Working with Arrays in Java
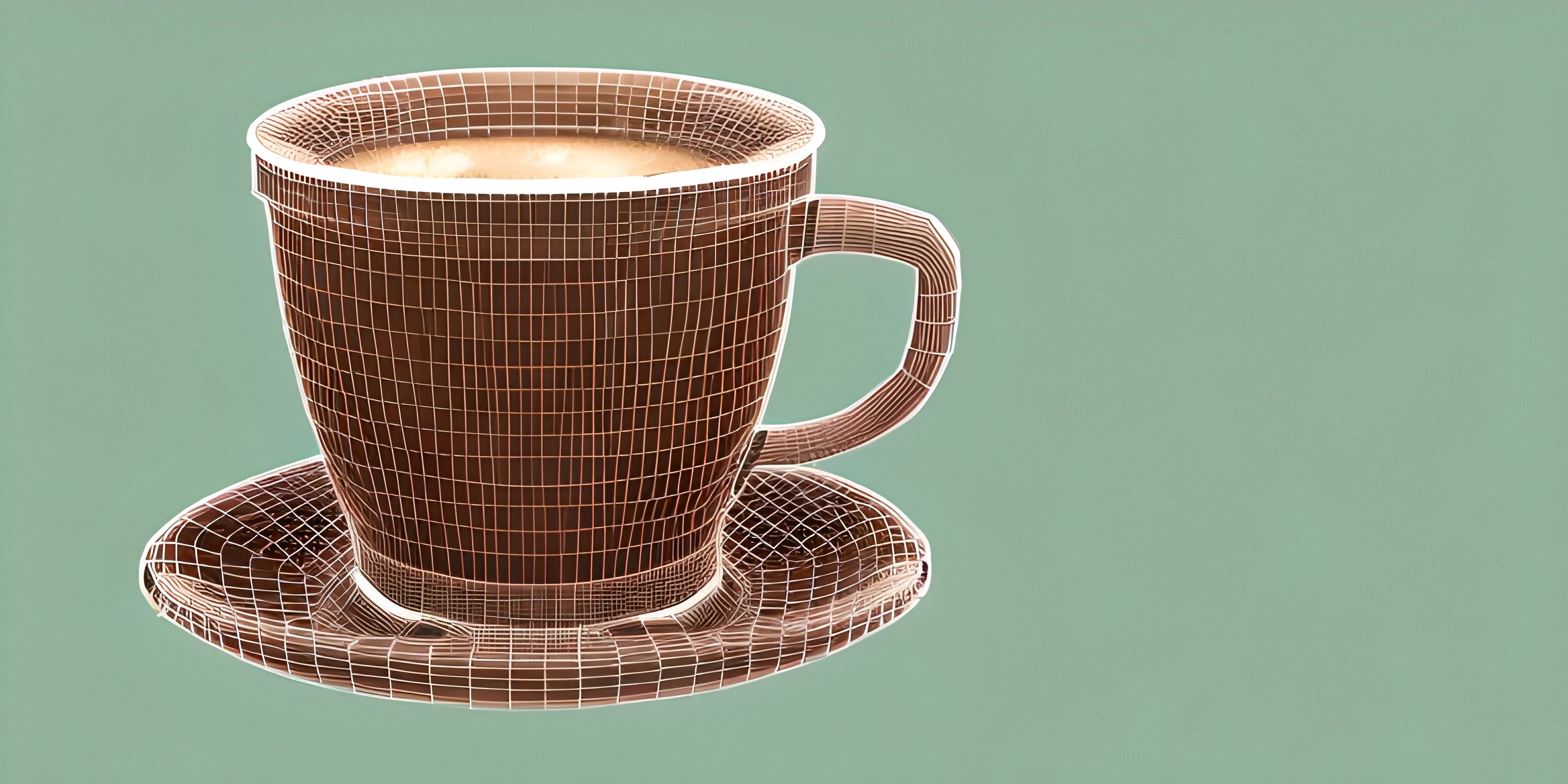
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Arrays are like a Swiss army knife in the world of programming – versatile, practical, and essential. In Java, arrays are used to store multiple values of the same data type in a single variable. They help organize, access, and manipulate data efficiently.
