A Detailed Explanation of the Intel x86 Instruction Set
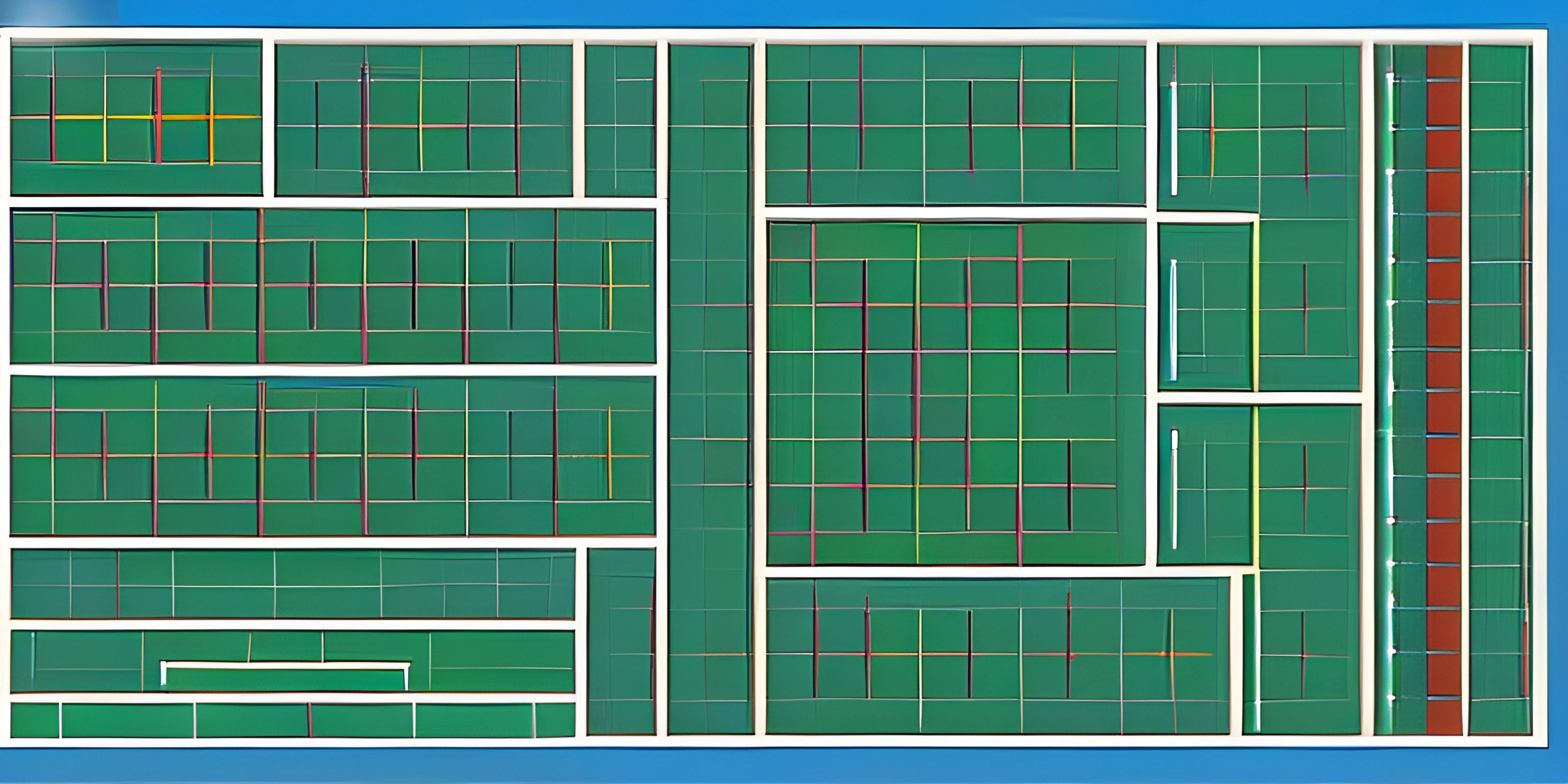
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Understanding the Intel x86 instruction set is like having the secret map to a vast treasure. It's the key to unlocking the power of countless modern computing systems. If you've ever wondered how your computer's brain performs its magic, you're about to enter a fascinating world of binary spells and logic wizardry. Buckle up, because this journey through the Intel x86 instruction set is like hopping on a roller coaster through the inner workings of your CPU!
What is the Intel x86 Instruction Set?
At its core, the Intel x86 instruction set is a collection of commands that a CPU can execute. Think of it as the native language your computer speaks, composed of precise and concise instructions that tell the processor what to do. These instructions can be as simple as adding two numbers or as complex as managing memory.
The Architecture
The x86 architecture, developed by Intel, has been around since the 1970s and has evolved significantly over the decades. It's like the Swiss army knife of computer architectures—versatile, reliable, and packed with functionality. The architecture includes a variety of registers, instruction formats, addressing modes, and more.
Registers
Registers are like the CPU's scratchpad. They are small, fast storage locations used to hold data temporarily. The most common registers in the x86 architecture include:
- EAX: The accumulator register, used for arithmetic operations.
- EBX: The base register, often used to hold base addresses.
- ECX: The count register, used for loop counters.
- EDX: The data register, used for I/O operations.
- ESI and EDI: Source and destination index registers, used for string operations.
- ESP and EBP: Stack pointer and base pointer, used for stack operations.
