Working with Constants in Java
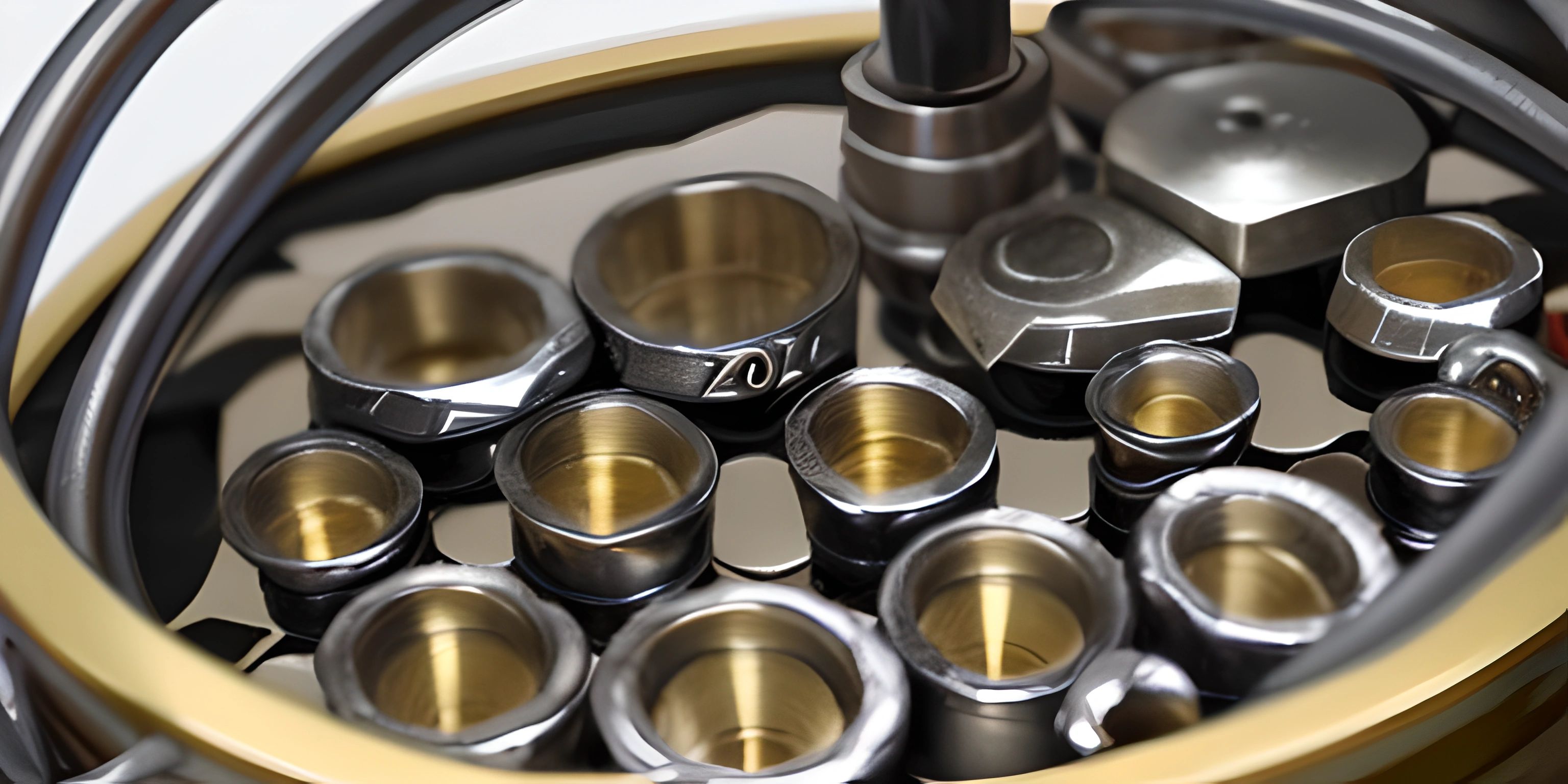
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In Java, constants are employed to store values that should not be changed after they are first set. This can be useful in various situations, such as defining values that remain constant throughout a program's execution, like the value of pi or the number of days in a week. By using constants, you can help ensure that your code remains consistent, easier to maintain, and less prone to errors.
