Understanding the Traveling Salesman Problem
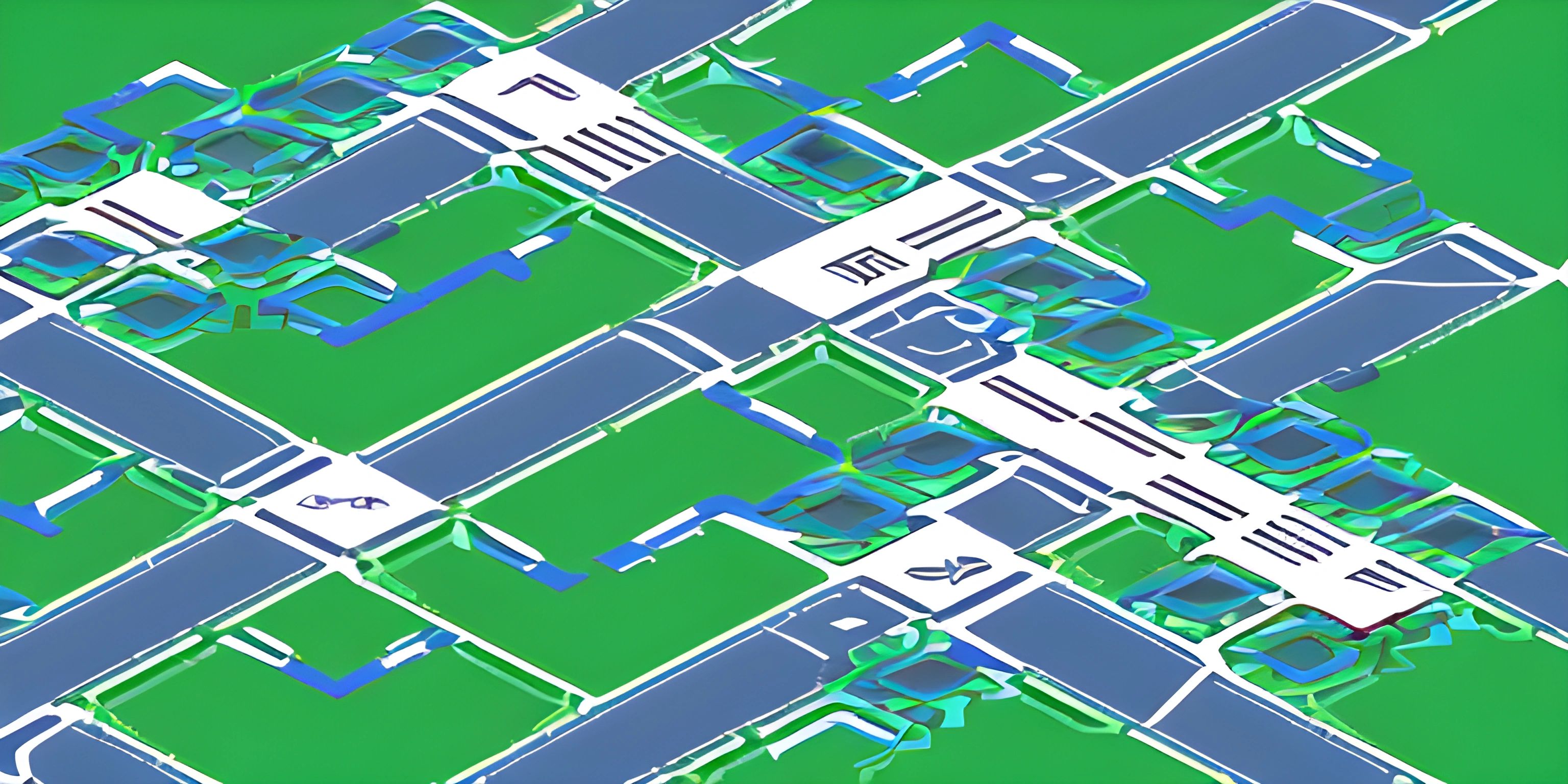
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a traveling salesman with a knack for adventure and a wallet that’s allergic to unnecessary expenses. You need to visit several cities, but you want to take the shortest possible route and return home without repeating any city. This is the crux of the Traveling Salesman Problem (TSP), a classic optimization conundrum that has puzzled mathematicians, computer scientists, and logistics experts for decades.
What is the Traveling Salesman Problem (TSP)?
In a nutshell, the Traveling Salesman Problem asks: Given a list of cities and the distances between each pair, what is the shortest possible route that visits each city exactly once and returns to the origin city?
Despite its seemingly simple premise, TSP is a NP-hard problem, meaning it’s incredibly difficult to solve efficiently as the number of cities increases. The simplicity of its question belies the complexity of its solutions.
Why is TSP Important?
TSP isn't just a theoretical exercise. It has practical applications in fields like logistics, manufacturing, and even DNA sequencing. For instance, delivery companies use TSP algorithms to optimize their routes, saving time and fuel. Likewise, circuit board manufacturers use TSP to minimize the length of wiring. In essence, solving TSP can lead to significant cost reductions and efficiency improvements.
Approaches to Solve TSP
Let's dive into some of the popular methods used to tackle the Traveling Salesman Problem. Each approach has its strengths and weaknesses, and the choice of method often depends on the specific requirements and constraints of the problem.
