Introduction to Fractals
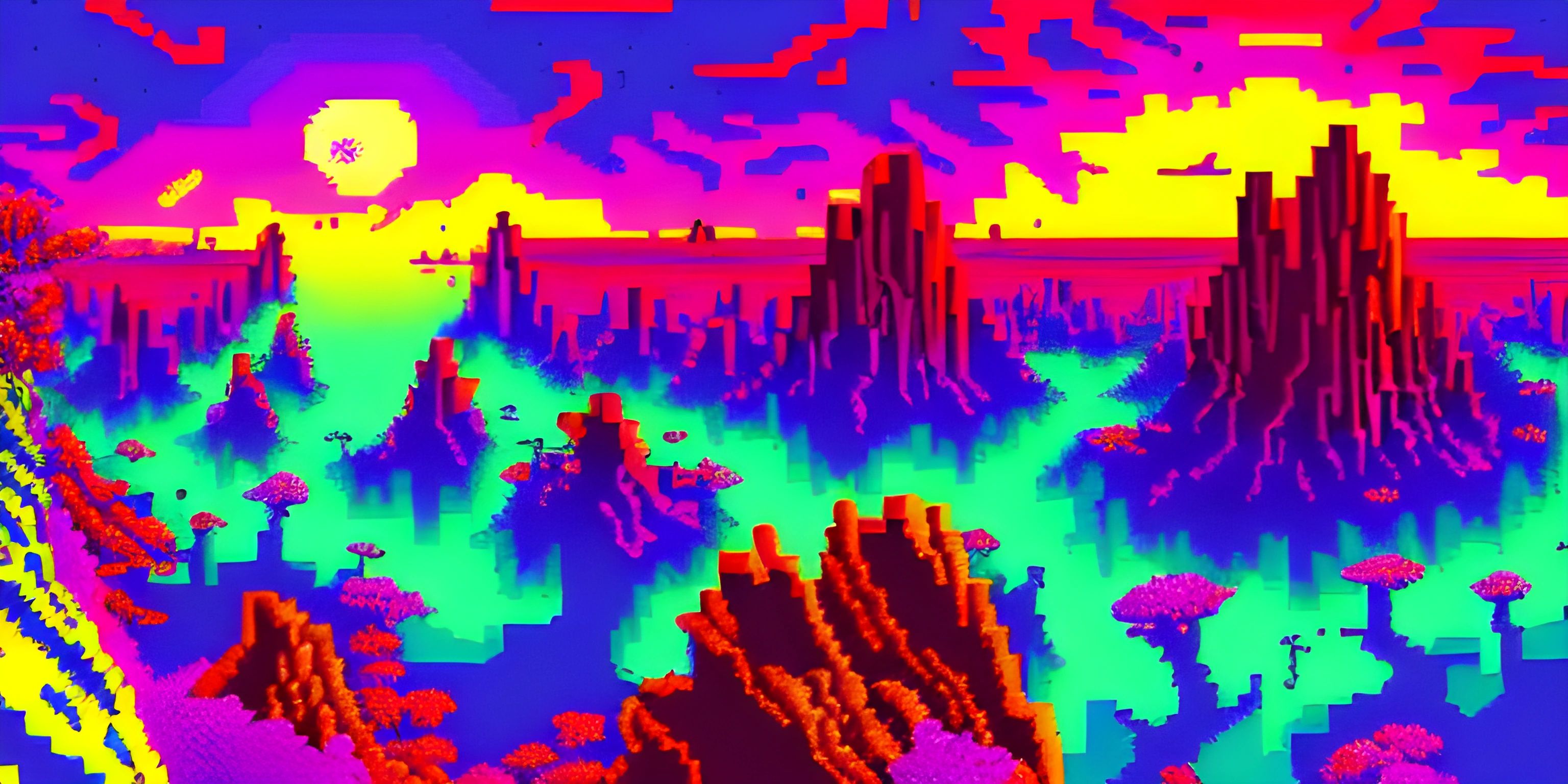
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Fractals are like the magic eye puzzles of the mathematical world. You know, those images where if you stare long enough, a 3D picture emerges? Well, fractals are similar, except instead of a dolphin or spaceship popping out, you get an infinitely intricate and self-similar pattern. Buckle up, because we're diving into a world where math meets art, and complexity meets simplicity.
What Exactly Are Fractals?
