Fundamentals of Divide and Conquer Algorithms
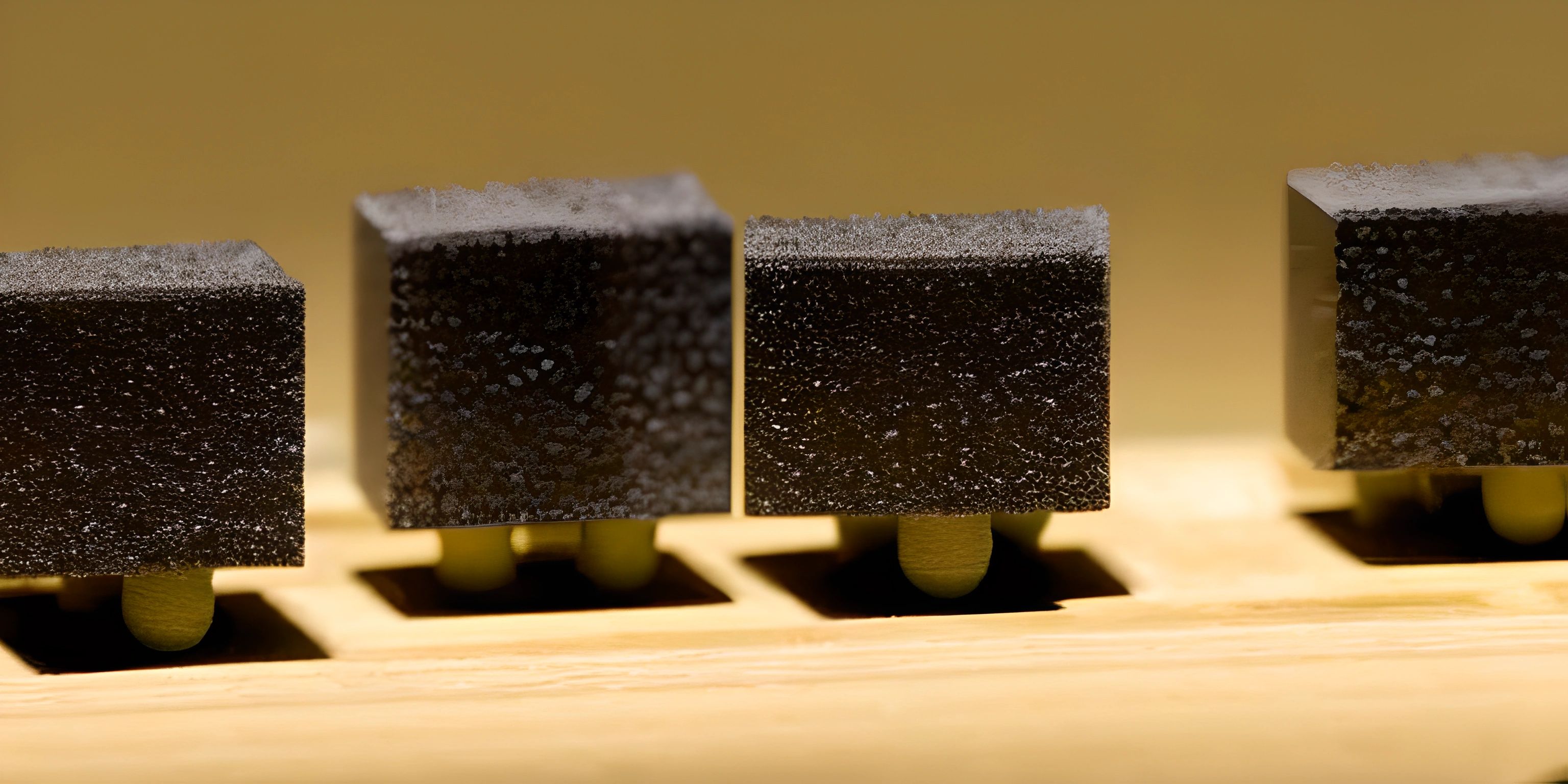
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Divide and conquer is a powerful problem-solving technique used in computer programming. Its essence lies in breaking down a complex problem into smaller, more manageable subproblems. By repeatedly dividing the problem and tackling the subproblems, we eventually conquer the original challenge. This technique is often used with recursive functions, making it an elegant and efficient approach to problem-solving.
