Java Runtime for Beginners
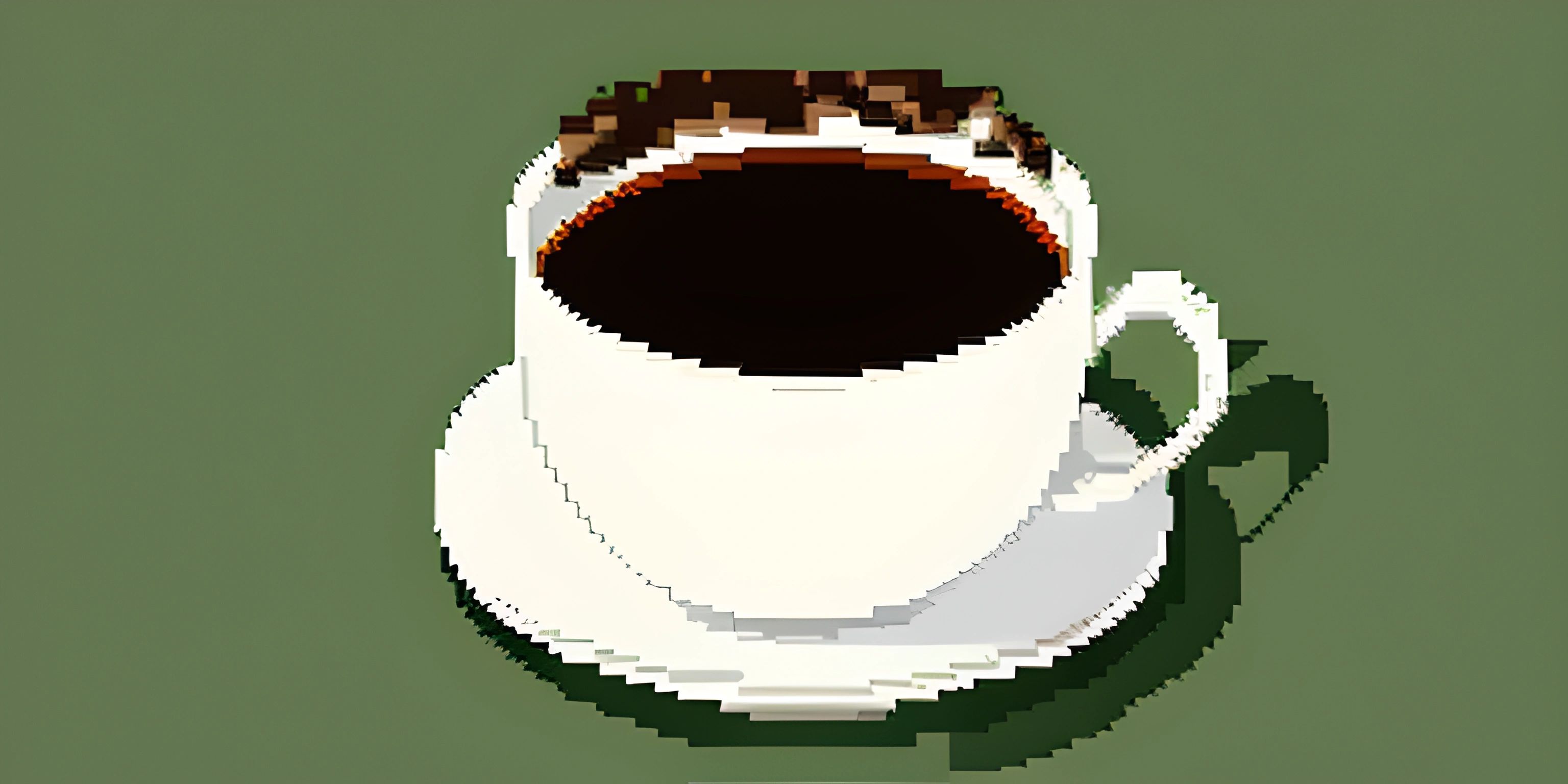
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of Java! As one of the most popular programming languages in the world, it's a great starting point for beginners who want to dive into coding. In this article, we'll cover the basics of the Java programming language, its runtime environment, and some fundamental concepts.
