Java Learning Roadmap
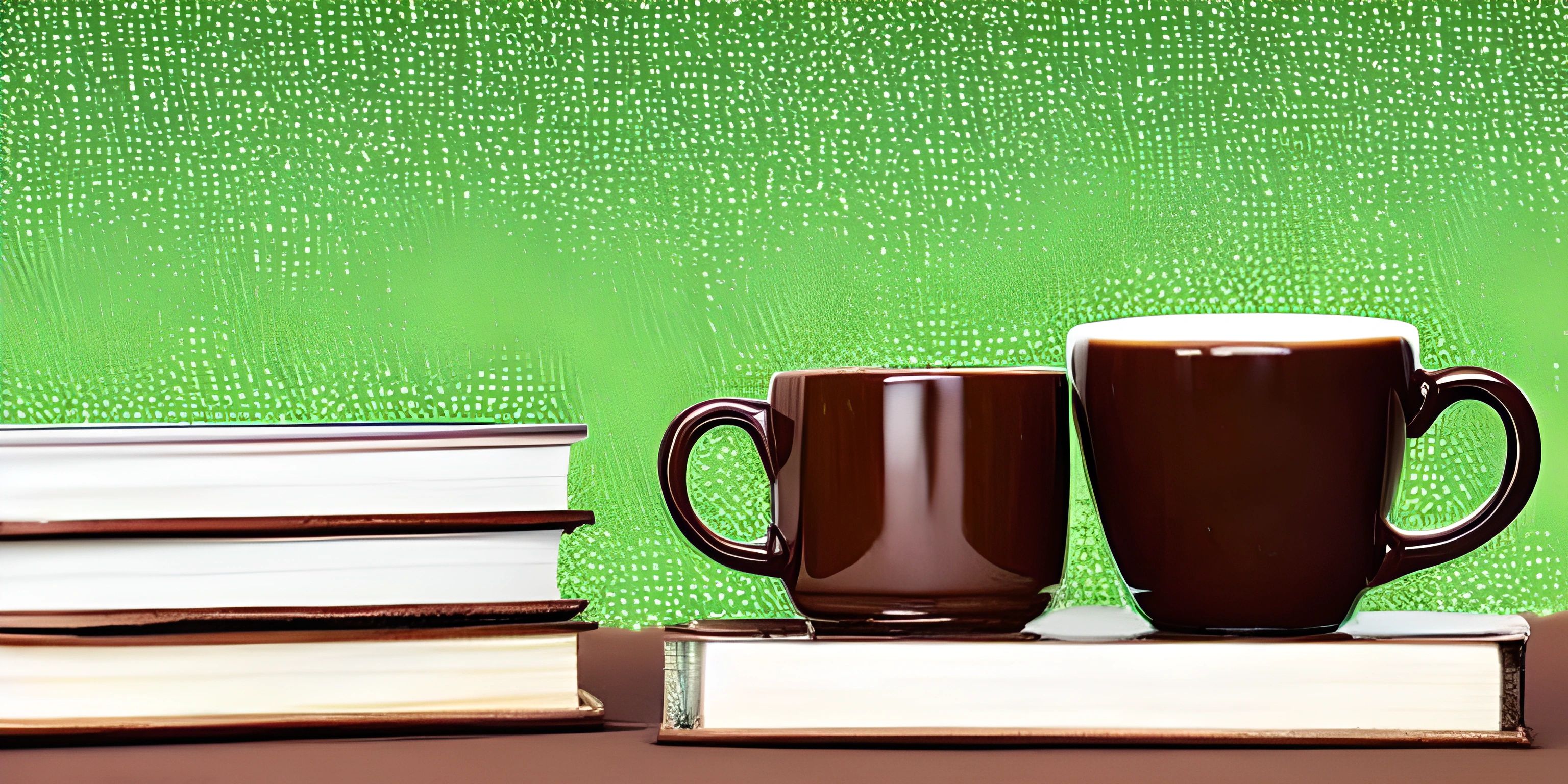
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Embarking on your Java programming journey? Welcome aboard! This Java learning roadmap is designed to guide you through the key concepts you'll encounter on your way to becoming a proficient Java developer. Buckle up, and let's dive into the world of Java!