Brute Force
The brute force method involves calculating the total distance of every possible route and selecting the shortest one. While this guarantees the optimal solution, it becomes impractical for large numbers of cities due to its factorial time complexity, O(n!).
import itertools # List of cities cities = ["A", "B", "C", "D"] # Distance matrix distances = { ("A", "B"): 10, ("A", "C"): 15, ("A", "D"): 20, ("B", "C"): 35, ("B", "D"): 25, ("C", "D"): 30 } def calculate_route_distance(route): distance = 0 for i in range(len(route) - 1): distance += distances.get((route[i], route[i+1]), distances.get((route[i+1], route[i]))) distance += distances.get((route[-1], route[0]), distances.get((route[0], route[-1]))) return distance # Generate all permutations of cities routes = list(itertools.permutations(cities)) # Find the shortest route shortest_route = min(routes, key=calculate_route_distance) shortest_distance = calculate_route_distance(shortest_route) print(f"The shortest route is {shortest_route} with a distance of {shortest_distance}.")
Dynamic Programming
Dynamic programming (DP) offers a more efficient solution compared to brute force by solving overlapping subproblems. One popular DP approach for TSP is the Held-Karp algorithm, which has a time complexity of O(n^2 * 2^n).
def held_karp(distances): n = len(distances) C = {} # Initialize distances for subsets of size 1 for k in range(1, n): C[(1 << k, k)] = distances[0][k] # Compute shortest paths for subsets of size > 1 for subset_size in range(2, n): for subset in itertools.combinations(range(1, n), subset_size): bits = 0 for bit in subset: bits |= 1 << bit for k in subset: prev_bits = bits & ~(1 << k) result = [] for m in subset: if m == k: continue result.append(C[(prev_bits, m)] + distances[m][k]) C[(bits, k)] = min(result) # Find the shortest path to return to the start bits = (2 ** n - 1) - 1 result = [] for k in range(1, n): result.append(C[(bits, k)] + distances[k][0]) return min(result) # Example distance matrix distances = [ [0, 10, 15, 20], [10, 0, 35, 25], [15, 35, 0, 30], [20, 25, 30, 0] ] print(f"The shortest distance using Held-Karp is {held_karp(distances)}.")
Approximation Algorithms
When exact solutions are infeasible, approximation algorithms can provide near-optimal solutions in a reasonable time. The Christofides algorithm, for example, guarantees a solution within 1.5 times the optimal route length.
Genetic Algorithms
Inspired by natural selection, genetic algorithms generate a population of possible solutions and iteratively evolve them. This approach is suitable for very large instances where other methods fall short.
import random def create_route(cities): route = random.sample(cities, len(cities)) return route def calculate_fitness(route, distances): return 1 / calculate_route_distance(route) def evolve_population(population, distances): population.sort(key=lambda route: calculate_fitness(route, distances), reverse=True) new_population = population[:10] for _ in range(len(population) - 10): parent1 = random.choice(population[:10]) parent2 = random.choice(population[:10]) child = crossover(parent1, parent2) mutate(child) new_population.append(child) return new_population def crossover(parent1, parent2): child = parent1[:len(parent1)//2] + [city for city in parent2 if city not in parent1[:len(parent1)//2]] return child def mutate(route): for swapped in range(len(route)): if random.random() < 0.1: swap_with = int(random.random() * len(route)) route[swapped], route[swap_with] = route[swap_with], route[swapped] # Example cities and distances cities = ["A", "B", "C", "D"] distances = { ("A", "B"): 10, ("A", "C"): 15, ("A", "D"): 20, ("B", "C"): 35, ("B", "D"): 25, ("C", "D"): 30 } population = [create_route(cities) for _ in range(100)] for _ in range(1000): population = evolve_population(population, distances) best_route = min(population, key=calculate_route_distance) best_distance = calculate_route_distance(best_route) print(f"The best route found by the genetic algorithm is {best_route} with a distance of {best_distance}.")
Conclusion
The Traveling Salesman Problem is a fascinating and complex challenge that highlights the beauty and difficulty of optimization in computer science. Whether you're using brute force, dynamic programming, or genetic algorithms, each approach offers unique insights and trade-offs. Understanding these methods not only solves TSP but also opens the door to tackling a wide array of optimization problems in the real world.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
Why is TSP considered a difficult problem to solve?
TSP is considered difficult because it is an NP-hard problem, meaning that there is no known polynomial-time algorithm to solve it for large numbers of cities. As the number of cities increases, the number of possible routes grows factorially, making it computationally expensive to find the optimal solution.
What are some real-world applications of TSP?
TSP has numerous real-world applications in logistics (optimizing delivery routes), manufacturing (minimizing wiring in circuit board design), and even genetics (DNA sequencing). Efficiently solving TSP can lead to significant cost savings and operational efficiencies in these fields.
How does the Held-Karp algorithm work?
The Held-Karp algorithm uses dynamic programming to solve TSP. It breaks down the problem into smaller subproblems, solves each subproblem just once, and stores the solutions. By combining these solutions, it finds the shortest possible route. This method is more efficient than brute force but still exponential in time complexity.
Are approximation algorithms always accurate?
Approximation algorithms do not always provide the optimal solution, but they can yield near-optimal solutions in a reasonable time frame. For example, the Christofides algorithm guarantees a solution within 1.5 times the optimal route length. These algorithms are useful when an exact solution is impractical due to time or computational constraints.
What makes genetic algorithms suitable for solving TSP?
Genetic algorithms are suitable for TSP because they can handle large, complex instances where traditional methods fall short. They use principles of natural selection to evolve a population of solutions, gradually improving their quality over generations. This approach is flexible and can adapt to various constraints and requirements.