a single line of code. Some common instructions include:
- Loading data from memory
- Storing data to memory
- Performing arithmetic operations
- Comparing values
- Jumping to another location in the code
Here's a very simple example of assembly language code, in this case for an x86 CPU:
mov eax, 1 add eax, 2
This code moves the value 1
into the eax
register, then adds 2
to the value stored in eax
. After execution, eax
would hold the value 3
.
Assemblers and Disassemblers
To create an executable program, assembly language code needs to be translated into machine code. This is done using a tool called an assembler. An assembler takes the assembly language source code and generates a binary file containing the machine code instructions that can be executed by the CPU.
On the flip side, a disassembler is a tool that can take a binary file and generate the corresponding assembly language code. Disassemblers are often used for reverse engineering or debugging purposes.
Use Cases for Assembly Language
While high-level languages are generally more popular due to their ease of use and portability, assembly language still has its place in the programmer's toolbox. Some scenarios where assembly language may be useful include:
- Performance optimization: When every cycle counts, assembly language can be used to write highly optimized code that takes full advantage of the CPU's capabilities.
- Low-level hardware control: In some cases, direct control over hardware components (like memory or peripheral devices) is required. Assembly language provides the necessary level of control for these tasks.
- Bootloaders and firmware: Many low-level system components, like bootloaders and firmware, are written in assembly language due to their need for direct hardware access and minimal resource usage.
- Reverse engineering and debugging: Understanding assembly language can be invaluable when debugging or reverse engineering software at the machine code level.
As you can see, assembly language still has a role to play in the world of programming. While it may not be the go-to language for everyday tasks, knowing assembly language and how it works can give you a deeper understanding of computer systems and unlock new possibilities in your programming journey.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is assembly language and why is it important?
Assembly language is a low-level programming language that is used to communicate directly with a computer's hardware. It is important because it allows programmers to write more efficient and faster programs, as well as to interact with the hardware at a more granular level. This can be particularly useful for tasks such as developing operating systems, device drivers, and other system-level software.
How does assembly language differ from high-level programming languages?
Assembly language is quite different from high-level programming languages like Python or Java. High-level languages are designed to be more human-readable and abstract away many hardware-specific details, whereas assembly language is closer to machine code, making it more challenging to read and write. Programs written in assembly language are typically more efficient and faster because they are designed to work directly with the computer's hardware. However, this also means that assembly language programs are usually harder to maintain and port to different hardware platforms.
What is the role of an assembler in assembly language programming?
An assembler is a program that translates assembly language code into machine code, which is the binary representation of instructions that a computer's hardware can execute. The assembler takes the mnemonics, or human-readable representations of operations and operands, from the assembly language code and converts them into their corresponding machine code instructions.
Can you provide an example of a simple assembly language program?
Sure! Here's a very basic example of an assembly language program that adds two numbers together and stores the result in a register. This example uses Intel x86 assembly language:
mov eax, 5 ; Move the value 5 into the EAX register mov ebx, 7 ; Move the value 7 into the EBX register add eax, ebx ; Add the values in EAX and EBX, store the result in EAX
In this example, the mov
instruction is used to move values into registers, and the add
instruction is used to perform addition. The result of the addition (12) is stored in the EAX register.
Is learning assembly language still relevant today?
While most modern software development uses high-level programming languages, learning assembly language can still be beneficial for several reasons. It helps build a deeper understanding of how computer hardware works, which can be valuable for troubleshooting, optimization, and debugging. Assembly language programming is also relevant for specific tasks such as writing operating systems, device drivers, firmware, and other low-level code. Additionally, knowing assembly language can be useful for reverse-engineering and analyzing malware or other security-related tasks.