Creating an Array
In Java, you can create an array by specifying its data type followed by square brackets, and then the name of the array. To allocate memory for the array, use the new
keyword followed by the data type, and the desired length of the array inside square brackets. Here's an example of creating an integer array:
int[] myNumbers = new int[5];
This creates an array named myNumbers
that can store up to 5 integer values. You can also initialize an array with values directly:
int[] myNumbers = {10, 20, 30, 40, 50};
Accessing Array Elements
Now that we know how to create an array, let's learn to access its elements. You can do this by specifying the index of the element you want to access inside square brackets. Remember, array indices in Java start at 0.
int[] myNumbers = {10, 20, 30, 40, 50}; int firstNumber = myNumbers[0]; // Accessing the first element (10) int thirdNumber = myNumbers[2]; // Accessing the third element (30)
Modifying Elements
To modify an array element, simply assign a new value to the desired index:
int[] myNumbers = {10, 20, 30, 40, 50}; myNumbers[1] = 25; // Changing the second element from 20 to 25
Our modified myNumbers
array now looks like this: {10, 25, 30, 40, 50}
.
Array Length
You can find the length of an array using the length
property:
int[] myNumbers = {10, 20, 30, 40, 50}; int arrayLength = myNumbers.length; // Returns 5
This is quite handy when you want to perform operations on the entire array.
Looping Through Arrays
One of the most common operations on arrays is iterating through their elements. You can use a for loop to achieve this:
int[] myNumbers = {10, 20, 30, 40, 50}; for (int i = 0; i < myNumbers.length; i++) { System.out.println("Element at index " + i + ": " + myNumbers[i]); }
Alternatively, you can use a for-each loop to iterate through the array without dealing with indices:
int[] myNumbers = {10, 20, 30, 40, 50}; for (int number : myNumbers) { System.out.println("Element: " + number); }
Multidimensional Arrays
Java supports multidimensional arrays as well. A multidimensional array is an array of arrays, where each element can hold another array. The most common type is a two-dimensional array, which is essentially a matrix:
int[][] matrix = new int[3][3]; // Creates a 3x3 matrix // Initializing the matrix with values int[][] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
You can access and modify elements in a multidimensional array using a combination of indices:
int element = matrix[1][2]; // Accessing the element at row 1 and column 2 (6) matrix[0][0] = 42; // Changing the value at row 0 and column 0 to 42
There you have it – a brief introduction to the world of arrays in Java! As you continue exploring and experimenting with arrays, you'll discover their true power and importance in programming. So keep calm and array on!
FAQ
What is an array in Java and what are its properties?
An array in Java is a collection of elements, usually of the same data type (e.g., int, float, String), stored in contiguous memory locations. Here are some properties of arrays in Java:
- They have a fixed size, which is determined at the time of creation.
- They can store elements of any data type, including objects or even other arrays (multidimensional arrays).
- Arrays are indexed, with the first element at index 0 and the last element at index (length - 1).
How do you declare, create, and initialize an array in Java?
To declare, create, and initialize an array in Java, follow these steps:
- Declare the array by specifying the data type, followed by square brackets, and then the array name (e.g.,
int[] myArray;
). - Create the array by using the
new
keyword, the data type, and specifying the size of the array in square brackets (e.g.,myArray = new int[5];
). - Initialize the array by assigning values to each index (e.g.,
myArray[0] = 1;
). You can also declare, create, and initialize an array in a single line like this:
int[] myArray = {1, 2, 3, 4, 5};
How do you access elements in a Java array?
To access elements in a Java array, use the array name followed by square brackets containing the index of the desired element. For example:
int[] myArray = {1, 2, 3, 4, 5}; int firstElement = myArray[0]; // Accesses the first element (1) int lastElement = myArray[myArray.length - 1]; // Accesses the last element (5)
What are some common operations performed on arrays in Java?
Some common operations performed on arrays in Java include:
- Iterating through an array using a for loop or for-each loop.
- Searching for an element in an array using linear search or binary search (if the array is sorted).
- Sorting an array using built-in methods like
Arrays.sort()
or by implementing sorting algorithms like Bubble sort or Quick sort. - Copying an array using
System.arraycopy()
orArrays.copyOf()
methods. - Comparing arrays using
Arrays.equals()
method to check if two arrays contain the same elements in the same order.
How can I work with multidimensional arrays in Java?
In Java, you can create multidimensional arrays (arrays of arrays) by adding additional square brackets when declaring the array. For example, to create a 2-dimensional array, you can declare it like this:
int[][] my2DArray = new int[3][4];
This creates a 2D array with 3 rows and 4 columns. You can access elements in a multidimensional array by specifying multiple indices, like this:
my2DArray[0][1] = 42; // Sets the element at row 0, column 1 to 42
You can also initialize a multidimensional array in a single line like this:
int[][] my2DArray = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
To iterate through a multidimensional array, you can use nested loops:
for (int i = 0; i < my2DArray.length; i++) { for (int j = 0; j < my2DArray[i].length; j++) { System.out.print(my2DArray[i][j] + " "); } System.out.println(); }