C++ Runtime Errors and Solutions
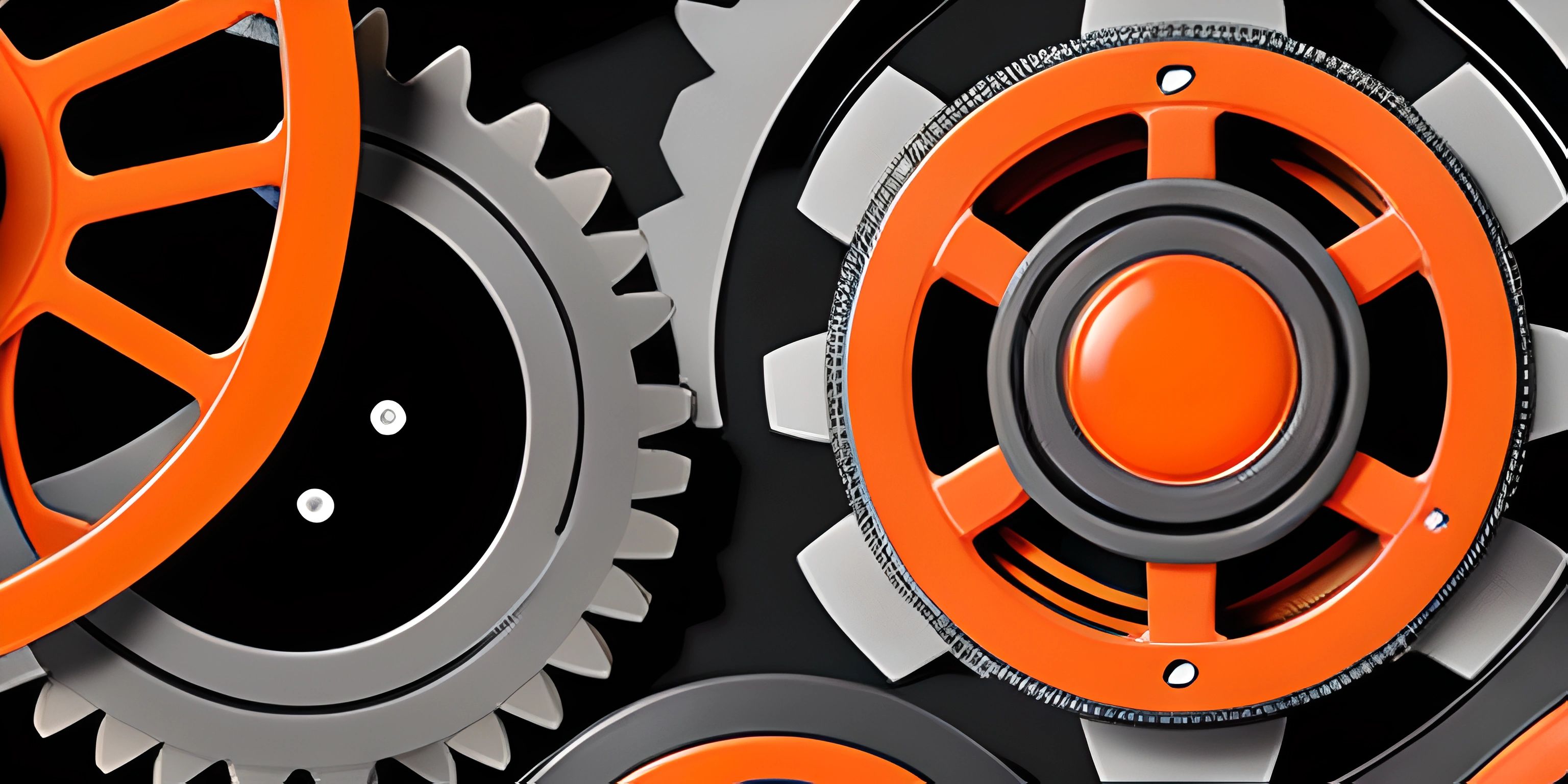
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming in C++ can be a very rewarding experience, but it can also be challenging—especially when faced with runtime errors. These errors are sneaky and can occur during the execution of a program, making them harder to track down than their compile-time counterparts. Fear not, because we're going to dive into some common runtime errors in C++, and explore how to identify and resolve them.
Error 1: Division by Zero
One common error in C++ is division by zero. This occurs when the denominator of a division operation is zero, which is mathematically undefined. When this happens, a program will crash with no mercy.
To fix this error, always ensure that the denominator is not zero before performing division. For example:
int numerator = 10; int denominator = 0; if (denominator != 0) { int result = numerator / denominator; cout << "The result is: " << result << endl; } else { cout << "Cannot divide by zero!" << endl; }
By checking for zero before dividing, we prevent a possible crash and instead provide a helpful message.
Error 2: Null Pointer Dereference
Another common runtime error is null pointer dereference. This occurs when we try to access an object or variable through a pointer that is set to nullptr
, leading to a crash.
To avoid this error, ensure that pointers are initialized to a valid memory location before using them. Additionally, always check for null pointers before accessing their contents. For example:
int* ptr = nullptr; // Allocate memory for the integer before using it ptr = new int; if (ptr != nullptr) { *ptr = 42; cout << "The value is: " << *ptr << endl; } else { cout << "The pointer is null!" << endl; } // Don't forget to free the allocated memory delete ptr;
By checking for nullptr
and allocating memory before using the pointer, we avoid a potential null pointer dereference.
Error 3: Buffer Overflow
Buffer overflow is a runtime error that occurs when a program writes more data to a buffer than it can hold, causing the extra data to overwrite adjacent memory locations. This can lead to crashes, unpredictable behavior, or even security vulnerabilities.
To prevent buffer overflow, always ensure that you stay within the bounds of your arrays and buffers. Use functions like strncpy
instead of strcpy
for copying strings, as the former allows you to control the number of characters copied. For example:
char destination[10]; char source[] = "This is a very long string."; // Use strncpy to copy only the first 9 characters, leaving room for the null terminator strncpy(destination, source, sizeof(destination) - 1); // Ensure the last character is a null terminator destination[sizeof(destination) - 1] = '\0'; cout << "The destination string is: " << destination << endl;
By carefully controlling the amount of data copied, we can prevent buffer overflows and protect our program.
Conclusion
Runtime errors in C++ can be tricky to track down and resolve, but with a bit of diligence and the right debugging techniques, you can overcome them. Remember to always validate your inputs, check for null pointers, and stay within the bounds of your arrays to avoid the most common runtime errors.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a runtime error?
A runtime error is an error that occurs during the execution of a program, as opposed to a compile-time error that occurs during the program's compilation. Runtime errors often involve invalid operations or memory access, leading to crashes or unexpected behavior.
How can I prevent division by zero in C++?
To prevent division by zero, always check that the denominator is not zero before performing a division operation. This can be done with a simple conditional statement that verifies the denominator's value.
What is a null pointer dereference, and how can I avoid it?
A null pointer dereference occurs when you try to access an object or variable through a pointer that is set to nullptr. To avoid this error, always initialize pointers to valid memory locations before using them and check for nullptr before accessing their contents.
What is a buffer overflow, and how can I prevent it?
A buffer overflow is a runtime error that occurs when a program writes more data to a buffer than it can hold, causing the extra data to overwrite adjacent memory locations. To prevent buffer overflow, ensure you stay within the bounds of your arrays and buffers, and use functions like strncpy instead of strcpy for copying strings, as the former allows you to control the number of characters copied.