Discord.js Embeds: Craft Rich Messages
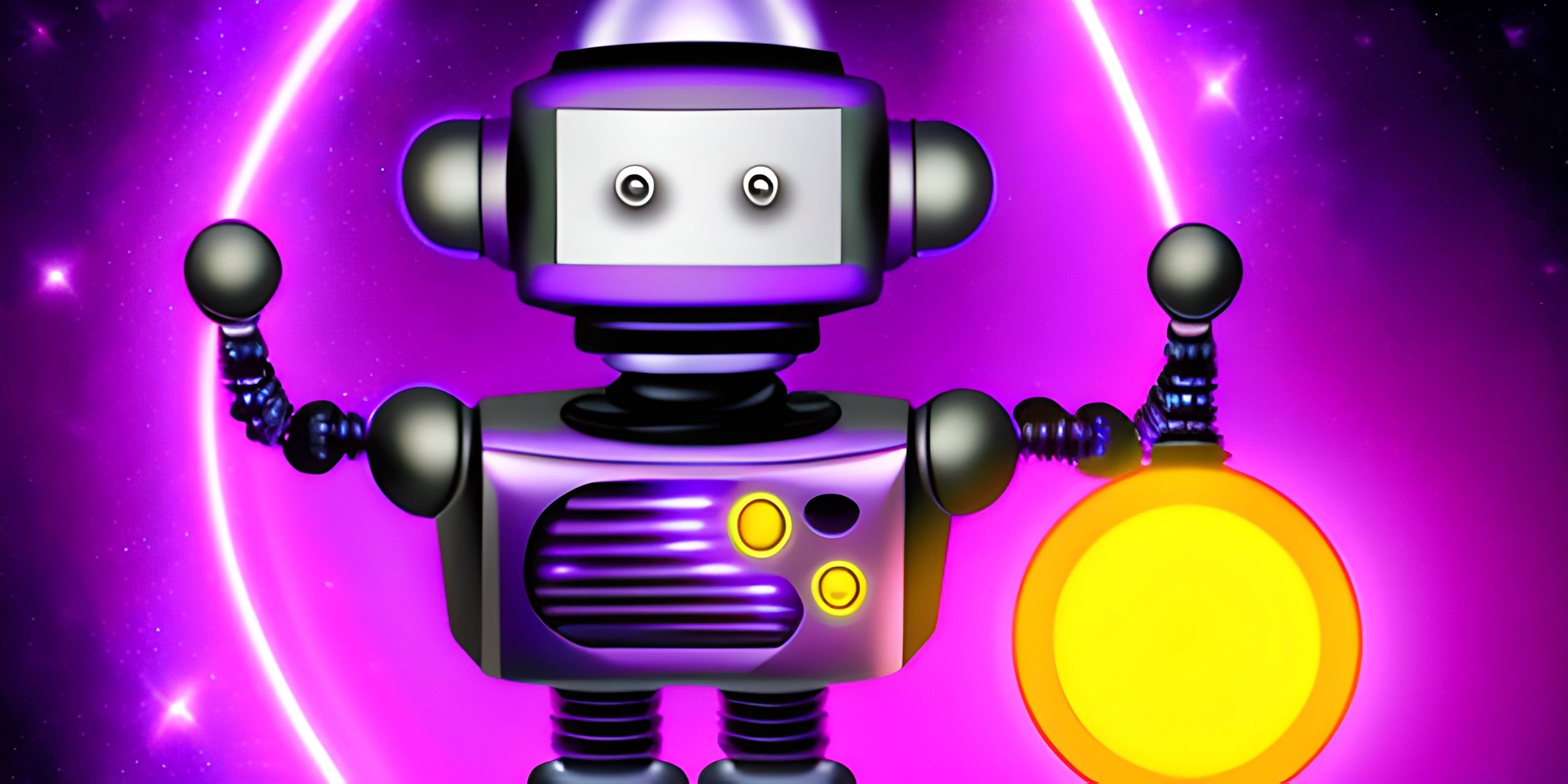
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to making your Discord bot's messages stand out, rich embeds are the way to go. Using Discord.js, we can create messages with colorful sidebars, fields, images, and more. Let's dive into the world of embeds and make your bot's messages look fantastic!