At its core, a fractal is a never-ending pattern that repeats itself at different scales. This property is known as self-similarity. Imagine zooming in on a coastline; the closer you get, the more detail you see, but the pattern of the coastline looks similar regardless of your zoom level. This is a key characteristic of fractals.
The Geometry of Fractals
Fractals can be described by fractal geometry, a branch of mathematics that goes beyond traditional Euclidean geometry. While Euclidean geometry deals with shapes like lines, circles, and triangles, fractal geometry explores shapes that have fractional dimensions. Yes, you read that right—fractional dimensions. It's like finding out your favorite pie isn't just cut into slices, but slices of slices, ad infinitum.
The Mandelbrot Set
The Mandelbrot Set is perhaps the most famous fractal. It's defined by a simple equation involving complex numbers:
[ z_{n+1} = z_n^2 + c ]
where ( z ) and ( c ) are complex numbers, and ( n ) is an iteration step. The beauty of the Mandelbrot Set lies in its boundary. No matter how much you zoom in, you keep discovering new structures, each as complex as the last.
Here's some Python code to generate a simple visualization of the Mandelbrot Set:
import numpy as np import matplotlib.pyplot as plt # Define the function for the Mandelbrot Set def mandelbrot(c, max_iter): z = c for n in range(max_iter): if abs(z) > 2: return n z = z * z + c return max_iter # Generate the Mandelbrot Set width, height = 800, 800 max_iter = 256 xmin, xmax = -2, 1 ymin, ymax = -1.5, 1.5 image = np.zeros((height, width)) for x in range(width): for y in range(height): cx = xmin + (x / width) * (xmax - xmin) cy = ymin + (y / height) * (ymax - ymin) c = complex(cx, cy) color = mandelbrot(c, max_iter) image[y, x] = color # Display the image plt.imshow(image, cmap='inferno', extent=(xmin, xmax, ymin, ymax)) plt.colorbar() plt.title("Mandelbrot Set") plt.show()
Iterative Algorithms
Fractals are often generated using iterative algorithms. An iterative algorithm repeatedly applies a mathematical operation to an initial value, refining the result step by step. The Mandelbrot Set is a prime example, but there are many other fractals generated through iterations.
Julia Sets
Julia Sets are closely related to the Mandelbrot Set. Each point in the complex plane is either part of the Julia Set or not, depending on how it behaves under iteration. The formula is similar to the Mandelbrot Set but uses a fixed complex number ( c ) for all points:
[ z_{n+1} = z_n^2 + c ]
Here's some Python code to generate a Julia Set:
# Define the function for the Julia Set def julia(z, c, max_iter): for n in range(max_iter): if abs(z) > 2: return n z = z * z + c return max_iter # Generate the Julia Set c = complex(-0.7, 0.27015) image = np.zeros((height, width)) for x in range(width): for y in range(height): zx = xmin + (x / width) * (xmax - xmin) zy = ymin + (y / height) * (ymax - ymin) z = complex(zx, zy) color = julia(z, c, max_iter) image[y, x] = color # Display the image plt.imshow(image, cmap='inferno', extent=(xmin, xmax, ymin, ymax)) plt.colorbar() plt.title("Julia Set") plt.show()
Real-World Applications
Fractals aren't just mathematical curiosities; they have practical applications in various fields.
Computer Graphics
In computer graphics, fractals are used to generate realistic textures and landscapes. The self-similar nature of fractals makes them perfect for creating natural-looking scenes, from mountains to clouds.
Signal and Image Compression
Fractal compression techniques exploit the self-similarity of images to reduce file sizes without losing much detail. This is particularly useful in medical imaging and satellite imagery.
FAQs
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the main characteristic of a fractal?
The main characteristic of a fractal is self-similarity, meaning the pattern repeats at different scales.
How is the Mandelbrot Set generated?
The Mandelbrot Set is generated using an iterative algorithm involving complex numbers. The formula is ( z_{n+1} = z_n^2 + c ).
What are some real-world applications of fractals?
Fractals are used in computer graphics for realistic textures, in signal and image compression, and even in modeling natural phenomena like coastlines and clouds.
What is a Julia Set?
A Julia Set is a type of fractal related to the Mandelbrot Set, generated using a fixed complex number ( c ) for all points.
Can fractals have fractional dimensions?
Yes, fractals can have fractional dimensions, which means they explore shapes that go beyond traditional Euclidean geometry.