Git Branching
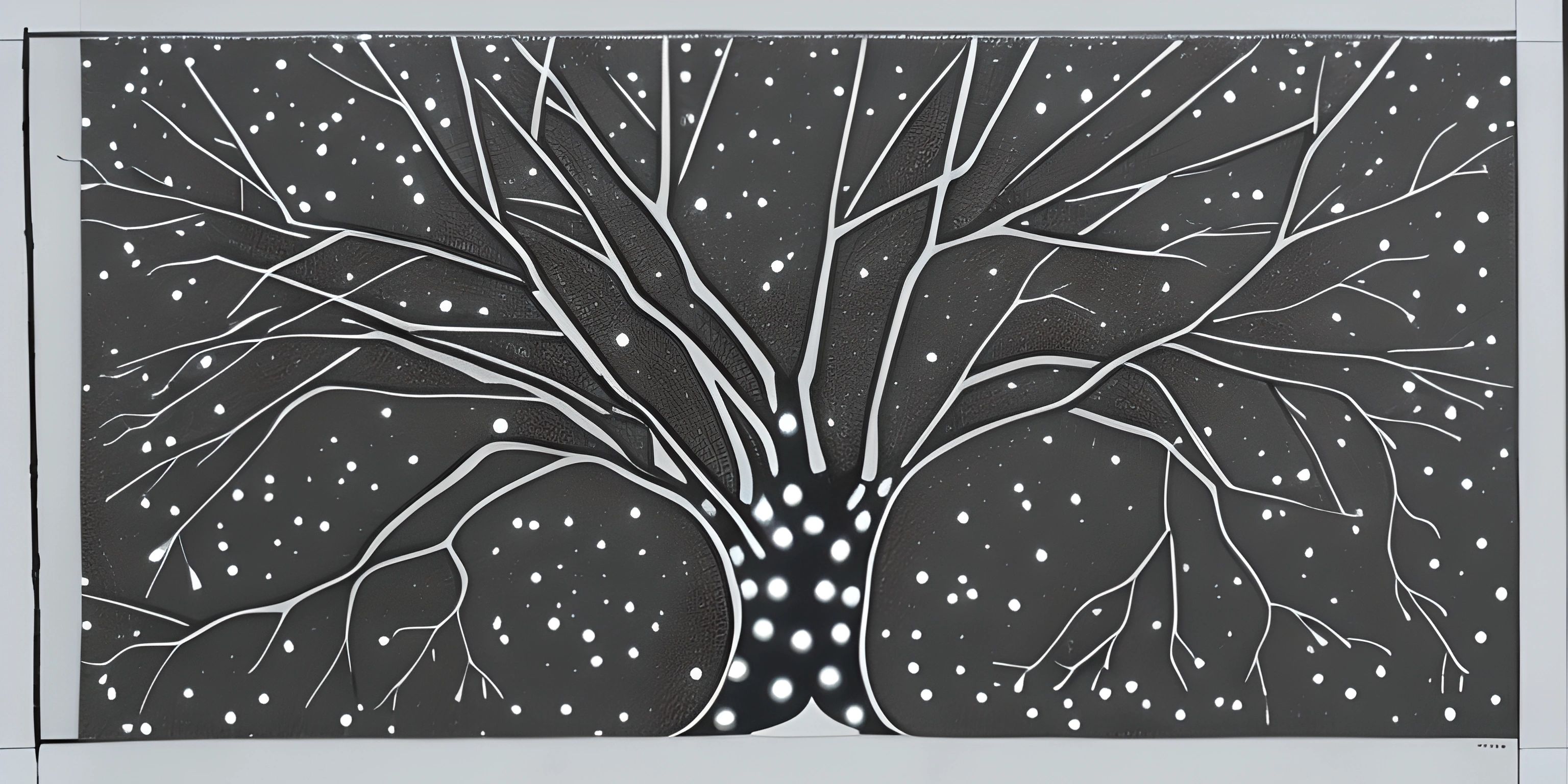
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When navigating the winding roads of code development, Git branches act like helpful road signs, guiding us through different features and bug fixes. In this world of version control, branches are essential for organizing and managing code changes.
Branching Out
Imagine you're working on a project with other developers, and you're all making changes to the same code. Without Git, this can quickly become chaotic. That's where branching comes in handy.
A Git branch is a unique, independent line of development within a repository. Think of it like a parallel universe where you can work on a new feature or fix a bug without disrupting the main branch (typically called master
or main
). When you're happy with your work, you can merge the changes back into the main branch, seamlessly integrating your code with the rest of the project.
Creating a Branch
To create a new branch, use the git checkout
command followed by the -b
flag and the name of your new branch:
git checkout -b my-new-branch
This command creates a new branch called my-new-branch
and switches to it right away. You're now free to make changes to the code without affecting the main branch. When you're ready to share your work, the next step is merging.
Merging Branches
Merging is the process of combining the changes from one branch with another. Before you can merge, you'll want to ensure your local branch is up-to-date with the remote repository by using git pull
. Once you've pulled the latest changes, you can switch back to the main branch and merge your feature branch:
git checkout main git merge my-new-branch
This merges the changes from my-new-branch
into main
. If there are no conflicts, your feature branch's changes are now safely integrated into the main branch.
Handling Conflicts
Sometimes, Git can't automatically merge branches and you'll encounter conflicts. Don't worry; resolving conflicts is a rite of passage for every programmer. Git will mark the problematic areas in the code, and it's up to you to manually resolve the differences. Once you've made the necessary adjustments, stage and commit the changes:
git add . git commit -m "Resolved conflicts and completed merge"
With the conflicts resolved, your branches are now successfully merged!
Branching Strategies
Developing a good branching strategy is crucial for maintaining a healthy and organized codebase. One popular approach is the Gitflow Workflow, which uses multiple branches to manage different aspects of development, such as features, releases, and hotfixes.
By incorporating Git branching into your development process, you'll create a more manageable and collaborative coding environment. Just remember, branches are your friends – they keep your code organized and your developers sane.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Getting Things Moving (psst, it's free!).
FAQ
What is Git branching and why is it useful?
Git branching is a powerful feature of Git that allows developers to create separate branches or timelines of code. This enables them to work on multiple features or fixes simultaneously, without affecting the main branch. It's useful because it helps in managing and organizing code, making it easier to collaborate and maintain a clean, stable codebase.
How do I create a new Git branch?
To create a new Git branch, you can use the git checkout
command with the -b
option, followed by the name of the new branch. For example:
git checkout -b my-new-feature-branch
This command will create a new branch called "my-new-feature-branch" and switch to it immediately.
How can I switch between branches in Git?
To switch between branches, use the git checkout
command followed by the name of the branch you want to switch to. For instance:
git checkout my-other-feature-branch
This command will switch from your current branch to "my-other-feature-branch."
How do I merge changes from one branch into another?
To merge changes from one branch into another, first, switch to the branch you want to merge into using the git checkout
command. Then, use the git merge
command followed by the name of the branch you want to merge. For example:
git checkout main git merge my-feature-branch
This command will merge the changes from "my-feature-branch" into the "main" branch.
How can I delete a Git branch?
To delete a local Git branch, use the git branch
command with the -d
option (for a merged branch) or the -D
option (for an unmerged branch) followed by the name of the branch you want to delete. For example:
git branch -d my-feature-branch
This command will delete the "my-feature-branch" if it's already merged. If it's not merged, use the -D
option instead:
git branch -D my-feature-branch