Introduction to Git
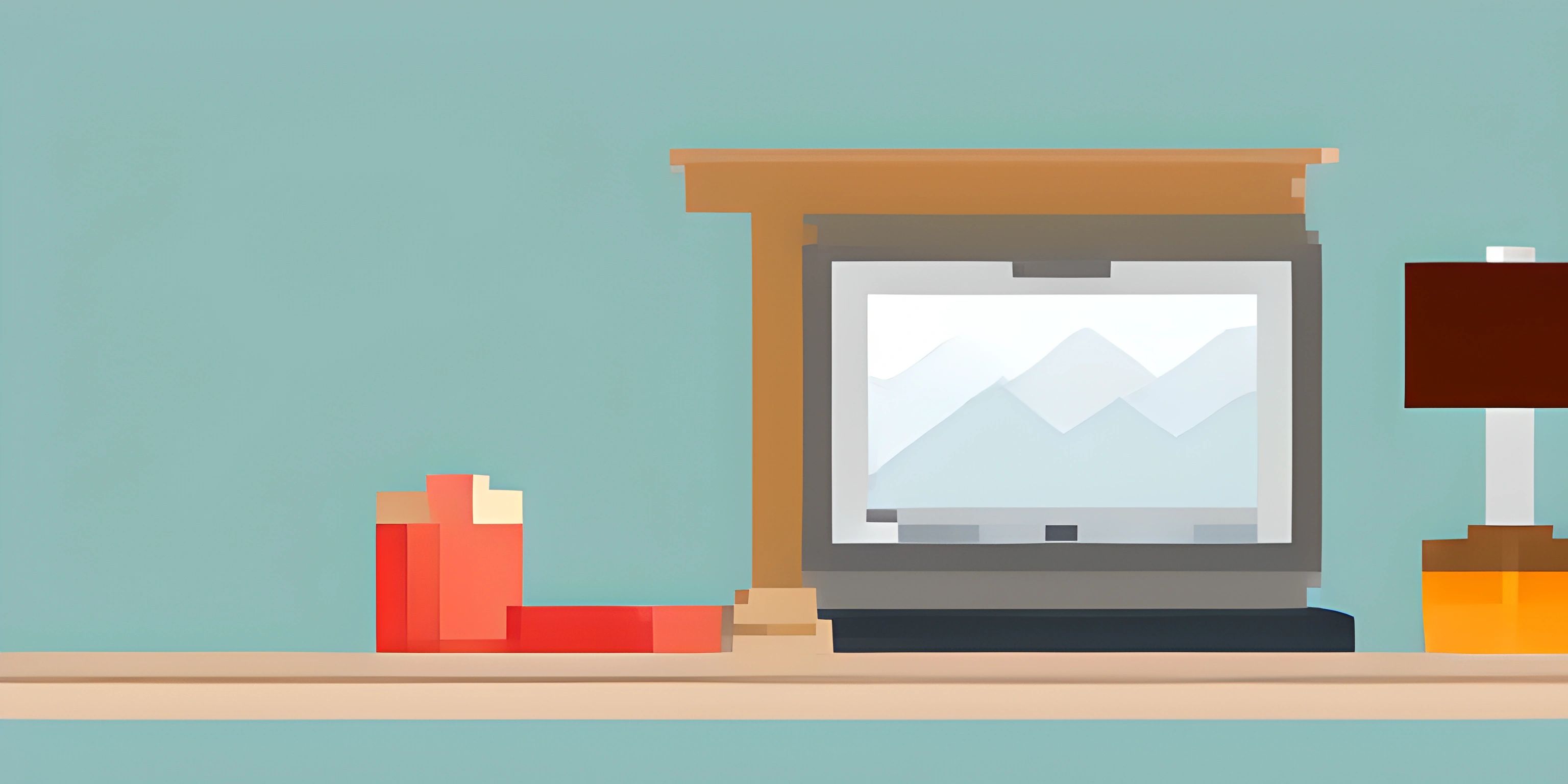
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Git is a powerful, modern version control system that developers use to keep track of their code. It makes collaboration easy, prevents data loss, and helps manage code changes efficiently. In this article, we'll learn the basics of Git, its purpose, and some essential Git commands.
