Java Polymorphism Basics
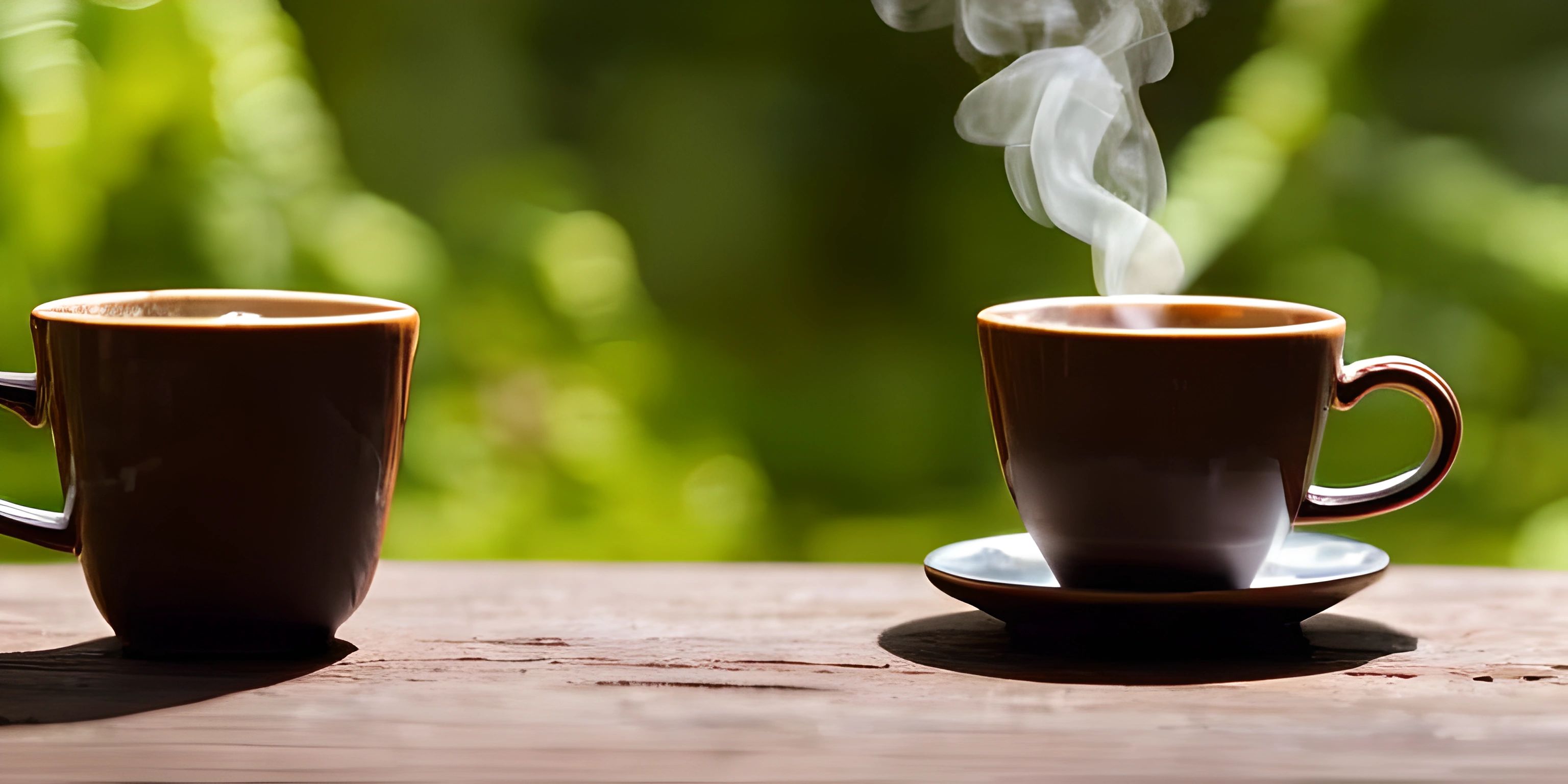
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Polymorphism is a powerful concept in Object-Oriented Programming (OOP) that allows us to write more flexible and reusable code. In Java, polymorphism is implemented through inheritance and interfaces. It's a magical trick that lets a single name represent multiple types of objects.
