Java Arrays for Beginners
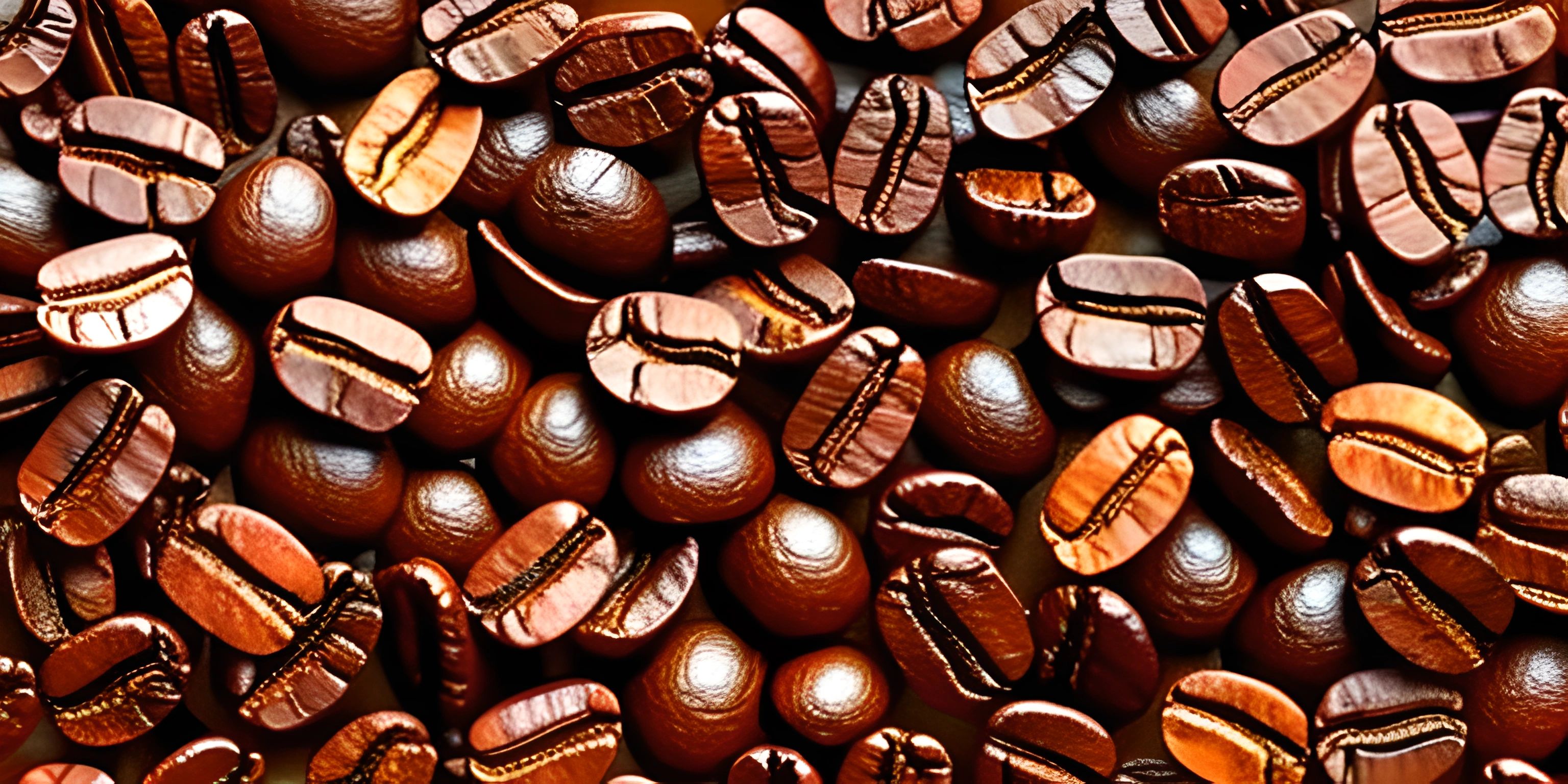
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a versatile programming language that comes with a rich set of data structures, and one of the most fundamental is the array. Arrays are a powerful way to store and organize data in your Java programs. They allow you to store a collection of elements of the same type in a single variable, making it easy to manage and access the data. In this article, you'll dive into the world of Java arrays and learn some basic operations you can perform on them.
What is an Array?
An array is a collection of elements, where each element is of the same data type. Elements in an array are stored in contiguous memory locations, making it easy to access them using an index. The index of an array starts from 0
and goes up to n-1
, where n
is the number of elements in the array.
Creating an Array in Java
In Java, you can create an array by declaring its data type followed by square brackets []
, and then providing a name for the array. You can initialize the array by specifying its size, or you can directly assign the values during declaration. Here's an example of creating an integer array:
int[] myNumbers = new int[5]; // creates an array of size 5
You can also initialize the array with values directly:
int[] myNumbers = {1, 2, 3, 4, 5}; // creates an array with the given values
Accessing Array Elements
You can access an array element by specifying its index within square brackets []
after the array's name. Remember that the index starts at 0
. Here's an example:
int[] myNumbers = {1, 2, 3, 4, 5}; int firstElement = myNumbers[0]; // accesses the first element in the array
Modifying Array Elements
Modifying an element in an array is as simple as accessing it and assigning a new value. Here's an example:
int[] myNumbers = {1, 2, 3, 4, 5}; myNumbers[2] = 42; // changes the third element to 42
Looping Through an Array
To loop through an array, you can use a for
loop. You can either use a traditional for
loop with an index or use the enhanced for
loop (also known as a "for-each" loop). Here's an example using both loops:
int[] myNumbers = {1, 2, 3, 4, 5}; // using a traditional for loop for (int i = 0; i < myNumbers.length; i++) { System.out.println(myNumbers[i]); } // using an enhanced for loop for (int number : myNumbers) { System.out.println(number); }
Now you're equipped with the basics of Java arrays and ready to start using them in your programs. As you continue learning and growing as a programmer, you'll discover more advanced features and operations that you can perform on arrays. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Word Splitter (psst, it's free!).