Exploring Anonymous Functions in JavaScript
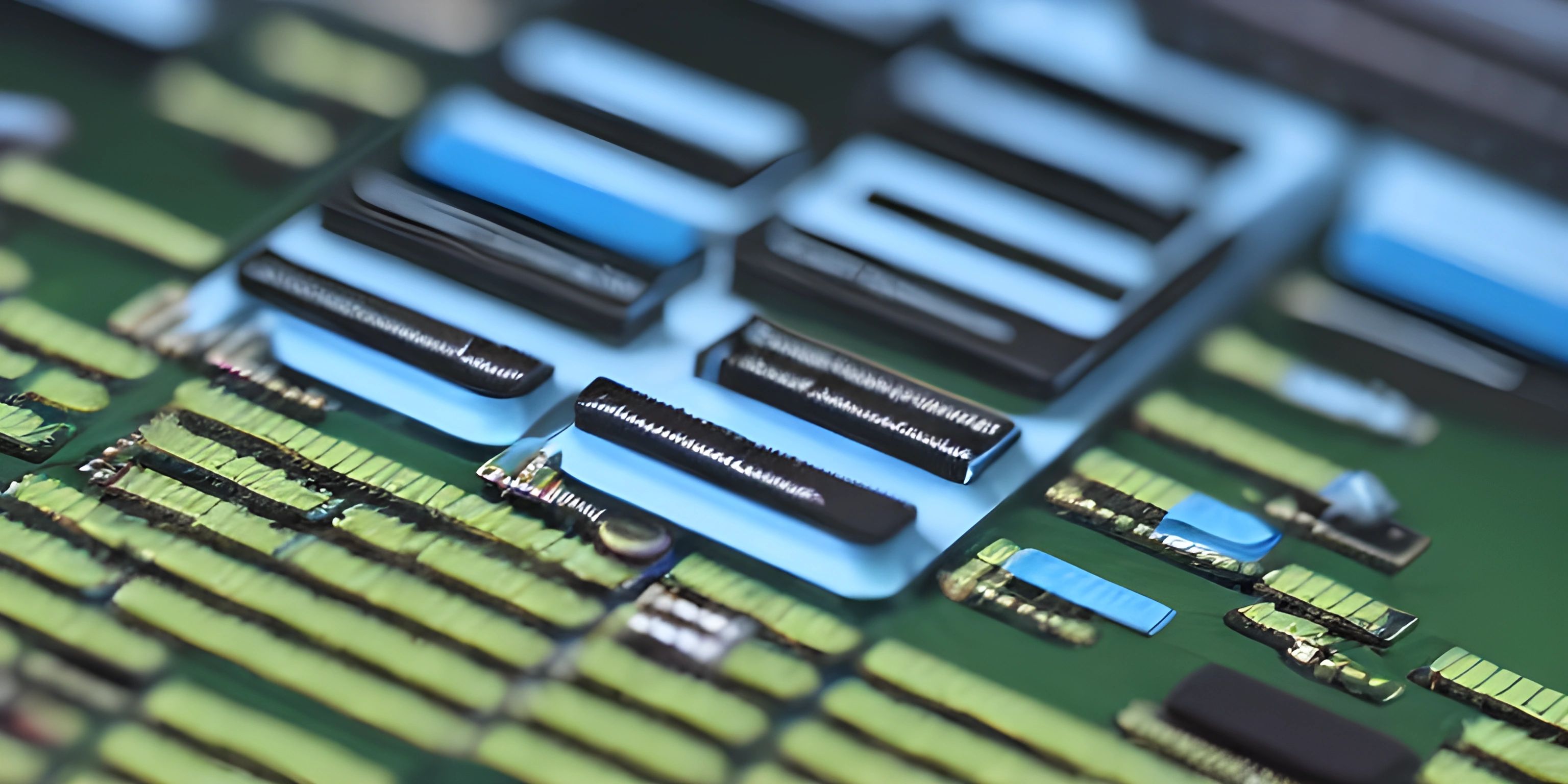
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Anonymous functions in JavaScript can be a bit of a mystery at first, but they're incredibly powerful tools once you get the hang of them. Picture this: you're at an amusement park, and you see a roller coaster with no name, just a series of wild loops and twists. That's an anonymous function! It's a function without a name, ready to jump into action wherever you place it.
