Quick Sort
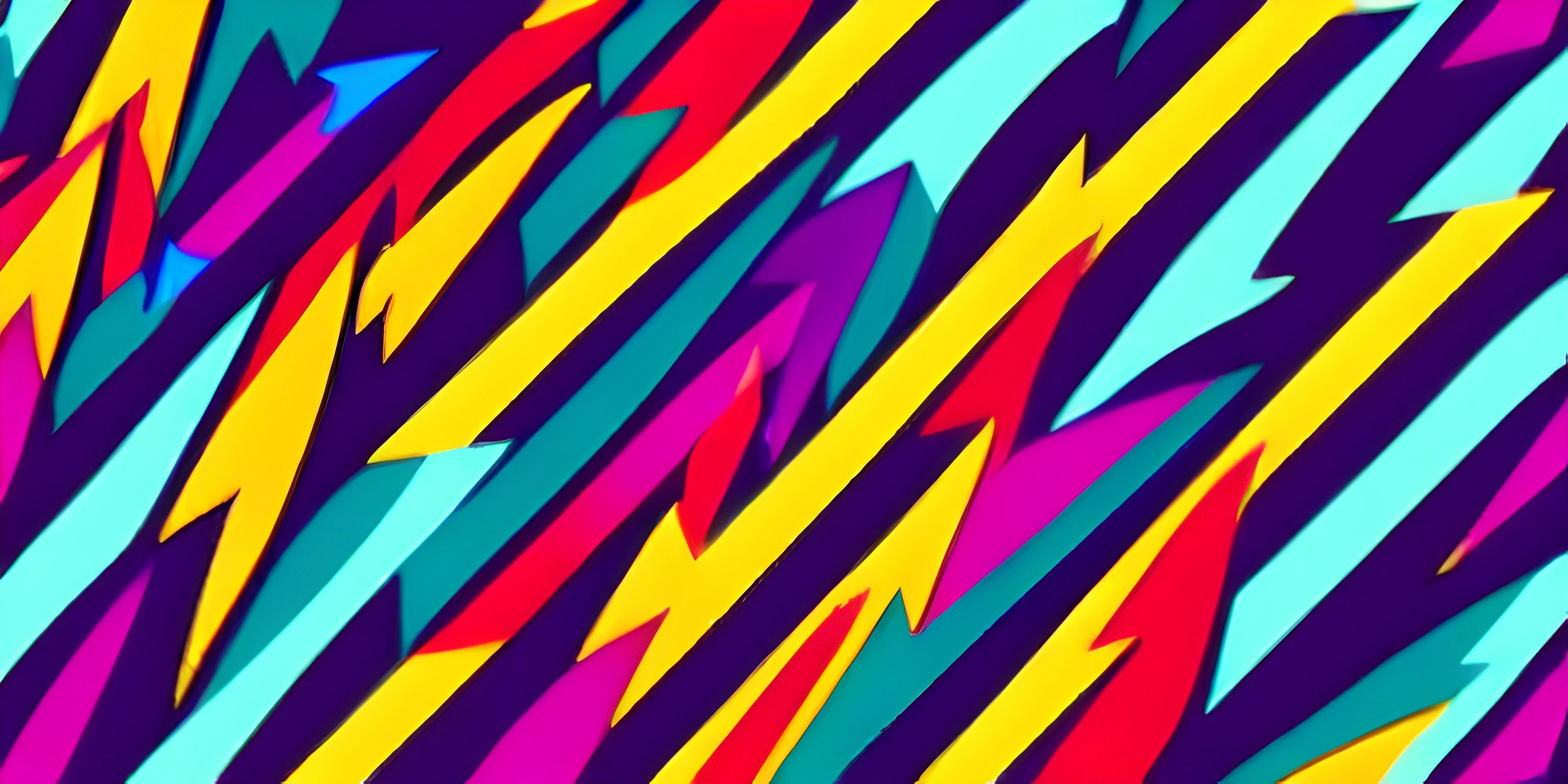
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting arrays is an essential task in many programming applications. One popular and efficient sorting algorithm is the Quick Sort. It's a divide-and-conquer algorithm that works by selecting a 'pivot' element from the array and partitioning the other elements into two groups, one with values less than the pivot and the other with values greater than the pivot. Then, the algorithm recursively sorts the two groups.
How Quick Sort Works
Here's a step-by-step explanation of the Quick Sort algorithm:
-
Choose a pivot element. There are several strategies for selecting the pivot, such as choosing the first, last, or middle element, or selecting it randomly.
-
Partition the array into two groups: one with elements less than the pivot and the other with elements greater than the pivot.
-
Recursively apply Quick Sort to both groups.
-
Combine the sorted groups and the pivot to obtain the sorted array.
Let's see an example in action:
array = [3, 8, 2, 5, 1, 4, 7, 6]
We'll select the first element as the pivot (3). After partitioning, we get:
less_than_pivot = [2, 1] pivot = 3 greater_than_pivot = [8, 5, 4, 7, 6]
Now we recursively apply Quick Sort to the two groups:
less_than_pivot_sorted = [1, 2] greater_than_pivot_sorted = [4, 5, 6, 7, 8]
Finally, we combine the groups and the pivot to get the sorted array:
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8]
Advantages of Quick Sort
Quick Sort has several advantages over other sorting algorithms:
-
Efficiency: Quick Sort's average case time complexity is O(n log n), making it faster than many other sorting algorithms like Bubble Sort and Insertion Sort.
-
In-place sorting: Unlike Merge Sort, Quick Sort doesn't require additional memory for temporary arrays, as it sorts the input array in-place.
-
Adaptability: Quick Sort can be easily adapted to sort different data types and customized with various pivot selection strategies.
However, Quick Sort's worst-case time complexity is O(n²), which can occur when the pivot selection is poor (e.g., always choosing the first or last element in a pre-sorted array). To mitigate this, you can use a randomized pivot selection strategy or switch to a different sorting algorithm like Heap Sort for large datasets.
Now that you've got a grasp on the Quick Sort algorithm and its benefits, you're ready to implement it in your programming language of choice, and optimize your sorting tasks like a pro!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).