Elixir Functions and Modules
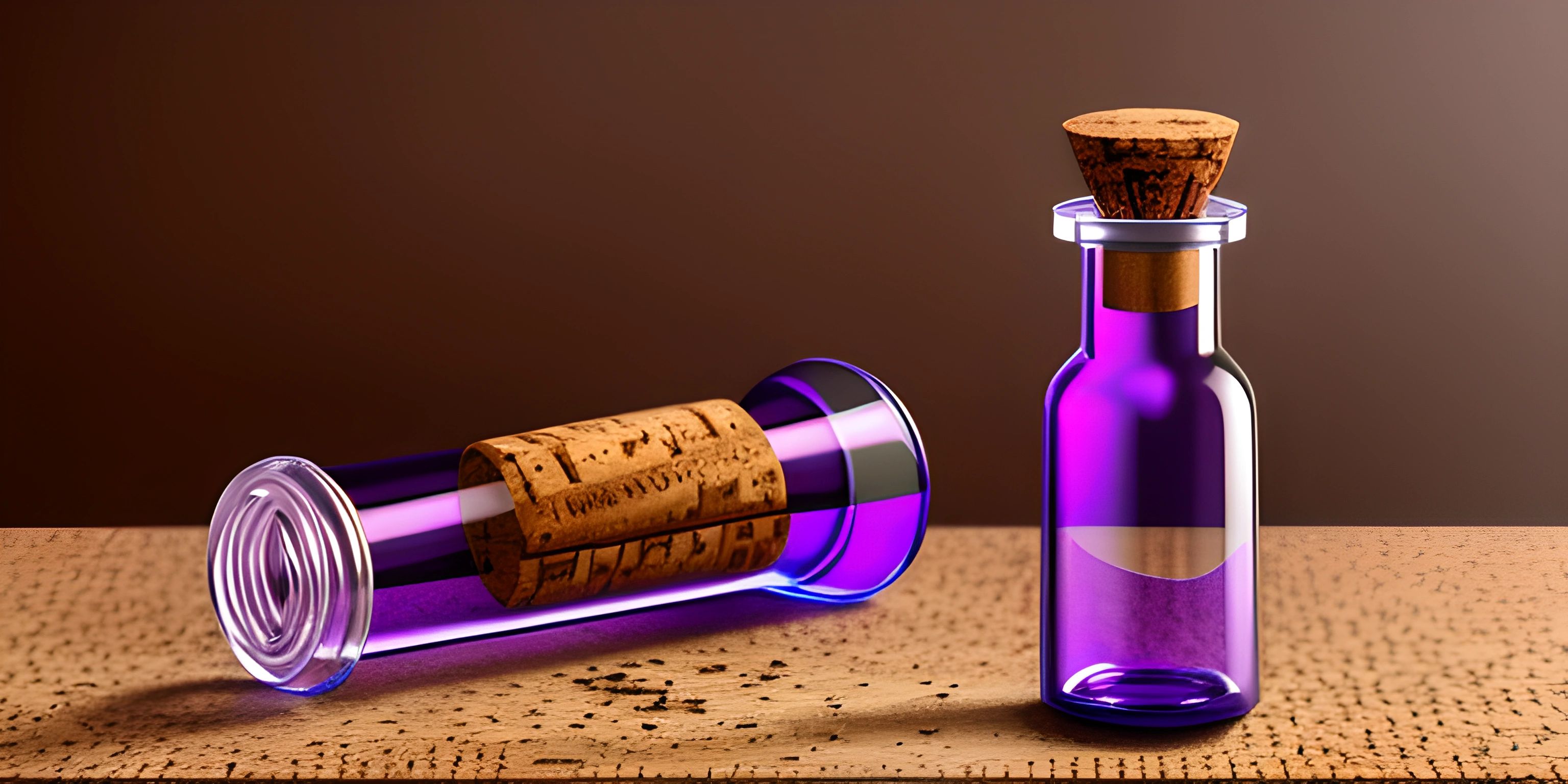
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When diving into the world of Elixir, two essential concepts to understand are functions and modules. Elixir is a functional programming language, which means functions are the lifeblood of Elixir code. In this article, we'll explore functions, both anonymous and named, as well as modules and how they help organize and structure your Elixir projects.
Functions in Elixir
In Elixir, functions are first-class citizens, which means they can be passed around, assigned to variables, and used just like any other value. There are two types of functions in Elixir: anonymous functions and named functions.
Anonymous Functions
Anonymous functions, as the name suggests, don't have a name. They are defined using the fn
keyword and can be assigned to variables. To invoke an anonymous function, the .
operator is used. Here's an example to help illustrate this concept:
# Define an anonymous function to add two numbers add = fn a, b -> a + b end # Invoke the anonymous function result = add.(1, 2) IO.puts("The result is #{result}") # The result is 3
In the example above, we define an anonymous function that takes two arguments and returns their sum. We then assign this function to the variable add
. To call the function, we use the .
operator, as seen in add.(1, 2)
.
Named Functions
Named functions, on the other hand, are functions with a specific name, and they must be defined inside a module. We'll discuss modules in more detail in the next section, but for now, let's see how to define a named function:
defmodule Math do def add(a, b) do a + b end end result = Math.add(1, 2) IO.puts("The result is #{result}") # The result is 3
In this example, we define a named function called add
inside the Math
module. The named function can be called using the module name followed by the function name, like Math.add(1, 2)
.
Modules in Elixir
Modules are the building blocks of Elixir code, providing a way to organize and structure your functions. They play a crucial role in managing the complexity of larger projects. Modules are defined using the defmodule
keyword, and named functions are defined within them. Let's explore an example:
defmodule Greetings do def hello(name) do "Hello, #{name}!" end def goodbye(name) do "Goodbye, #{name}." end end IO.puts(Greetings.hello("Alice")) # Hello, Alice! IO.puts(Greetings.goodbye("Bob")) # Goodbye, Bob.
In this example, we define a Greetings
module that contains two named functions: hello
and goodbye
. These functions can be accessed using the module name followed by the function name, as seen in the IO.puts
calls.
Conclusion
Elixir relies heavily on functions and modules for code organization and structure. Understanding how to work with anonymous and named functions, as well as modules, is fundamental to becoming proficient in Elixir. By mastering these concepts, you'll be well on your way to writing clean, maintainable, and efficient Elixir code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).