Discord.js - Responding to Messages
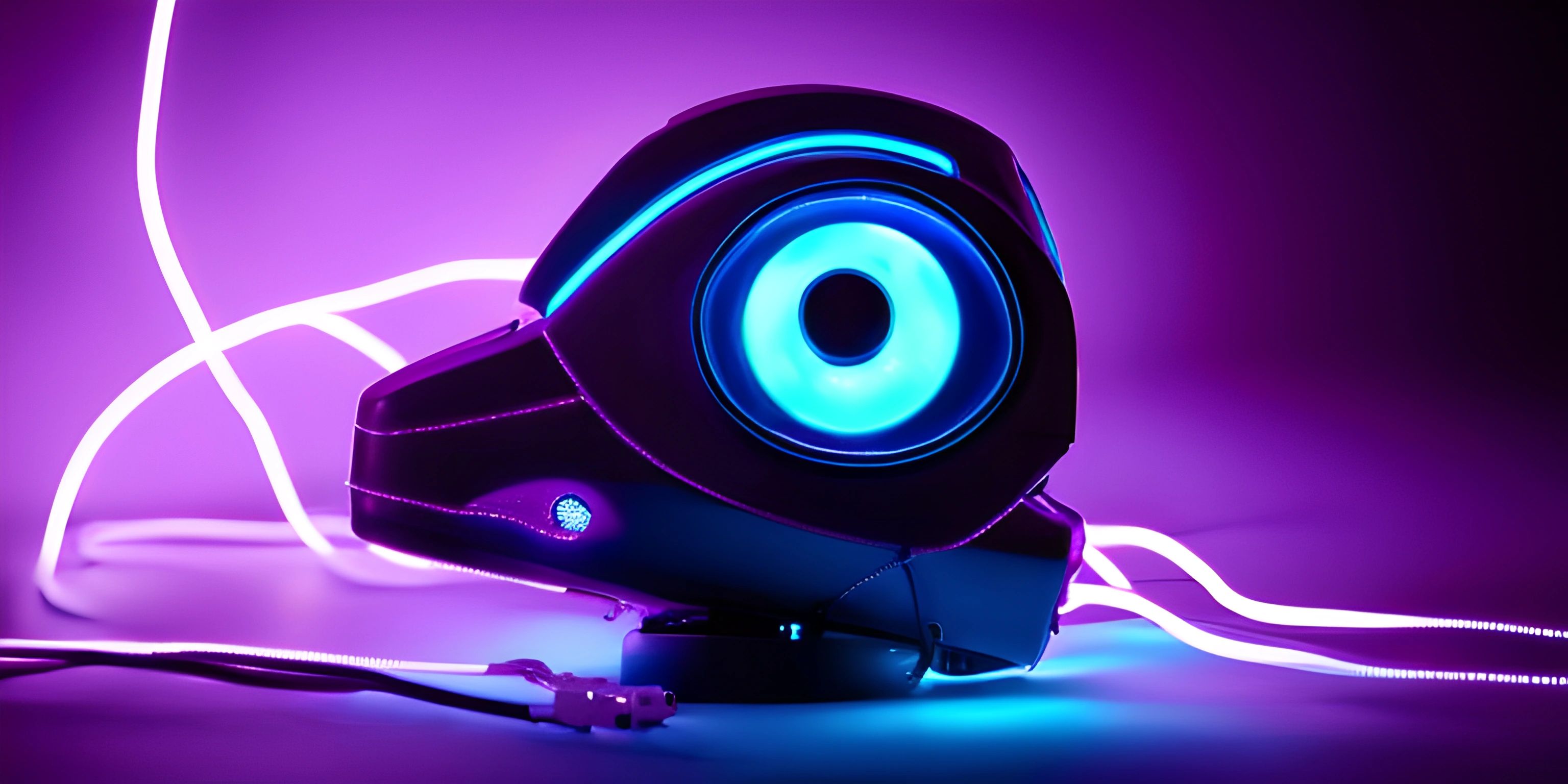
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When building a Discord bot using Discord.js, one of the most common tasks is listening for and responding to messages. Luckily, Discord.js makes this process straightforward, allowing your bot to interact with users in no time!
