Java Arrays: Introduction
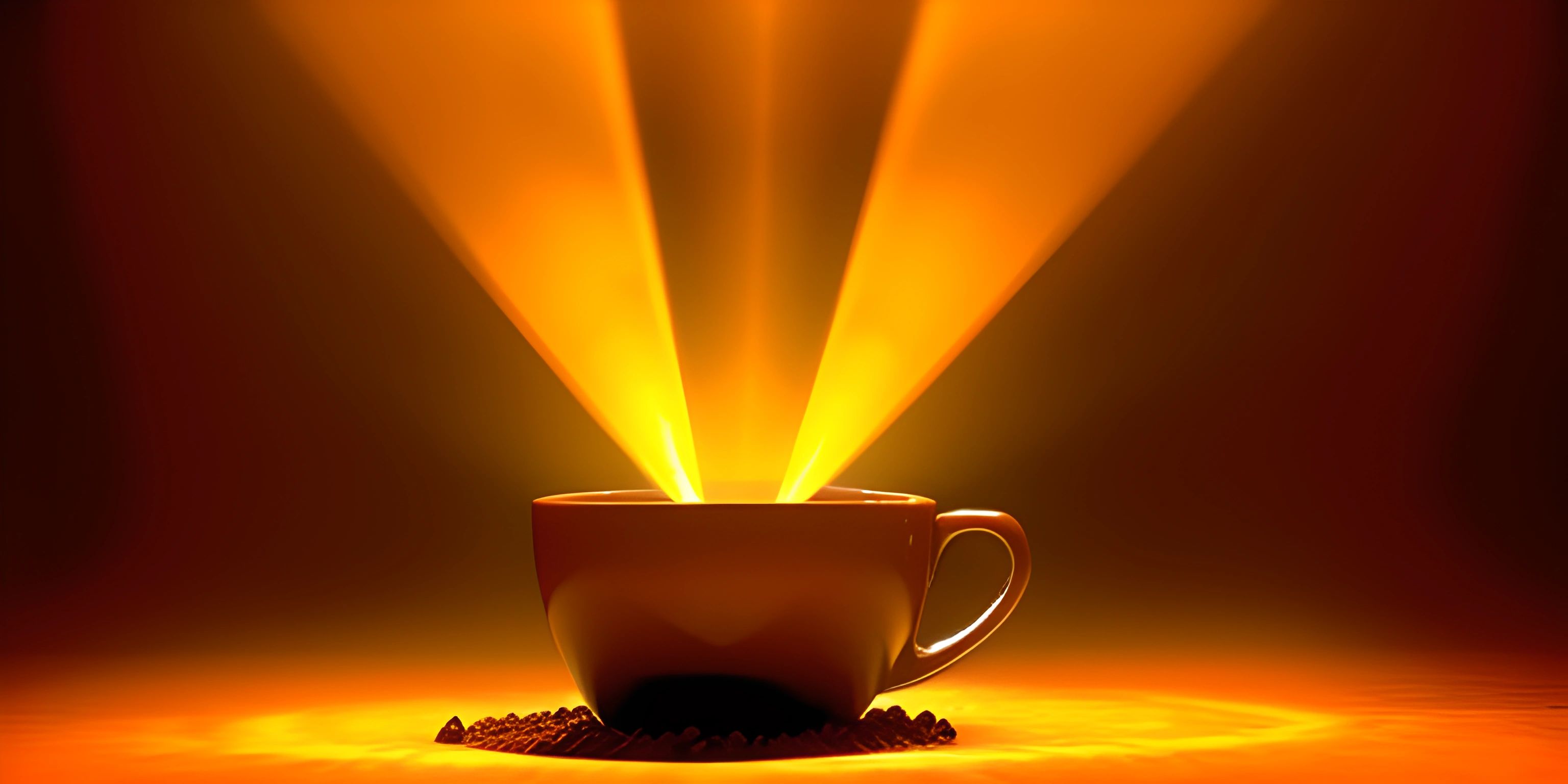
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ah, arrays! The bread and butter of many programming tasks. In Java, arrays are powerful data structures that store a collection of elements of the same type. They are incredibly handy when you want to store and manipulate a group of related data. So, buckle up as we dive into the world of Java arrays!
What are Java Arrays?
In Java, an array is a fixed-size data structure that can store elements of the same data type (such as integers, strings, or even custom objects). The elements in an array are stored in sequential order, and you can access them using an index, which starts at 0
.
Declaring and Initializing Arrays
To declare an array in Java, you need to specify its data type followed by square brackets []
and the array's name. To initialize an array, you can either assign its size using the new
keyword or directly assign the elements using curly braces {}
. Here's an example:
// Declare and initialize an array of integers int[] numbers = new int[5]; // This array can hold 5 integer elements // Declare and initialize an array of strings String[] names = {"Alice", "Bob", "Charlie"};
Accessing Array Elements
To access an array element, use its index within square brackets []
after the array's name. Remember, the index starts from 0
. Here's how you can access and modify elements in an array:
int[] numbers = {10, 20, 30, 40, 50}; // Access the first element (index 0) int firstNumber = numbers[0]; // 10 // Modify the third element (index 2) numbers[2] = 100; // The array now looks like {10, 20, 100, 40, 50}
Looping Over Arrays
Looping through arrays is a common operation, and you can use either a for
loop or a for-each
loop to iterate through the elements. Here's an example:
String[] names = {"Alice", "Bob", "Charlie"}; // Using a for loop for (int i = 0; i < names.length; i++) { System.out.println(names[i]); } // Using a for-each loop for (String name : names) { System.out.println(name); }
Multidimensional Arrays
Java also supports multidimensional arrays, which are arrays of arrays. The most common example is a two-dimensional array, which is essentially a table with rows and columns. To declare and initialize a two-dimensional array, use double square brackets [][]
:
// Declare and initialize a 2D array of integers int[][] matrix = new int[3][3]; // This is a 3x3 matrix // Declare and initialize a 2D array with values int[][] coordinates = { {1, 2}, {3, 4}, {5, 6} };
Accessing and modifying elements in a multidimensional array is similar to the process for a one-dimensional array:
int[][] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; // Access the element at row 1, column 2 int element = matrix[1][2]; // 6 // Modify the element at row 0, column 1 matrix[0][1] = 10; // The matrix now looks like {{1, 10, 3}, {4, 5, 6}, {7, 8, 9}}
With this introduction to Java arrays, you're now ready to harness their power in your programs! As you practice and explore more, you'll discover various methods and techniques to work with arrays, making your code more efficient and elegant. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reading Data Files (psst, it's free!).