What Are Anonymous Functions?
An anonymous function is a function that doesn't have a name. Instead of naming the function, you can define it inline. These functions are often used as arguments to other functions or as shorter alternatives to full function declarations.
Basic Syntax
Here's a quick example to illustrate an anonymous function in action:
setTimeout(function() { console.log("Hello, world!"); }, 1000);
In the code above, we pass an anonymous function to setTimeout
. This function will run after 1000 milliseconds, printing "Hello, world!" to the console. Notice that the function doesn't have a name.
Why Use Anonymous Functions?
Anonymous functions are like ninjas in the world of programming—they can sneak into your code, perform their task, and vanish without a trace. Here are some reasons why you might want to use them:
- Conciseness: They make your code shorter and cleaner.
- Convenience: Handy for one-off functions that you don't need to call elsewhere.
- Scope: They can help manage variable scope, especially within closures and IIFEs.
Example: Array Methods
A common use for anonymous functions is with array methods like map
, filter
, and reduce
.
let numbers = [1, 2, 3, 4, 5]; let doubled = numbers.map(function(num) { return num * 2; }); console.log(doubled); // [2, 4, 6, 8, 10]
In the example above, we're using an anonymous function to double each number in the array. The map
method takes this function and applies it to every element in the array.
Arrow Functions
JavaScript ES6 introduced a more concise way to write anonymous functions called arrow functions. Arrow functions provide a shorter syntax and automatically bind the this
value.
Arrow Function Syntax
Here's how you can rewrite the previous example using an arrow function:
let doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6, 8, 10]
With arrow functions, you can omit the function
keyword and the curly braces if the function has a single statement.
Anonymous Functions in Callbacks
One of the most common places you'll see anonymous functions is in callbacks. Callbacks are functions that are passed as arguments to other functions and are executed after some operation is completed.
Example: Event Listeners
When working with event listeners, you often use anonymous functions as callbacks:
document.getElementById("myButton").addEventListener("click", function() { alert("Button was clicked!"); });
In this example, an anonymous function is used as a callback for the "click" event on a button. When the button is clicked, the anonymous function runs, showing an alert.
Closures
Anonymous functions are often used to create closures. A closure is a function that retains access to its lexical scope, even when the function is executed outside that scope.
Example: Closure with Anonymous Function
Here's an example of a closure created with an anonymous function:
function makeCounter() { let count = 0; return function() { count++; return count; }; } let counter = makeCounter(); console.log(counter()); // 1 console.log(counter()); // 2
In this example, the anonymous function returned by makeCounter
maintains access to the count
variable, even after makeCounter
has finished executing.
Immediately Invoked Function Expressions (IIFEs)
An IIFE is an anonymous function that runs as soon as it's defined. IIFEs are useful for creating a new scope to avoid polluting the global scope.
Example: IIFE
Here's how you can create and use an IIFE:
(function() { console.log("This function runs immediately!"); })();
In the code above, the anonymous function is defined and immediately invoked, printing a message to the console.
When Not to Use Anonymous Functions
While anonymous functions are incredibly useful, there are times when named functions are a better choice:
- Debugging: Named functions provide more clarity in stack traces.
- Reusability: If you need to call the function in multiple places, a named function is more appropriate.
- Recursion: For recursive functions, having a name is essential.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What is an anonymous function?
An anonymous function is a function that doesn't have a name. It's often used as an argument to other functions or for short, one-off operations.
What are some common uses for anonymous functions?
They are commonly used in callbacks, array methods like map
, filter
, and reduce
, and for creating closures and IIFEs.
How do arrow functions relate to anonymous functions?
Arrow functions are a more concise way to write anonymous functions, introduced in ES6. They simplify the syntax and automatically bind the this
value.
Can anonymous functions be used for recursive operations?
No, for recursive operations, named functions are necessary because they need a way to refer to themselves.
What are closures, and how do anonymous functions help create them?
Closures are functions that retain access to their lexical scope, even when executed outside that scope. Anonymous functions help create closures when they are defined within another function and maintain access to the variables from that outer function.