Prerequisites
Before diving into message handling, make sure you have the following:
- A basic understanding of Node.js.
- Discord.js installed and set up in a project.
- A Discord bot created and added to your server.
Listening for Messages
To listen for messages, we'll use the message
event provided by the Client
class in Discord.js. First, let's import the necessary library and create a Client
instance:
const { Client } = require("discord.js"); const client = new Client();
Next, add an event listener for the message
event, which will be fired every time a new message is sent in a channel the bot has access to:
client.on("message", (message) => { console.log(`Received message: ${message.content}`); });
Now, when the bot receives a message, it will log the message content to the console. Make sure to replace YOUR_BOT_TOKEN
with your bot's token, and start the bot by running node index.js
:
client.login("YOUR_BOT_TOKEN");
Responding to Messages
To make the bot respond to specific messages, add a conditional statement inside the event listener. For example, let's make the bot reply with "Hello, I'm a bot!" when someone sends a message containing the word "bot":
client.on("message", (message) => { if (message.content.toLowerCase().includes("bot")) { message.channel.send("Hello, I'm a bot!"); } });
Remember to check that the message author is not the bot itself, as this can cause an infinite loop of messages:
client.on("message", (message) => { // Ignore messages from the bot itself if (message.author.bot) return; if (message.content.toLowerCase().includes("bot")) { message.channel.send("Hello, I'm a bot!"); } });
Handling Commands
For more advanced functionality, you might want to implement commands that users can trigger with specific keywords or phrases. To achieve this, split the message content into an array of words and check the first word for a specific command. For example, let's make the bot respond to a "!greet" command:
client.on("message", (message) => { if (message.author.bot) return; const words = message.content.split(" "); const command = words[0].toLowerCase(); if (command === "!greet") { message.channel.send("Hello, welcome to the server!"); } });
Now you know how to listen for and respond to messages in Discord.js! With these basics in place, you can expand your bot's functionality and create engaging interactions for your Discord community. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
How do I make my Discord bot listen for messages using Discord.js?
To make your Discord bot listen for messages using Discord.js, you need to create an event listener for the "message" event. Here's a basic example in JavaScript:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("message", (message) => { // Your code to handle the message goes here }); client.login("your_bot_token");
Replace "your_bot_token" with the token of your Discord bot.
How do I send a response to a specific message in Discord.js?
To send a response to a specific message, you can use the message.reply()
method inside the "message" event listener. For example:
client.on("message", (message) => { if (message.content === "!ping") { message.reply("Pong!"); } });
In this example, your bot will reply "Pong!" whenever someone sends a message containing "!ping".
How do I prevent the bot from responding to its own messages?
To prevent your bot from responding to its own messages, you can add a condition to check if the message author is the bot itself. If it is, just return and do nothing:
client.on("message", (message) => { // Ignore messages from the bot itself if (message.author.bot) return; // Your code to handle the message goes here });
How can I add custom commands to my Discord bot using Discord.js?
To add custom commands, you can use a conditional statement to check if the message content matches a specific command. For example:
client.on("message", (message) => { // Ignore messages from the bot itself if (message.author.bot) return; // Check for specific commands and respond accordingly if (message.content === "!hello") { message.reply("Hello there!"); } else if (message.content === "!goodbye") { message.reply("Goodbye! Have a great day!"); } });
Can I make my Discord bot respond to messages with mentions?
Yes, you can make your Discord bot respond to messages with mentions. You can check if the message mentions your bot using the message.mentions.users
property. For example:
client.on("message", (message) => { // Ignore messages from the bot itself if (message.author.bot) return; // Check if the bot is mentioned if (message.mentions.users.has(client.user.id)) { message.reply("You mentioned me!"); } });