Java Runtime Environment (JRE)
When you write and run a Java program, the code isn't executed directly by your computer's hardware. Instead, it's processed by the Java Runtime Environment (JRE), a piece of software that translates your code into something your computer can understand.
The JRE is part of the larger Java Development Kit (JDK), which includes everything you need to not only run but also develop Java applications. The JDK comes with the Java Virtual Machine (JVM), a software tool that converts your Java code into bytecode – a platform-independent, machine-readable format.
With the JVM, you can write Java code once and run it on any platform that supports Java. This "write once, run anywhere" feature is one of the reasons Java is so popular.
Hello, World! in Java
To give you a taste of what Java code looks like, let's start with the classic "Hello, World!" example:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
This code defines a class called HelloWorld
and a main
method within it. The main
method is the entry point of any Java program, and it's where the JVM starts executing your code. In this case, the main
method simply prints "Hello, World!" to the console.
Basic Java Concepts
Now that you've seen a simple Java program, let's explore some fundamental concepts in the language.
Variables
In Java, you store data in variables. To create a variable, you need to declare its type and give it a name, like so:
int myNumber = 42;
Here, we've created a variable named myNumber
of type int
(short for integer) and assigned it the value 42. Java has several built-in data types, such as int
, float
, double
, boolean
, and char
, as well as custom types you can define yourself using classes.
Conditional Statements
Java allows you to make decisions based on conditions using if-else statements. Here's a quick example:
int temperature = 22; if (temperature > 30) { System.out.println("It's hot outside!"); } else { System.out.println("It's not too hot."); }
In this code, we check if the temperature
variable is greater than 30. If it is, we print "It's hot outside!"; otherwise, we print "It's not too hot.".
Loops
When you want to repeat a block of code multiple times, you can use a loop. Java has several types of loops, such as for and while loops. Here's an example of a simple for
loop:
for (int i = 0; i < 5; i++) { System.out.println("Iteration number: " + i); }
This loop prints the numbers 0 through 4, along with the text "Iteration number:".
Conclusion
There's much more to learn about Java, but this brief introduction should give you a good starting point. As you continue to explore this powerful language, you'll come across concepts like methods, arrays, and object-oriented programming. Enjoy your journey through the world of Java programming!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the Java Runtime Environment (JRE)?
The Java Runtime Environment (JRE) is a software package that provides the necessary components to run Java applications. It includes the Java Virtual Machine (JVM), core libraries, and other components required to execute Java bytecode. Simply put, JRE is the environment where your Java programs run.
How do I install the Java Runtime Environment?
To install the Java Runtime Environment, follow these steps:
- Visit the official Oracle website to download the JRE: Java SE Downloads
- Choose the appropriate version for your operating system (Windows, macOS, or Linux).
- Follow the installation instructions provided on the website.
What is the role of the Java Virtual Machine (JVM) in Java Runtime?
The Java Virtual Machine (JVM) is a critical component of the Java Runtime Environment. It's responsible for converting Java bytecode into machine code that can be executed by the host computer. The JVM ensures that Java applications can run on any platform that has a compatible JVM, providing the "write once, run anywhere" capability that makes Java so versatile.
Can you provide a simple example of a Java program to understand the basic concepts?
Sure! Here's a basic Java program that prints "Hello, World!" to the console:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
To run this program, save it as HelloWorld.java
, then compile it using the Java Development Kit (JDK) with the command javac HelloWorld.java
. Finally, run the compiled bytecode using the JRE with the command java HelloWorld
. You should see "Hello, World!" printed on your screen.
What are some common Java libraries and tools included in the Java Runtime Environment?
The Java Runtime Environment includes a wide variety of libraries and tools that make it easier to develop and run Java applications. Some common ones are:
java.lang
: Contains fundamental classes and interfaces, such asObject
,String
, andSystem
.java.util
: Provides a collection of utility classes, likeArrayList
,HashMap
, andDate
.java.io
: Contains classes for handling input and output streams, file operations, and serialization.java.net
: Offers classes for implementing networking applications, such as sockets and URL handling.java.math
: Includes classes for performing arithmetic operations likeBigInteger
andBigDecimal
.java.nio
: Provides non-blocking I/O capabilities and advanced file operations.java.security
: Contains classes for implementing security features, such as encryption and digital signatures.