Instruction Formats
Each instruction in the x86 instruction set is composed of an opcode (operation code) and operands (data). The opcode specifies the operation to be performed, while the operands provide the data on which the operation acts. For example, the instruction ADD EAX, 1
tells the CPU to add the value 1 to the contents of the EAX register.
Addressing Modes
Addressing modes define how the CPU should interpret the operands. The x86 architecture supports several addressing modes, including:
- Immediate: The operand is a constant value. For example,
MOV EAX, 5
moves the value 5 into the EAX register. - Register: The operand is a register. For example,
MOV EAX, EBX
copies the contents of the EBX register into the EAX register. - Direct: The operand is a memory address. For example,
MOV EAX, [0x00400000]
moves the value at memory address 0x00400000 into the EAX register. - Indirect: The operand is a memory address contained in a register. For example,
MOV EAX, [EBX]
moves the value at the address stored in the EBX register into the EAX register.
Common Instructions
Now, let's dive into some of the most commonly used instructions in the x86 instruction set. These instructions form the building blocks of assembly language programs.
Data Movement Instructions
MOV
: Copies data from one location to another. For example,MOV EAX, EBX
copies the contents of EBX into EAX.PUSH
andPOP
: Add and remove data from the stack. For example,PUSH EAX
places the contents of EAX onto the stack, andPOP EAX
removes the top value from the stack into EAX.
Arithmetic Instructions
ADD
andSUB
: Perform addition and subtraction. For example,ADD EAX, 2
adds 2 to the contents of EAX, whileSUB EAX, 1
subtracts 1 from EAX.MUL
andDIV
: Perform multiplication and division. For example,MUL EBX
multiplies the contents of EAX by EBX, storing the result in EAX and EDX.
Logic Instructions
AND
,OR
, andXOR
: Perform bitwise logical operations. For example,AND EAX, EBX
performs a bitwise AND operation between EAX and EBX, storing the result in EAX.NOT
andNEG
: Perform bitwise NOT and arithmetic negation. For example,NOT EAX
inverts all the bits in EAX.
Control Flow Instructions
JMP
: Unconditionally jumps to a specified address. For example,JMP 0x00400000
jumps to the instruction at address 0x00400000.JE
,JNE
,JG
,JL
: Conditional jumps based on the comparison of values. For example,JE 0x00400000
jumps to the address if the zero flag is set, indicating equality.CALL
andRET
: Call and return from procedures. For example,CALL 0x00400000
jumps to the procedure at the specified address, whileRET
returns from the procedure.
Example Program
Let's put it all together with a simple example program that adds two numbers and prints the result. We'll use the NASM assembler for this example.
section .data ; Declare data segment num1 db 5 num2 db 10 result db 0 section .text global _start _start: ; Load numbers into registers mov al, [num1] mov bl, [num2] ; Add numbers add al, bl ; Store result mov [result], al ; Exit program mov eax, 1 ; syscall number for exit xor ebx, ebx ; return code 0 int 0x80 ; call kernel
This program:
- Declares three variables:
num1
,num2
, andresult
. - Loads the values of
num1
andnum2
into theAL
andBL
registers. - Adds the values in
AL
andBL
, storing the result inAL
. - Moves the result from
AL
to theresult
variable. - Exits the program by calling the
exit
syscall.
Advanced Topics
For those who have mastered the basics, there's a whole universe of advanced topics to explore:
SIMD Instructions
Single Instruction, Multiple Data (SIMD) instructions allow the CPU to perform the same operation on multiple data points simultaneously, significantly speeding up tasks like multimedia processing and scientific computing. The SSE (Streaming SIMD Extensions) and AVX (Advanced Vector Extensions) are examples of SIMD instruction sets in the x86 architecture.
Protected Mode
Protected mode is an operational mode of x86-compatible CPUs that allows for advanced features like virtual memory, paging, and safe multi-tasking. It's like giving your CPU superpowers to manage complex applications and operating systems efficiently.
Interrupts and Exceptions
Interrupts are signals that alert the CPU to stop its current activities and execute a specific piece of code. They are essential for handling events like I/O operations, timer ticks, and system calls. Exceptions are similar but are triggered by error conditions, such as division by zero or invalid memory access.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is the x86 instruction set?
The x86 instruction set is a collection of commands that a CPU can execute. It includes instructions for data movement, arithmetic, logic, and control flow, among others. It's the native language of x86-compatible processors, allowing them to perform various operations.
What are registers in the x86 architecture?
Registers are small, fast storage locations within the CPU used to hold data temporarily. Common registers in the x86 architecture include EAX, EBX, ECX, EDX, ESI, EDI, ESP, and EBP. They play a crucial role in executing instructions efficiently.
What are addressing modes?
Addressing modes define how the CPU should interpret the operands of an instruction. Common addressing modes in the x86 architecture include immediate, register, direct, and indirect. Each mode specifies a different way to access data for operations.
What is the purpose of the MOV instruction?
The MOV instruction is used to copy data from one location to another. It can move data between registers, memory, and immediate values. For example, MOV EAX, EBX
copies the contents of the EBX register into the EAX register.
What are SIMD instructions?
SIMD (Single Instruction, Multiple Data) instructions allow the CPU to perform the same operation on multiple data points simultaneously. They are used to speed up tasks like multimedia processing and scientific computing. Examples include SSE (Streaming SIMD Extensions) and AVX (Advanced Vector Extensions).