Genetic Algorithms: Fitness Functions
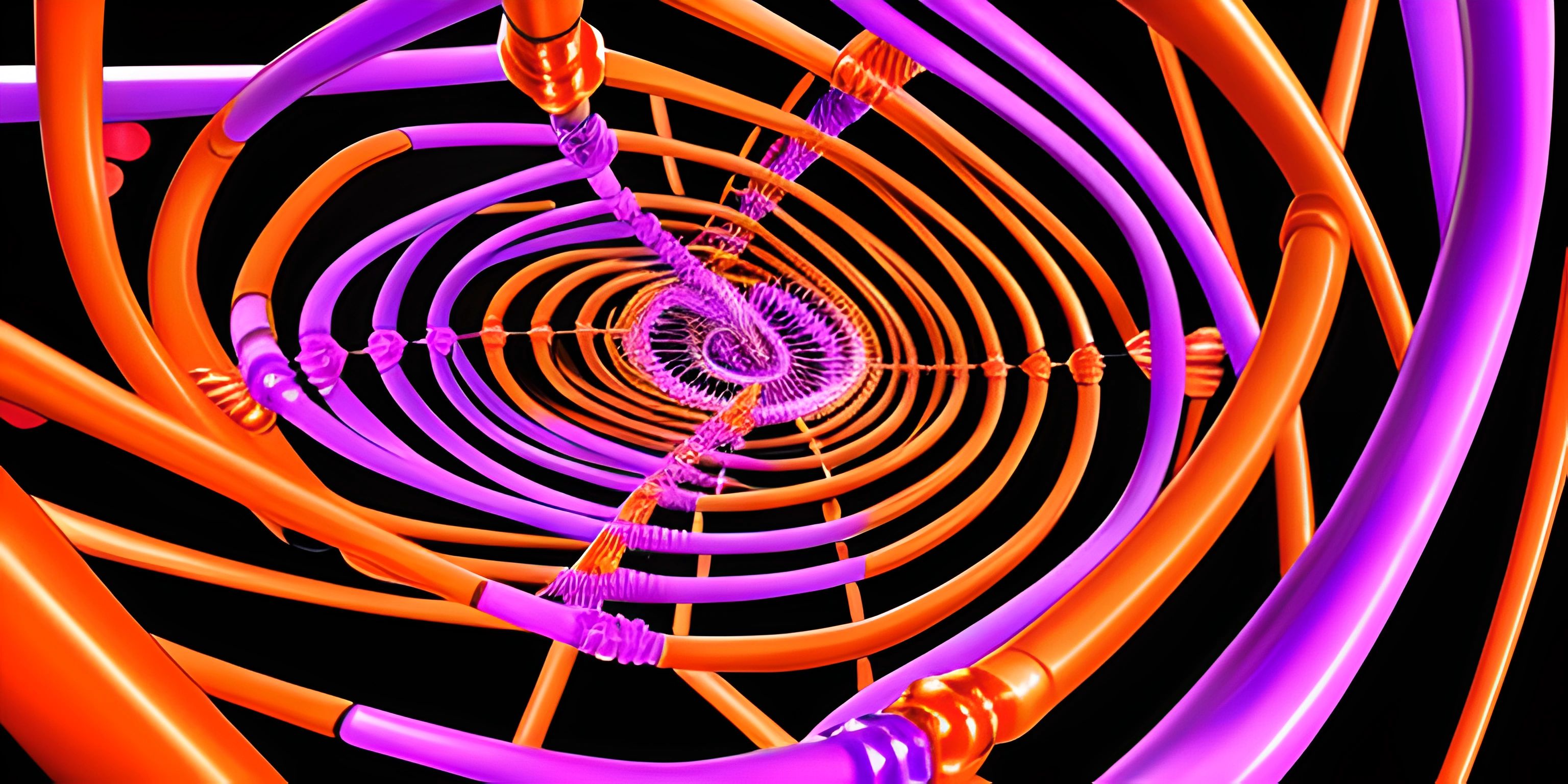
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Genetic algorithms are a powerful optimization technique that mimics the process of natural selection. In order to make these algorithms work their magic, we need a key component: the fitness function. This article will guide you through the process of designing and implementing fitness functions tailored for your specific genetic algorithm problems.
What is a Fitness Function?
A fitness function is a crucial part of a genetic algorithm. It assesses the quality, or "fitness," of candidate solutions (also known as individuals or chromosomes) to a problem. The fitness function is used to rank individuals and helps the algorithm decide which individuals to keep and which to discard during the selection phase.
Designing a Fitness Function
Designing an effective fitness function is crucial for the success of your genetic algorithm. Here are a few guidelines to follow when creating one:
-
Be Problem-Specific: Your fitness function should be tailored to the specific problem you're trying to solve. It should capture the essence of the problem and reflect the objectives you want to achieve.
-
Reward Good Performance: The fitness function should differentiate between good and bad solutions, assigning higher fitness scores to better solutions, and lower scores to worse solutions.
-
Avoid Local Optima: A good fitness function should help your genetic algorithm escape local optima – a suboptimal solution that appears optimal within a limited search space. Design your fitness function to encourage exploration of the search space, avoiding premature convergence.
-
Be Efficient: The fitness function may be called thousands or millions of times during the algorithm's execution. Ensure that it's computationally efficient to avoid slowing down the algorithm.
Implementing a Fitness Function
Now that we know what makes a good fitness function, let's dive into implementing one. We'll use Python to create a simple fitness function for the classic Traveling Salesman Problem (TSP).
In the TSP, the goal is to find the shortest possible route for a salesman to visit a set of cities and return to the starting city. Our fitness function will score an individual's route based on the total distance traveled.
import math def distance(city1, city2): """Calculate the Euclidean distance between two cities.""" x_diff = city1[0] - city2[0] y_diff = city1[1] - city2[1] return math.sqrt(x_diff ** 2 + y_diff ** 2) def tsp_fitness(individual, cities): """Evaluate the fitness of an individual's route for the TSP.""" # Calculate the total distance traveled in the route total_distance = sum(distance(cities[individual[i]], cities[individual[i+1]]) for i in range(len(individual)-1)) # Add the distance from the last city back to the starting city total_distance += distance(cities[individual[-1]], cities[individual[0]]) # We want to minimize the distance, so we'll use the inverse as the fitness score return 1 / total_distance
With this fitness function, our genetic algorithm can now evaluate and rank individuals based on their performance in solving the TSP. Remember, a higher fitness score indicates a better solution.
Conclusion
Designing and implementing an effective fitness function is vital for the success of your genetic algorithm. Keep in mind the guidelines mentioned above and tailor your fitness function to your specific problem. With a well-designed fitness function in hand, you'll be well on your way to harnessing the power of genetic algorithms for optimization and problem-solving. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).