How Divide and Conquer Works
Let's dive into the process of divide and conquer. It generally consists of three main steps:
- Divide: Break the problem into smaller subproblems.
- Conquer: Solve the smaller subproblems, either directly or recursively.
- Combine: Merge the solutions of the subproblems to obtain the final solution.
A classic example of a divide and conquer algorithm is the merge sort, an efficient sorting algorithm that recursively divides an array into halves, sorts the halves, and then merges them back together.
Advantages of Divide and Conquer
Divide and conquer algorithms offer several benefits:
- Efficiency: By breaking problems down into smaller parts, divide and conquer algorithms can often reduce the time complexity of a problem, leading to faster execution.
- Simplicity: Complex problems can be made simpler by breaking them down into smaller, more manageable subproblems.
- Parallelism: Divide and conquer algorithms often lend themselves well to parallelization, where multiple subproblems can be solved simultaneously, further increasing efficiency.
Divide and Conquer in Action
Let's take a look at a simple example to better understand how divide and conquer algorithms work. We'll implement a binary search algorithm to find the position of a target value within a sorted array:
def binary_search(arr, target): return binary_search_helper(arr, target, 0, len(arr) - 1) def binary_search_helper(arr, target, left, right): if left > right: return -1 mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: return binary_search_helper(arr, target, mid + 1, right) else: return binary_search_helper(arr, target, left, mid - 1)
In this example, the binary_search
function uses divide and conquer to locate the target value. It first checks the middle element of the array. If the middle element is not the target, the function is recursively called on either the left or the right half of the array, depending on whether the target is greater or smaller than the middle element. The process continues until the target is found or the search space is empty.
As you can see, divide and conquer algorithms are a powerful approach to problem-solving in computer programming. They help to simplify complex problems, improve efficiency, and can often be parallelized. With a solid understanding of the fundamentals, you'll be well-equipped to tackle a wide range of programming challenges using divide and conquer techniques.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What are divide and conquer algorithms?
Divide and conquer algorithms are a problem-solving approach in computer programming that works by breaking a problem into smaller subproblems, solving those subproblems, and finally combining their solutions to get the solution for the original problem. This method is efficient in handling large and complex problems and is widely used in various programming domains.
How do divide and conquer algorithms work?
Divide and conquer algorithms work in three main steps:
- Divide: Break the given problem into smaller subproblems.
- Conquer: Solve the smaller subproblems, either by directly solving them or by applying the same divide and conquer approach recursively.
- Combine: Merge the solutions of the subproblems to obtain the solution for the original problem.
Can you provide an example of a divide and conquer algorithm?
Sure! One famous example of a divide and conquer algorithm is the Merge Sort algorithm. It's a sorting technique that follows these steps:
- Divide: Split the unsorted array into two equal halves.
- Conquer: Recursively apply the Merge Sort algorithm to each half until each half has only one element.
- Combine: Merge the sorted halves by comparing and placing elements in the correct order.
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = merge_sort(arr[:mid]) right = merge_sort(arr[mid:]) return merge(left, right) def merge(left, right): result = [] i = j = 0 while i < len(left) and j < len(right): if left[i] < right[j]: result.append(left[i]) i += 1 else: result.append(right[j]) j += 1 result.extend(left[i:]) result.extend(right[j:]) return result
Are there any disadvantages to using divide and conquer algorithms?
While divide and conquer algorithms can be highly efficient and effective at solving complex problems, they may not be suitable for all situations. Some potential disadvantages include:
- Increased memory usage: In some cases, divide and conquer algorithms may require additional memory to store the subproblems and intermediate results.
- Complexity: The algorithm can be more complex to understand and implement compared to other problem-solving approaches, especially for beginners.
Are there any alternatives to divide and conquer algorithms?
Yes, there are other problem-solving approaches in computer programming, such as dynamic programming, greedy algorithms, and backtracking. The choice of the most suitable method depends on the specific problem, its constraints, and the desired performance characteristics. It's essential to evaluate different approaches and choose the one that best fits the problem at hand.