Creating Constants in Java
In Java, constants are created using the final
keyword. When you declare a variable as final
, it can only be assigned a value once. After that, the value cannot be changed. This is how you create a constant in Java.
Here's an example of creating a constant in Java:
final int DAYS_IN_WEEK = 7;
In this example, we've created a constant named DAYS_IN_WEEK
and assigned it the value 7
. The final
keyword indicates that this value should not be changed.
Why Use Constants?
Using constants in your Java programs offers several benefits:
-
Immutability: Constants help ensure that once a value is set, it cannot be changed unintentionally. This makes your code less susceptible to bugs caused by accidental changes to important values.
-
Readability: Constants are typically given meaningful names, which makes your code more readable and easier to understand.
-
Maintainability: When you use constants, you can easily update the value of a constant in one place, and the change will be reflected throughout your code. This can save you time and effort when making updates.
Constants Naming Convention
In Java, it's a common convention to name constants using uppercase letters with words separated by underscores. This makes them easy to identify and distinguish from regular variables. For example:
final double PI = 3.14159; final String COMPANY_NAME = "Cratecode";
Constants in Action
Let's look at an example of how constants can be useful in a Java program. Suppose we're working on a program that calculates the circumference of a circle. We know that the formula for the circumference is 2 * PI * radius
. We can use a constant for the value of pi:
public class CircleCircumference { public static void main(String[] args) { final double PI = 3.14159; double radius = 5.0; double circumference = 2 * PI * radius; System.out.println("The circumference of the circle is: " + circumference); } }
By using a constant for the value of pi, we've made our code more readable and less prone to errors. If we need to update the value of pi, we can do so in one place, and the change will be reflected throughout our code.
In conclusion, constants are an essential programming concept in Java that can help improve your code's readability, maintainability, and reduce the likelihood of errors. By creating constants using the final
keyword and following Java's naming conventions, you can make your programs more robust and efficient.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is a constant in Java and why is it important?
A constant in Java is a variable whose value cannot be changed once it is assigned. Constants are important because they help in preventing accidental changes to values that should remain constant throughout the execution of a program. They make the code more readable and maintainable, and also provide a central point of control for values that are used in multiple places.
How do I create a constant in Java?
In Java, you can create a constant by using the final
keyword along with the variable declaration. This ensures that the value of the variable cannot be changed after it has been initialized. Here's an example:
public static final double PI = 3.14159;
In this example, we've declared a constant named PI
with the value 3.14159
. The public static final
keywords make it a constant accessible from anywhere in the code.
Can I create constants for different data types?
Yes, you can create constants for any data type in Java, such as int
, float
, double
, boolean
, String
, and other object types. Just make sure to use the final
keyword along with the variable declaration. For example:
public static final int MAXIMUM_ATTEMPTS = 3; public static final String WELCOME_MESSAGE = "Welcome to Cratecode!";
In this example, we've declared two constants, MAXIMUM_ATTEMPTS
of type int
and WELCOME_MESSAGE
of type String
.
How do I access and use constants in my Java code?
To access and use a constant in your Java code, simply reference it by its name. Since constants are usually declared with the public static
keywords, you can access them directly using the class name followed by the constant name. For example:
public class MathUtils { public static final double PI = 3.14159; } public class Circle { public double calculateArea(double radius) { return MathUtils.PI * radius * radius; } }
In this example, the PI
constant from the MathUtils
class is used in the calculateArea
method of the Circle
class.
Can I modify the value of a constant after it has been initialized?
No, you cannot modify the value of a constant after it has been initialized. The final
keyword ensures that the value of the variable cannot be changed after its initialization. Attempting to modify the value will result in a compilation error.