Java Polymorphism
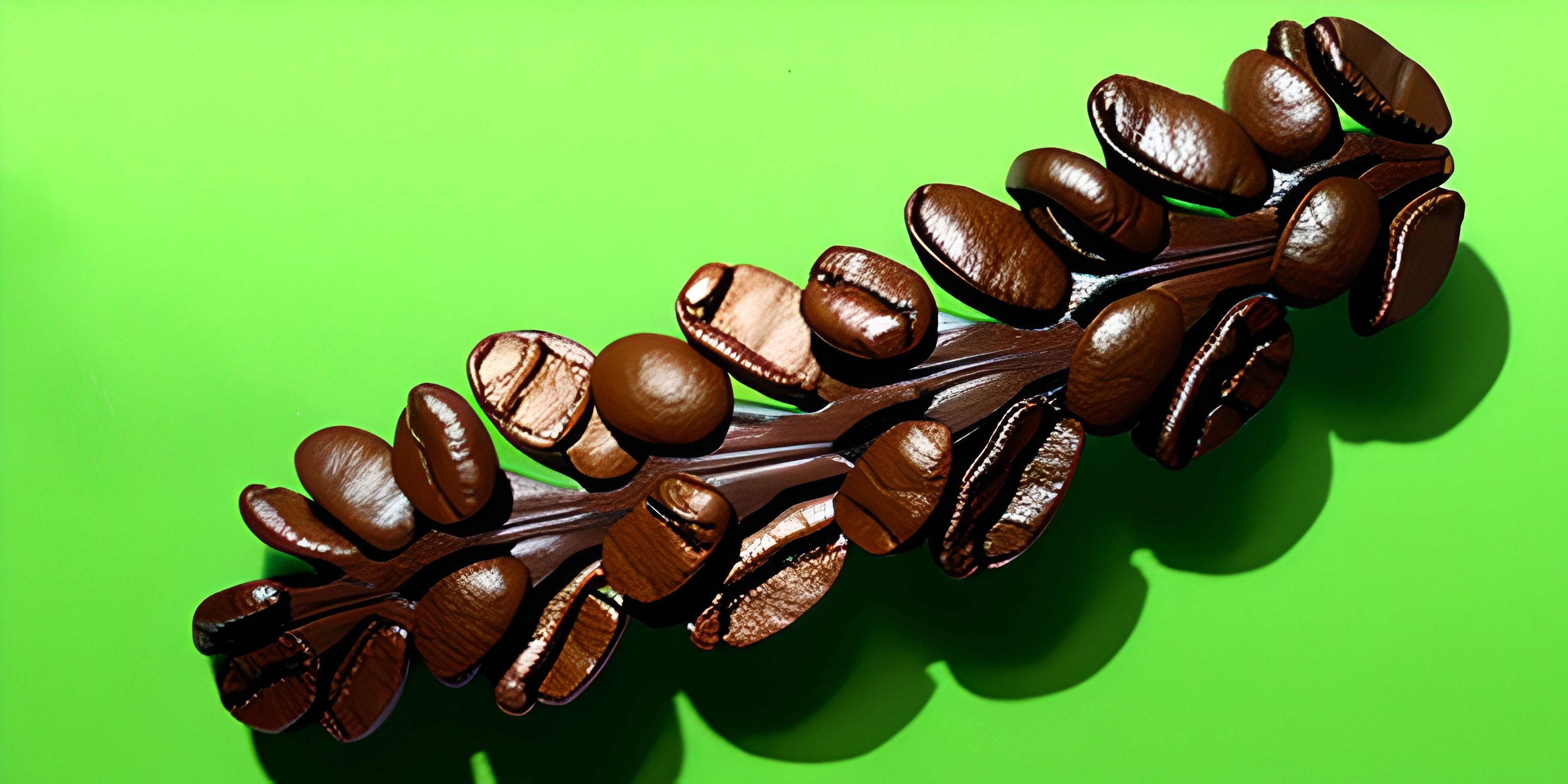
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Polymorphism is a fundamental concept in object-oriented programming (OOP), and Java is no exception. It allows objects of different classes to be treated as objects of a common superclass or interface. In this sense, polymorphism enables us to write more flexible and reusable code.
Inheritance and Polymorphism
In Java, polymorphism is closely related to inheritance. When a class inherits from another class, it can inherit its methods and properties, making it possible to reuse and extend code in a systematic way.
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Dog extends Animal { void makeSound() { System.out.println("The dog barks"); } } class Cat extends Animal { void makeSound() { System.out.println("The cat meows"); } }
In this example, the Dog
and Cat
classes both extend the Animal
class, inheriting its makeSound()
method. However, they override this method to provide their own implementation. This is an example of polymorphism in action.
Method Overriding
Method overriding is a way to provide a new implementation for a method inherited from a superclass. When a subclass overrides a method, the original method in the superclass is replaced by the new implementation in the subclass.
Animal myAnimal = new Dog(); myAnimal.makeSound(); // The dog barks
In this example, we create a new Dog
object and assign it to a variable of type Animal
. When we call the makeSound()
method on this object, Java automatically calls the appropriate method implementation for the Dog
class, demonstrating polymorphism.
Interfaces and Polymorphism
Java also supports polymorphism through interfaces. An interface is a collection of abstract methods that any class implementing the interface must provide its own implementation for.
interface Animal { void makeSound(); } class Dog implements Animal { public void makeSound() { System.out.println("The dog barks"); } } class Cat implements Animal { public void makeSound() { System.out.println("The cat meows"); } }
In this example, we define an Animal
interface with a makeSound()
method. The Dog
and Cat
classes both implement this interface, providing their own implementations for the makeSound()
method. As with inheritance, this demonstrates polymorphism in action.
Benefits of Polymorphism
Polymorphism provides several benefits, such as:
- Code reusability: By allowing objects of different classes to be treated as objects of a common superclass or interface, we can write more generic and reusable code.
- Flexibility: Polymorphism enables us to easily extend our code by adding new classes or modifying existing ones without affecting other parts of the program.
- Abstraction: Polymorphism allows us to abstract the behavior of different classes behind a common interface, making it easier to reason about and manage our code.
By understanding and applying polymorphism in Java, you can write more versatile, maintainable, and efficient code that takes full advantage of the benefits of object-oriented programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).