Inheritance and Polymorphism
Inheritance in Java is a mechanism that allows one class to inherit the properties and methods of another class. Polymorphism, on the other hand, enables a subclass to provide a specific implementation of a method that's already defined in its superclass. This technique is called method overriding.
Let's start with a simple example of inheritance. Suppose we have a base class Animal
and two subclasses Dog
and Cat
:
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Dog extends Animal { void makeSound() { System.out.println("The dog barks"); } } class Cat extends Animal { void makeSound() { System.out.println("The cat meows"); } }
Here, Dog
and Cat
override the makeSound
method from Animal
. Now, let's see polymorphism in action:
class Main { public static void main(String[] args) { Animal myAnimal = new Animal(); Animal myDog = new Dog(); Animal myCat = new Cat(); myAnimal.makeSound(); // The animal makes a sound myDog.makeSound(); // The dog barks myCat.makeSound(); // The cat meows } }
Even though the type of myDog
and myCat
variables is Animal
, they call the makeSound
method of their respective subclasses, thanks to polymorphism.
Interfaces and Polymorphism
Interfaces in Java provide another way to achieve polymorphism. An interface is a collection of abstract methods (methods without a body) that can be implemented by any class. A class can implement multiple interfaces, allowing for a more flexible design.
Let's create an interface SoundMaker
:
interface SoundMaker { void makeSound(); }
Now, we can implement this interface in our Dog
and Cat
classes:
class Dog implements SoundMaker { void makeSound() { System.out.println("The dog barks"); } } class Cat implements SoundMaker { void makeSound() { System.out.println("The cat meows"); } }
With the interface in place, we can use polymorphism to create an array of SoundMaker
objects, regardless of their actual type:
class Main { public static void main(String[] args) { SoundMaker[] soundMakers = new SoundMaker[3]; soundMakers[0] = new Dog(); soundMakers[1] = new Cat(); soundMakers[2] = new Dog(); for (SoundMaker sm : soundMakers) { sm.makeSound(); // The dog barks, The cat meows, The dog barks } } }
This example demonstrates the power of polymorphism in creating flexible code that can work with different objects that share a common behavior, in this case, making sounds.
Conclusion
Polymorphism in Java opens up a world of possibilities in designing flexible and reusable code. It allows us to create objects that can take on multiple forms, depending on their actual type. Through inheritance and interfaces, we can achieve polymorphism and create code that is efficient and more maintainable. So, next time you encounter a pack of dogs and cats, remember the magic of polymorphism that makes them all sound makers in the world of Java.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is polymorphism in Java?
Polymorphism is one of the four fundamental principles of object-oriented programming (OOP). In Java, polymorphism allows objects of different classes to be treated as objects of a common superclass. This provides flexibility and reusability of code, as methods can work with different types of objects without knowing their specific class at compile-time.
How do you implement polymorphism in Java?
In Java, polymorphism is implemented using inheritance, interfaces, and method overriding. Here's a simple example using inheritance and method overriding:
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Dog extends Animal { @Override void makeSound() { System.out.println("The dog barks"); } } class Cat extends Animal { @Override void makeSound() { System.out.println("The cat meows"); } } public class Main { public static void main(String[] args) { Animal myAnimal = new Dog(); myAnimal.makeSound(); // Output: The dog barks } }
What is the difference between static and dynamic polymorphism in Java?
Static polymorphism, also known as compile-time polymorphism, occurs when the exact method to be called is determined during compile-time. This is achieved through method overloading, where a class has multiple methods with the same name but different parameters. Dynamic polymorphism, or runtime polymorphism, occurs when the exact method to be called is determined during runtime. This is achieved through method overriding and inheritance, allowing a subclass to provide a specific implementation of a method that is already defined in its superclass.
Can interfaces be used to achieve polymorphism in Java?
Yes, interfaces can be used to achieve polymorphism in Java. When a class implements an interface, it must provide an implementation for all of its methods. This allows objects of different classes to be treated as objects of a common interface, providing flexibility and reusability of code. Here's an example:
interface Shape { void draw(); } class Circle implements Shape { @Override public void draw() { System.out.println("Drawing a circle"); } } class Square implements Shape { @Override public void draw() { System.out.println("Drawing a square"); } } public class Main { public static void main(String[] args) { Shape myShape = new Circle(); myShape.draw(); // Output: Drawing a circle } }