Overriding Methods in Java
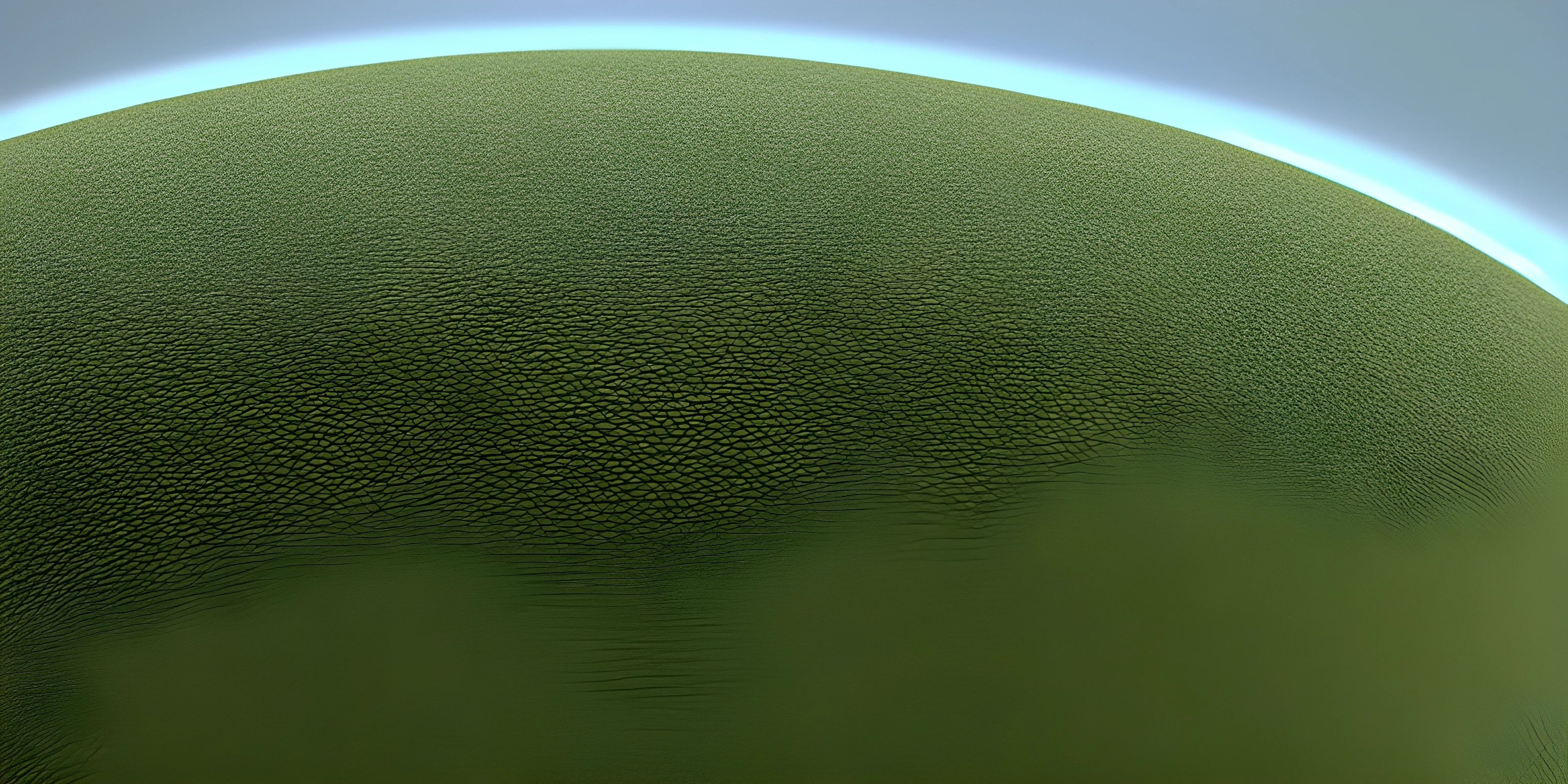
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Strap on your coding boots, we're going on a Java journey! We're exploring one of the core concepts of object-oriented programming in Java - method overriding. If you're new to this, fear not. Picture method overriding like a talent show, where everyone has the chance to showcase their own unique act, even if the stage (or method name) remains the same.
The Backdrop of Method Overriding
Method overriding is a feature of Java, letting a subclass provide a specific implementation of a method that's already provided by its parent class. It’s like the parent class says: “Here’s how we do things around here”, and the subclass says: “Well, actually, I have a better way to do that.” This is the crux of polymorphism, one of the four pillars of object-oriented programming.
class Parent { void sayHello() { System.out.println("Hello from Parent"); } } class Child extends Parent { void sayHello() { System.out.println("Hello from Child"); } } public class Main { public static void main(String[] args) { Parent p = new Child(); p.sayHello(); // Outputs: Hello from Child } }
In this code, the sayHello()
method in the Child
class overrides the sayHello()
method in the Parent
class.
The Nuts and Bolts of Method Overriding
To override a method, the method in the child class must have the same name, parameter list, and return type as the method in the parent class. The @Override
annotation is not required, but it's good practice to include it because it makes your code easier to understand, and the compiler can warn you if the method is not actually being overridden.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is method overriding in Java?
Method overriding in Java is a feature that allows a subclass to provide a specific implementation of a method that's already provided by its parent class. It's a way for the subclass to change how a certain method works, offering a different behavior than the one defined in the parent class.
When should I use method overriding?
You should use method overriding when you want a subclass to provide a different implementation of a method that's already provided by the parent class. It's typically used when the method from the parent class doesn't quite fit the subclass, and the subclass needs to tweak it to suit its needs.
Is the @Override annotation mandatory for method overriding?
No, the @Override
annotation is not mandatory for method overriding. However, it's highly recommended to use it because it improves code readability and helps detect errors at compile-time if the method is not correctly overriding a method from the parent class.