Getting Started with Java
Java is a widely-used programming language known for its platform independence, versatility, and object-oriented approach. Before you start writing Java code, you'll first need to install Java on your machine and understand the Java Development Kit (JDK).
Java Basics
-
Syntax and Data Types: Start by learning Java's basic syntax and data types, such as integers, floats, and booleans.
-
Variables and Constants: Get familiar with variables and constants to store and manipulate data in your programs.
-
Control Structures: Learn how to use conditional statements and loops to control the flow of your programs.
-
Arrays and Collections: Understand how to work with arrays and collections, which are essential for managing groups of objects.
Object-Oriented Programming
Java is an object-oriented programming language, so mastering its core principles is crucial:
-
Classes and Objects: Learn how to define classes and create objects as instances of those classes.
-
Inheritance and Polymorphism: Delve into inheritance and polymorphism to promote code reusability and flexibility.
-
Encapsulation and Abstraction: Study encapsulation and abstraction to improve the modularity and maintainability of your code.
Advanced Java Concepts
Once you're comfortable with the basics, it's time to explore more advanced topics:
-
Exception Handling: Learn how to handle exceptions gracefully to ensure your programs can recover from unexpected situations.
-
File I/O and Serialization: Understand how to work with files and perform serialization to store and retrieve data.
-
Concurrency: Discover Java's concurrency features to build efficient, high-performance programs that can execute tasks in parallel.
-
Networking: Explore Java's networking capabilities to create applications that communicate over the internet.
Java Libraries and Frameworks
As you become more proficient in Java, you'll want to explore popular libraries and frameworks that can help you build complex applications with ease:
-
JavaFX: Dive into JavaFX to create visually stunning and interactive desktop applications.
-
Spring: Learn the Spring framework to build scalable, enterprise-level applications with ease.
-
Hibernate: Get acquainted with Hibernate for efficient object-relational mapping and database management.
This roadmap is just the beginning of your Java adventure. As you progress, you'll find countless resources and communities to help you along the way. Keep learning, practicing, and building projects to hone your skills and become a confident Java developer. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What are the key concepts I should focus on while learning Java?
The key concepts to focus on while learning Java include:
- Basic syntax and data types
- Control structures (loops, conditionals)
- Object-oriented programming (classes, inheritance, encapsulation, polymorphism)
- Exception handling
- File I/O
- Multithreading and concurrency
- Collections and generics
- Networking and sockets
- Java libraries and APIs
- Java frameworks (Spring, Hibernate)
How can I start learning Java from scratch?
To start learning Java from scratch, follow these steps:
- Install the Java Development Kit (JDK) on your computer.
- Set up an Integrated Development Environment (IDE) for Java, such as Eclipse or IntelliJ IDEA.
- Begin with learning the basics of Java syntax, data types, and control structures.
- Progress to object-oriented programming concepts and Java-specific features.
- Practice solving Java programming problems and building small projects.
- Explore Java libraries, frameworks, and advanced topics, such as multithreading and networking.
- Join Java communities and forums to seek guidance, share knowledge, and network with other Java developers.
Which Java libraries and frameworks should I learn to become a proficient Java developer?
Some popular Java libraries and frameworks that can help you become a proficient developer include:
- Spring Framework: An application framework for Java that simplifies the development of Java applications.
- Hibernate ORM: A Java framework for mapping an object-oriented domain model to a relational database.
- JavaFX: A platform for creating rich internet applications using Java.
- JUnit: A testing framework for Java applications.
- Apache Commons: A collection of reusable Java components.
- Log4j: A logging library for Java applications.
- Gson: A Java library for converting Java objects to JSON and vice versa.
- Guava: A set of core libraries for Java, developed by Google.
- Mockito: A mocking framework for unit testing in Java.
How can I improve my problem-solving skills in Java programming?
To improve your problem-solving skills in Java programming, try the following:
- Practice regularly by solving Java programming exercises and challenges available on platforms like LeetCode, HackerRank, and CodeSignal.
- Work on small-to-medium-sized projects to apply your skills in a real-world context.
- Participate in coding competitions or hackathons to test your skills under time constraints and pressure.
- Learn from other developers by reading their code, participating in code reviews, and engaging in discussions on Java forums and communities.
- Continuously update your knowledge about Java best practices, design patterns, and new features introduced in the language.