Why Use Git?
Imagine you're working on a project with a team of developers. You're all making changes to the code, and you need a way to manage those changes, review them, and make sure nothing breaks. That's where Git comes in!
Git helps you:
- Keep track of changes made to the code.
- Collaborate with other developers.
- Roll back to a previous version of the code if something goes wrong.
- Create different branches of the code to work on new features or bug fixes.
In short, Git makes life easier for developers.
Git Repositories
A Git repository is a directory that contains your project's code and its history. When you initialize a Git repository, Git stores information about all the changes you make to your project in a hidden folder called .git
. This folder stores the entire history of your project.
To create a new Git repository, navigate to your project's root folder using the command line and run the following command:
git init
This command initializes a new Git repository in your project folder.
Basic Git Commands
Let's dive into some basic Git commands that you'll likely use often.
git status
git status
shows the current state of your working directory. It tells you which files have been modified, deleted, or added, as well as which files are staged (ready to be committed) and which are not.
git status
git add
To stage changes to your code for a commit, you'll use the git add
command. You can stage all changes in a directory, or stage only specific files.
git add . # Stage all changes in the directory git add file1.txt file2.txt # Stage specific files
git commit
After staging your changes, you'll want to commit them. A commit is like taking a snapshot of your current project state. Use the git commit
command along with the -m
flag to add a commit message describing the changes made.
git commit -m "My first commit"
git log
To view the commit history of your repository, use the git log
command. This will display a list of all the commits made to the repository, along with their unique identifiers and commit messages.
git log
git diff
The git diff
command shows the differences between your working directory and the latest commit. This is useful for reviewing changes before staging or committing them.
git diff
git pull
When working with a remote repository (like on GitHub), you'll need to synchronize your local repository with the remote one. The git pull
command fetches the latest changes from the remote repository and merges them into your local repository.
git pull
git push
After committing changes to your local repository, you'll want to push those changes to the remote repository so that others can access them. Use the git push
command to do this.
git push
These are just the basics of Git, but they'll get you started on managing your code effectively. As you become more comfortable with Git, you'll discover additional commands and techniques to help you manage your projects more efficiently. Until then, happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Welcome to Cratecode! (psst, it's free!).
FAQ
What is Git and why is it essential in software development?
Git is a widely-used distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It allows developers to track changes in their code, collaborate with others, and revert to earlier versions when needed. Git is essential because it helps maintain the integrity of code, fosters collaboration, and simplifies the development process.
How do I install Git on my computer?
To install Git, follow these steps based on your operating system:
- Windows: Download the installer from the official Git website (https://git-scm.com/download/win) and run it.
- macOS: Install Git using Homebrew by running
brew install git
in the terminal. If you don't have Homebrew, you can download the installer from the official Git website (https://git-scm.com/download/mac). - Linux: Use the package manager for your distribution to install Git. For example, on Ubuntu or Debian-based systems, run
sudo apt-get install git
in the terminal.
How do I create a new Git repository for my project?
To create a new Git repository for your project, follow these steps:
- Navigate to your project folder using the terminal or command prompt.
- Run the command
git init
. This will initialize a new Git repository in your project folder.
What are some essential Git commands to get started?
Here are some essential Git commands to get started:
git init
: Initialize a new Git repository.git add .
: Add all changes in the working directory to the staging area.git commit -m "Your commit message"
: Commit the staged changes with a descriptive message.git status
: Check the status of your working directory, including changes and staged files.git log
: Show the commit history.
How can I revert to a previous commit in Git?
To revert to a previous commit in Git, use the git checkout
command followed by the commit hash. For example:
git checkout 3a7b61e
This will switch your working directory to the specified commit. Be careful when using this command, as any uncommitted changes in your working directory might be lost.