Creating and Using Objects in Java
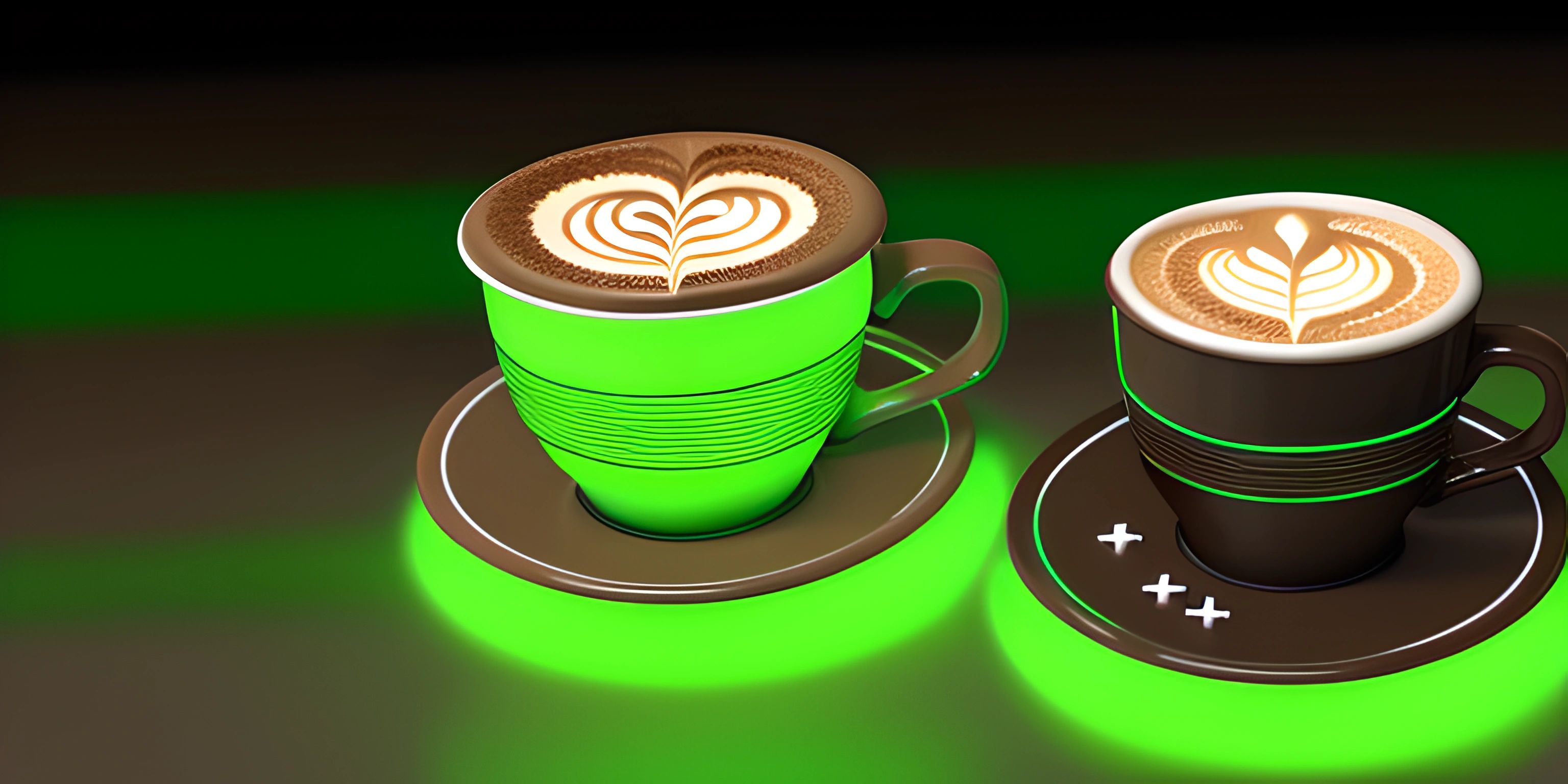
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Objects are the backbone of Java, an object-oriented programming language. They are the building blocks that allow you to create complex software systems. In this article, we'll dive into creating and using objects in Java through classes, constructors, and instances.
