Haskell Functional Programming
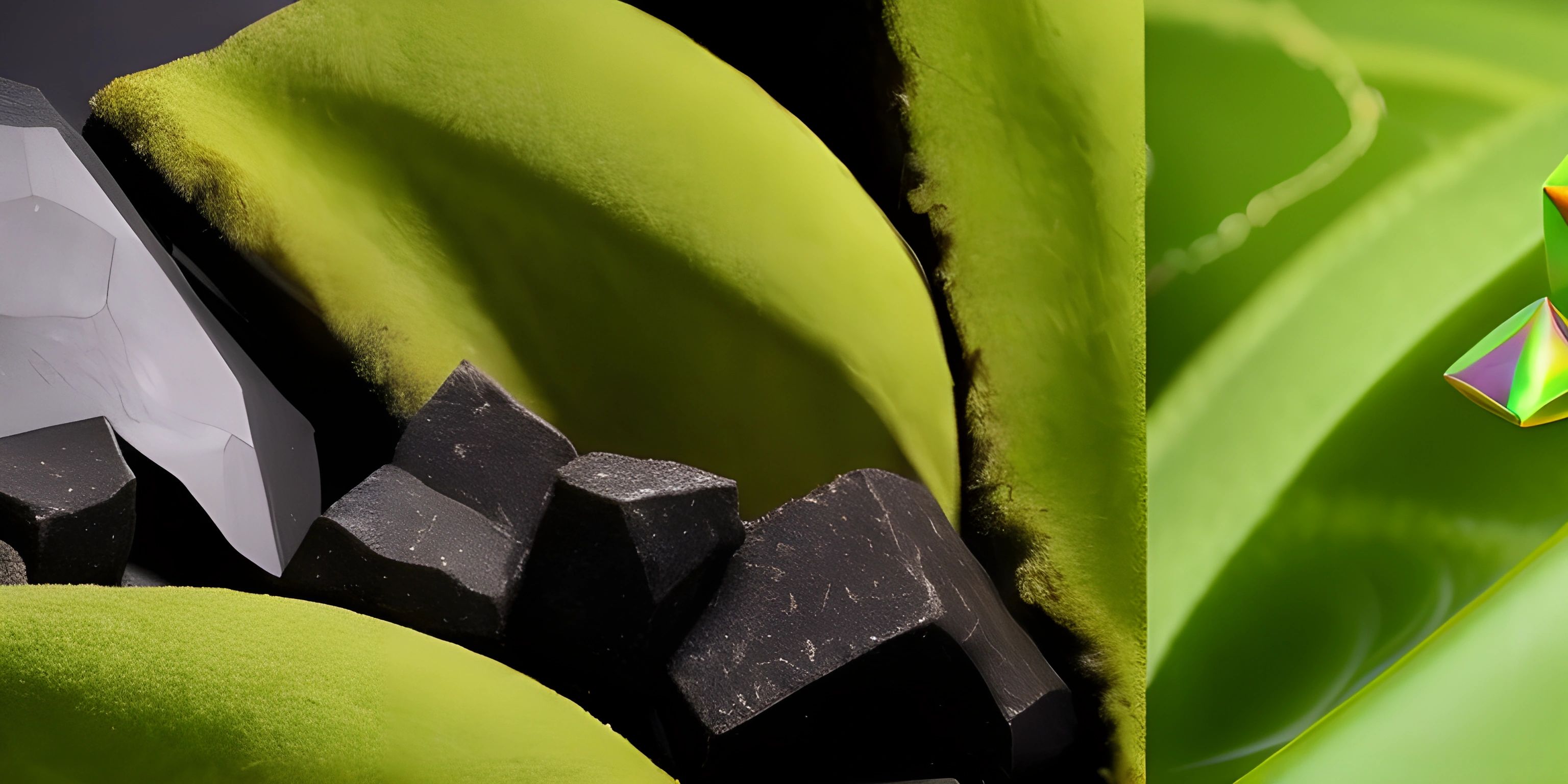
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the fascinating world of functional programming with Haskell, a language that is built from the ground up with functional concepts in mind. Functional programming (FP) is a programming paradigm where functions are the primary building blocks, and Haskell is a prime example of a language that embraces FP. In this article, we'll explore the main functional concepts in Haskell, including pure functions, recursion, lambda expressions, higher-order functions, and lazy evaluation.
Pure Functions
A pure function is a function that always returns the same output for the same input, and it does not have any side effects, like modifying global variables or performing I/O operations. In Haskell, functions are pure by default. For instance, let's look at the following example:
double :: Int -> Int double x = x * 2
The double
function takes an Int
as an input and returns another Int
. This function is pure because it always returns the same output for the same input and has no side effects. Purity is a core concept in Haskell and functional programming, as it leads to more predictable and easier-to-understand code.
Recursion
Since Haskell is a functional language, it heavily relies on recursion as a way to solve problems. Recursion is a technique where a function calls itself, usually with a reduced problem size, to solve the initial problem. Here's an example of how to calculate the factorial of a number using recursion in Haskell:
factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1)
This function calculates the factorial of a number n
by multiplying it with the factorial of n - 1
, until n
reaches 0.
Lambda Expressions
Lambda expressions, also known as anonymous functions, are a way to create functions without giving them a name. They are often used for short, simple tasks like passing a function as an argument to a higher-order function. In Haskell, you can create a lambda expression using the following syntax:
\x -> x * 2
This lambda expression takes an input x
and returns x * 2
. You can use this lambda expression in various situations, such as mapping it over a list:
map (\x -> x * 2) [1, 2, 3, 4] -- Output: [2, 4, 6, 8]
Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return them as results. They are a powerful tool in functional programming, as they allow us to abstract away common patterns and create more reusable code. Let's look at an example of a higher-order function, map
:
map :: (a -> b) -> [a] -> [b] map _ [] = [] map f (x:xs) = f x : map f xs
The map
function takes a function f
and a list [a]
, applies the function f
to each element of the list, and returns a new list [b]
.
Lazy Evaluation
Haskell uses lazy evaluation, which means that expressions are only evaluated when their values are needed. This can lead to increased performance, as computations are only performed when necessary. Lazy evaluation also allows for the creation of infinite data structures, such as the list of all natural numbers:
naturals :: [Integer] naturals = [0..]
Even though this list is infinite, Haskell only evaluates the elements that are needed, making it possible to work with such data structures.
FAQ
What is functional programming?
Functional programming is a programming paradigm that emphasizes the use of functions as the primary building blocks of the code. It focuses on concepts like pure functions, recursion, lambda expressions, higher-order functions, and lazy evaluation.
What makes Haskell a functional programming language?
Haskell is a functional programming language because it has been designed from the ground up with functional concepts in mind. Functions are the primary building blocks of Haskell code, and it promotes the use of pure functions, recursion, lambda expressions, higher-order functions, and lazy evaluation.
How does lazy evaluation work in Haskell?
Lazy evaluation in Haskell means that expressions are only evaluated when their values are needed. This can lead to increased performance, as computations are only performed when necessary. It also allows for the creation of infinite data structures, which are only evaluated as far as needed.