Handling ArrayIndexOutOfBoundsException in Java
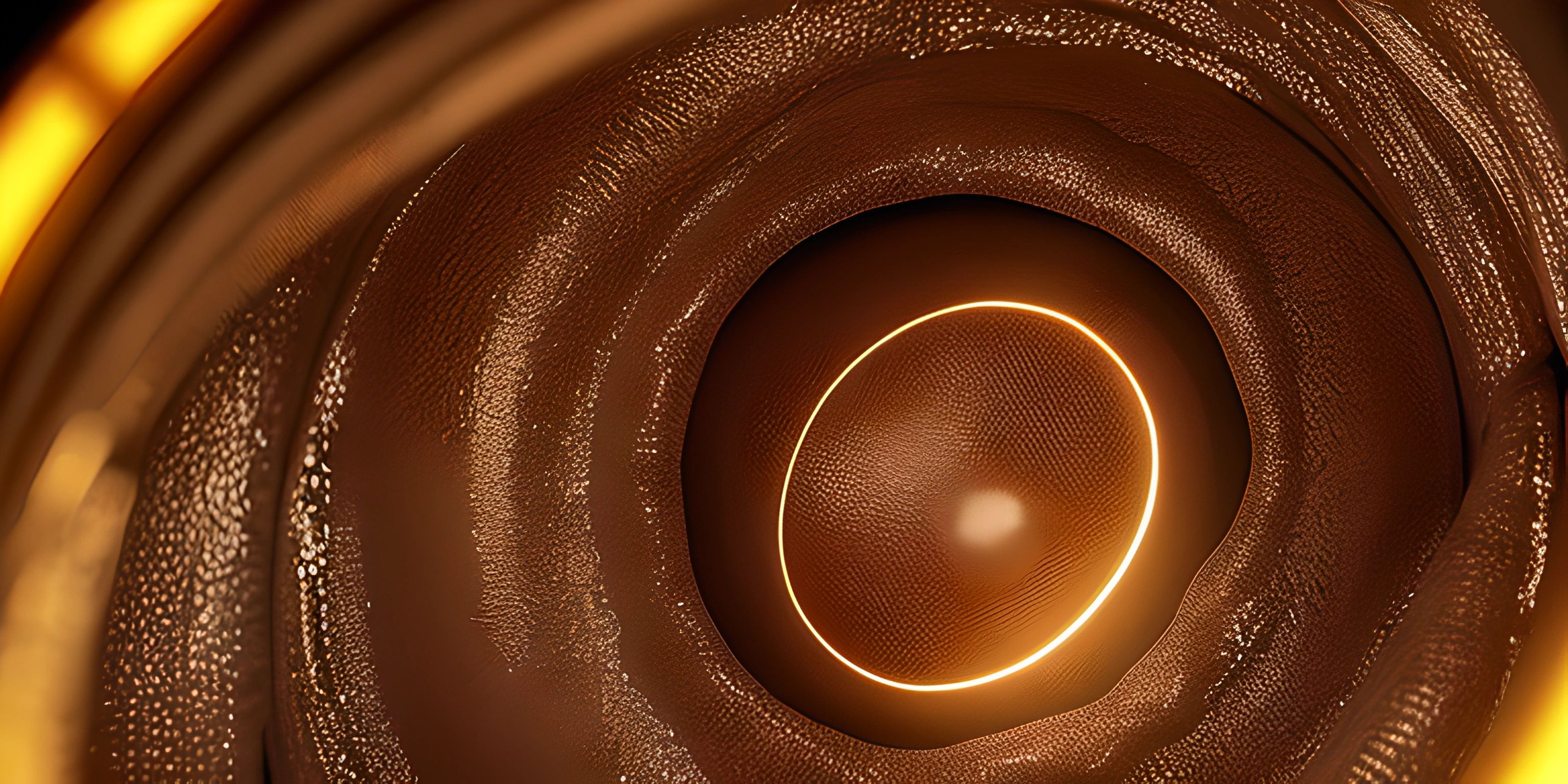
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with arrays in Java, it's quite common to run into the infamous ArrayIndexOutOfBoundsException
. This exception occurs when an attempt is made to access an array element with an index that is either negative or greater than or equal to the array's length. In this article, we'll explore how to handle this exception and prevent it from causing issues in your Java programs.
Understanding ArrayIndexOutOfBoundsException
Before we dive into handling the exception, it's essential to understand what causes it. In Java, arrays have a fixed size, and their indices start from 0 and go up to length - 1
. Accessing an element outside this range will result in an ArrayIndexOutOfBoundsException
.
Here's a simple example that demonstrates this exception:
public class Main { public static void main(String[] args) { int[] numbers = {10, 20, 30}; System.out.println(numbers[3]); // Throws ArrayIndexOutOfBoundsException } }
In this example, the numbers
array has a length of 3. However, we're trying to access the element at index 3, which doesn't exist (the valid indices are 0, 1, and 2), resulting in the exception.
Handling the Exception
One way to handle the ArrayIndexOutOfBoundsException
is to use a try-catch block. When an exception is thrown inside a try block, the catch block will handle it.
public class Main { public static void main(String[] args) { int[] numbers = {10, 20, 30}; try { System.out.println(numbers[3]); // Throws ArrayIndexOutOfBoundsException } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Invalid array index: " + e.getMessage()); } } }
In this example, we've enclosed the problematic code inside a try block. If the exception is thrown, it will be caught and handled by the catch block, which prints an error message.
Preventing the Exception
While using try-catch blocks can handle exceptions, it's often better to prevent them from occurring in the first place. In the case of ArrayIndexOutOfBoundsException
, you can prevent it by checking the array index before accessing it.
public class Main { public static void main(String[] args) { int[] numbers = {10, 20, 30}; int index = 3; if (index >= 0 && index < numbers.length) { System.out.println(numbers[index]); } else { System.out.println("Invalid array index: " + index); } } }
In this example, we've added a conditional check before accessing the array element. If the index is within the valid range, we print the element; otherwise, we print an error message.
By using this approach, we can prevent the ArrayIndexOutOfBoundsException
from occurring and maintain better control over our program's execution.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What is ArrayIndexOutOfBoundsException?
ArrayIndexOutOfBoundsException is a runtime exception in Java that occurs when an attempt is made to access an array element with an index that is either negative or greater than or equal to the array's length.
How can I handle the ArrayIndexOutOfBoundsException?
You can handle the ArrayIndexOutOfBoundsException using a try-catch block. Enclose the code that may cause the exception in a try block and use a catch block to handle the exception if it occurs.
How can I prevent the ArrayIndexOutOfBoundsException from occurring?
You can prevent the ArrayIndexOutOfBoundsException by checking the array index before accessing it. Ensure the index is within the valid range (0 to length - 1) before trying to access an array element.