Classes
A class in Java is a blueprint for creating objects. It defines the structure and behavior of the objects that will be created. Classes contain properties (variables) and methods (functions) that define the state and behavior of the objects. Let's start with a basic class, Dog
:
public class Dog { // Properties String name; int age; // Methods void bark() { System.out.println("Woof!"); } }
The Dog
class has two properties, name
and age
, and one method, bark()
. This class serves as a blueprint for creating Dog
objects with specific names and ages that can also bark.
Constructors
Constructors are special methods in a class that are called when an object is created. They are used to initialize the object's properties. In Java, a constructor has the same name as the class and has no return type. If you don't provide a constructor, Java automatically creates a default constructor for you. Here's an example of a custom constructor for the Dog
class:
public class Dog { // Properties String name; int age; // Constructor public Dog(String dogName, int dogAge) { name = dogName; age = dogAge; } // Methods void bark() { System.out.println("Woof!"); } }
In this case, we've provided a constructor that takes two parameters, dogName
and dogAge
. When we create a new Dog
object, we'll pass the desired name and age to the constructor, which will initialize the object's properties.
Instances
An instance is an individual object created from a class. To create an instance of a class, we use the new
keyword followed by the class name and constructor parameters (if any). Here's an example of creating a Dog
object using the Dog
class:
public class Main { public static void main(String[] args) { // Create a Dog object Dog myDog = new Dog("Buddy", 3); // Access the object's properties System.out.println("My dog's name is: " + myDog.name); System.out.println("My dog is " + myDog.age + " years old."); // Call the object's methods myDog.bark(); } }
This code creates a Dog
object named myDog
with the name "Buddy" and age 3. We can then access the object's properties and methods using the dot notation, like myDog.name
and myDog.bark()
.
In conclusion, objects are an essential aspect of Java and object-oriented programming. By understanding classes, constructors, and instances, you're well on your way to mastering Java programming. Continue exploring Java concepts like inheritance and interfaces to build more complex and powerful Java applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is an object in Java, and why is it important?
An object in Java is an instance of a class, which encapsulates data and methods that operate on the data. Objects are important because they're the building blocks of object-oriented programming (OOP). OOP allows you to model real-world entities as software components, making it easier to design and maintain complex applications.
How do I create an object in Java?
To create an object in Java, you need to define a class first. A class is like a blueprint for creating objects. Once you've defined a class, you can create an object (an instance of the class) using the new
keyword and calling the constructor method. Here's an example:
class Dog { String breed; int age; Dog(String breed, int age) { this.breed = breed; this.age = age; } } public class Main { public static void main(String[] args) { Dog myDog = new Dog("Labrador", 3); } }
How do I access and modify data within a Java object?
You can access and modify an object's data by using its instance variables and methods. To access or modify an instance variable, use the dot notation with the object name, followed by the variable name:
myDog.breed = "Golden Retriever"; String dogBreed = myDog.breed;
To call an instance method, use the dot notation with the object name, followed by the method name and parentheses:
myDog.bark();
What is a constructor in Java, and how does it work?
A constructor is a special method in a Java class that's called when a new object is created. It has the same name as the class and doesn't have a return type. Constructors are used to initialize the object's instance variables and perform any setup required for the object. You can define a constructor with parameters to customize the object's initialization. If you don't define a constructor, Java provides a default constructor with no parameters.
How can I create multiple objects from the same class?
You can create multiple objects from the same class by repeatedly using the new
keyword and calling the constructor method. Each object will be a separate instance with its own set of instance variables:
Dog dog1 = new Dog("Labrador", 3); Dog dog2 = new Dog("Golden Retriever", 2); Dog dog3 = new Dog("Poodle", 5);