Creating an Embed
To get started, we'll need to install and import the discord.js
library. If you haven't already, install it using npm
:
npm install discord.js
Now, let's import the library and create a new Discord.MessageEmbed()
object:
const Discord = require("discord.js"); const exampleEmbed = new Discord.MessageEmbed();
VoilĂ ! We've created a basic embed object. But it's a bit... bland. Let's give it some flair!
Adding Content to an Embed
Let's start by setting a title, description, and color for our embed:
exampleEmbed.setTitle("Cratecode Bot"); exampleEmbed.setDescription("This is an example of a rich embed!"); exampleEmbed.setColor("#FF5733");
Now our embed has a title, description, and a snazzy orange sidebar. But we can do even more!
Adding Fields
Fields are an excellent way to display information in a clean, organized manner. To add a field, use the addField()
method:
exampleEmbed.addField("Field 1", "This is the content of Field 1."); exampleEmbed.addField("Field 2", "This is the content of Field 2.");
That's it! Now our embed has two fields displaying information. You can add as many fields as needed, but remember to keep it clean and readable.
Adding Images and Thumbnails
Nothing grabs attention like a good image. To add a thumbnail or main image to your embed, use the setThumbnail()
and setImage()
methods:
exampleEmbed.setThumbnail("https://example.com/thumbnail.png"); exampleEmbed.setImage("https://example.com/main-image.png");
With those lines, we've added a thumbnail and a main image to our embed. Make sure to use valid image URLs!
Sending the Embed
Now that we've crafted our beautiful embed, it's time to send it. You can send an embed just like a regular message:
channel.send({ embeds: [exampleEmbed] });
Replace channel
with the appropriate channel object in your bot's code.
And that's it! Your bot has now sent a visually appealing rich embed using Discord.js. Keep experimenting with different properties and methods to make your bot's messages truly unique! For more information on the various properties and methods available, consult the Discord.js documentation.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is a Rich Embed in Discord.js?
A Rich Embed in Discord.js is a special type of message that contains more visual elements and structure than a standard text message. With rich embeds, you can create visually appealing and organized messages, which may include images, thumbnails, colors, and fields that help display information more effectively.
How do I create a Rich Embed using Discord.js?
To create a Rich Embed using Discord.js, you need to use the MessageEmbed
class. Here's an example of how to create a basic embed:
const { MessageEmbed } = require("discord.js"); const exampleEmbed = new MessageEmbed() .setColor('#0099ff') .setTitle('Example Embed') .setDescription('This is a sample description') .setThumbnail('https://example.com/thumbnail.png') .addField('Field 1', 'Content of field 1') .addField('Field 2', 'Content of field 2');
How do I send a Rich Embed message in Discord.js?
Once you've created a Rich Embed using the MessageEmbed
class, you can send it as a message by calling the send()
method on a text channel object. Here's an example:
// Assuming you have a text channel object named 'channel' channel.send({ embeds: [exampleEmbed] });
Can I add images to my Rich Embed message in Discord.js?
Yes, you can add images to your Rich Embed messages using the .setImage()
and .setThumbnail()
methods of the MessageEmbed
class. Pass the URL of the image as an argument to these methods like this:
const exampleEmbed = new MessageEmbed() .setImage('https://example.com/image.png') .setThumbnail('https://example.com/thumbnail.png');
How do I customize the appearance of my Rich Embed message in Discord.js?
You can customize the appearance of your Rich Embed message using various methods of the MessageEmbed
class, such as .setColor()
, .setTitle()
, .setDescription()
, .setThumbnail()
, and .addField()
. Each method allows you to modify a specific aspect of the embed, like the color, title, description, thumbnail, and fields, respectively.