MATLAB Array Operations
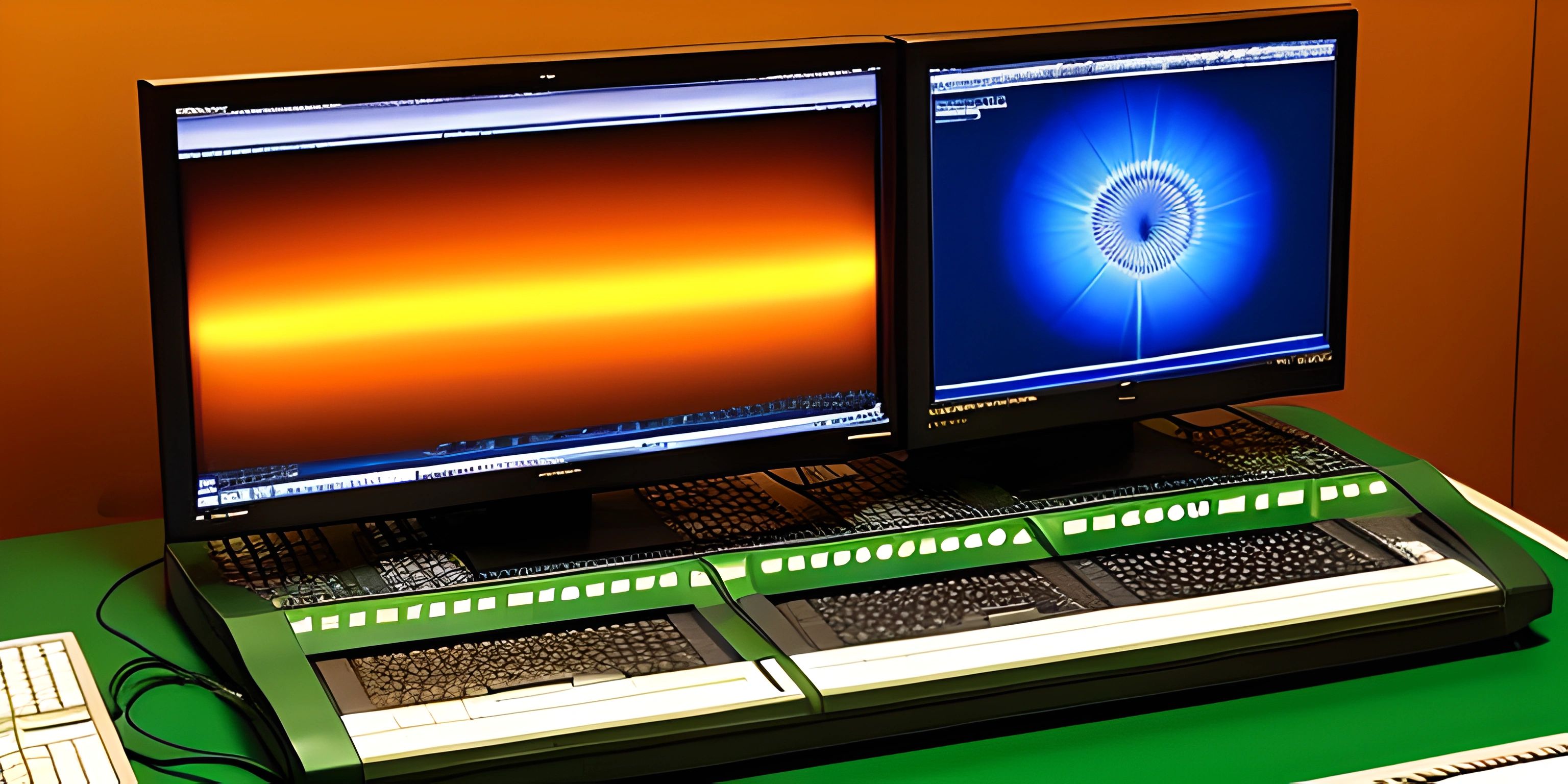
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB is an incredibly popular programming language for engineers, scientists, and researchers. One of its most powerful features is its built-in support for array manipulation. In this article, we'll dive into some common array operations in MATLAB, including element-wise operations, array concatenation, and reshaping.
Element-wise Operations
In MATLAB, we can perform element-wise operations on arrays using the .*
, ./
, and .^
operators. These operators perform multiplication, division, and exponentiation, respectively, on each element of the arrays. Let's see some examples:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; B = [9, 8, 7; 6, 5, 4; 3, 2, 1]; % Element-wise multiplication C = A .* B; % C = [9, 16, 21; 24, 25, 24; 21, 16, 9] % Element-wise division D = A ./ B; % D = [0.1111, 0.2500, 0.4286; 0.6667, 1.0000, 1.5000; 2.3333, 4.0000, 9.0000] % Element-wise exponentiation E = A .^ B; % E = [1, 256, 2187; 4096, 3125, 1296; 343, 64, 9]
Array Concatenation
We can concatenate arrays in MATLAB using square brackets []
. We can concatenate arrays either horizontally (side-by-side) or vertically (one on top of the other).
Horizontal Concatenation
To concatenate arrays horizontally, we place them side-by-side within square brackets, separated by a space or a comma:
A = [1, 2, 3]; B = [4, 5, 6]; C = [A, B]; % or [A B] % C = [1, 2, 3, 4, 5, 6]
Vertical Concatenation
To concatenate arrays vertically, we place them one on top of the other within square brackets, separated by a semicolon:
A = [1, 2, 3]; B = [4, 5, 6]; C = [A; B]; % C = [1, 2, 3; % 4, 5, 6]
Reshaping Arrays
MATLAB provides the reshape
function to change the dimensions of an array without altering its elements. The syntax for the function is as follows:
B = reshape(A, m, n);
Where A
is the input array, m
and n
are the desired number of rows and columns, respectively, and B
is the reshaped output array.
For example:
A = [1, 2, 3, 4, 5, 6]; B = reshape(A, 2, 3); % B = [1, 3, 5; % 2, 4, 6]
Keep in mind that the product of the new dimensions (i.e., m
times n
) must be equal to the number of elements in the original array.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).
FAQ
How do I perform element-wise operations in MATLAB?
In MATLAB, you can perform element-wise operations using the .*
, ./
, and .^
operators for element-wise multiplication, division, and exponentiation, respectively. For example, C = A .* B
will perform element-wise multiplication between arrays A and B, and store the result in C.
How can I concatenate arrays in MATLAB?
To concatenate arrays in MATLAB, you can use square brackets []
. To concatenate arrays horizontally (side-by-side), place them within the square brackets separated by a space or a comma. To concatenate arrays vertically (one on top of the other), place them within the square brackets separated by a semicolon.
How can I reshape an array in MATLAB?
To reshape an array in MATLAB, you can use the reshape
function. The syntax is B = reshape(A, m, n)
, where A
is the input array, m
and n
are the desired number of rows and columns, respectively, and B
is the reshaped output array. The product of the new dimensions (m
times n
) must be equal to the number of elements in the original array.