Java Generics: Wildcards
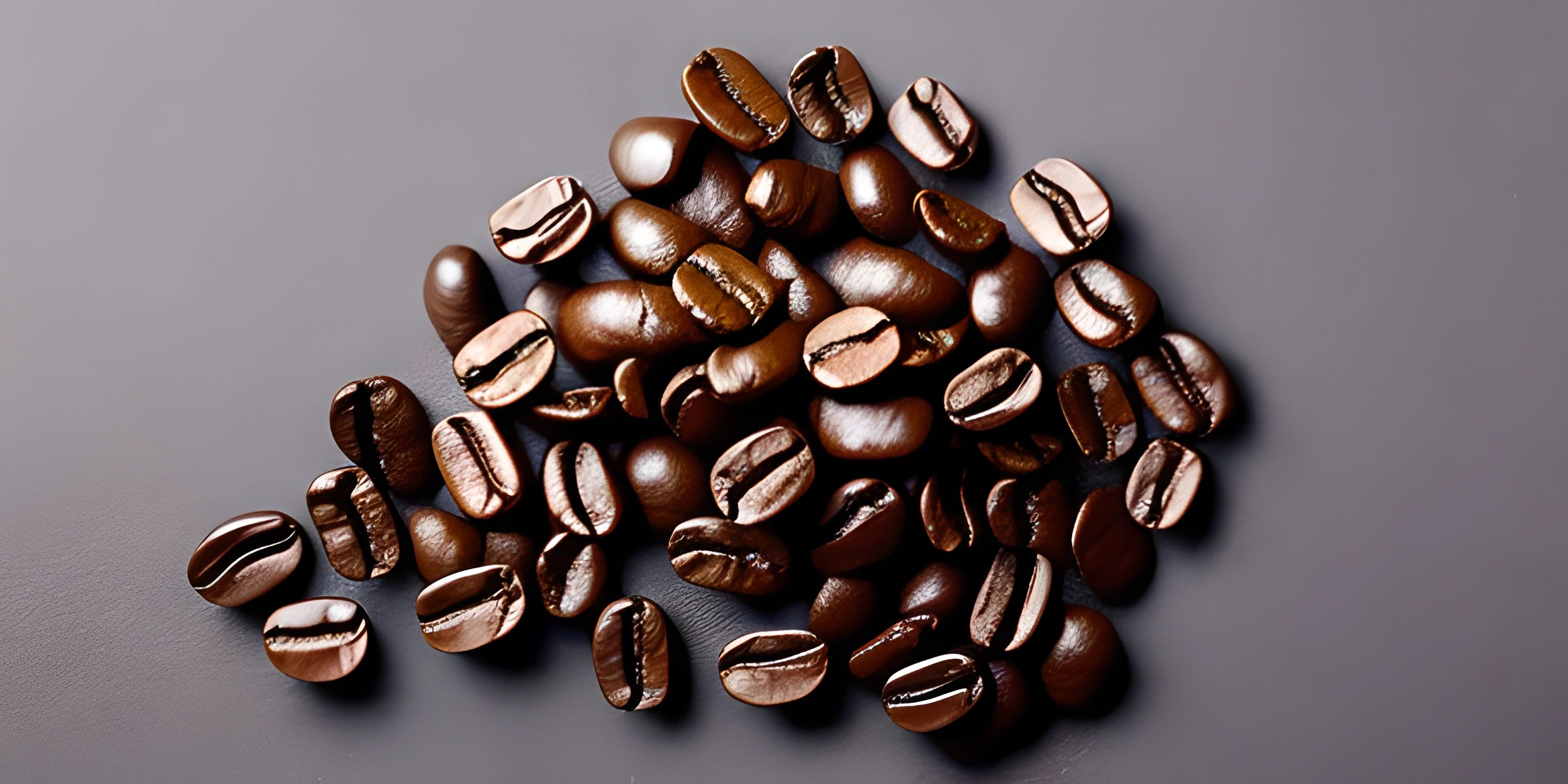
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Generics are a powerful feature in Java that enables you to write more flexible and reusable code. One aspect that makes generics even more versatile is the use of wildcards. They allow you to create more adaptable methods and data structures, enabling your code to work with different types of objects while providing compile-time type safety.
Java Generics and Wildcards
In Java, generics are used to create parameterized types. These types allow you to write code that works with multiple data types while maintaining type safety. A common use case of generics is in collections like List<T>
, where T
is a type parameter representing the type of objects stored in the list.
Wildcards add an extra layer of flexibility to generics by allowing you to work with unknown types. A wildcard is represented by the question mark ?
in the type parameter. There are two kinds of wildcards in Java generics: bounded and unbounded.
Unbounded Wildcards
An unbounded wildcard represents an unknown type. It is useful when you want to write a method that works with a generic type, but you don't care about the specific type parameter. Here's an example using List<?>
:
public static void printList(List<?> list) { for (Object item : list) { System.out.println(item); } }
This printList
method accepts a list of any type and prints its elements. You can use it with a List<Integer>
, List<String>
, or any other type of list.
Bounded Wildcards
Bounded wildcards provide more control over the unknown type by specifying an upper or lower bound for it. There are two types of bounded wildcards: upper-bounded and lower-bounded.
Upper-Bounded Wildcards
An upper-bounded wildcard restricts the unknown type to a specific class or any of its subclasses. It is declared using the ? extends T
syntax. The following example demonstrates how to use an upper-bounded wildcard:
public static double sumOfNumbers(List<? extends Number> numbers) { double sum = 0; for (Number num : numbers) { sum += num.doubleValue(); } return sum; }
The sumOfNumbers
method accepts a list of any type that extends Number
(e.g., Integer
, Double
, Long
). This way, you can use the method with different types of number lists without duplicating code.
Lower-Bounded Wildcards
A lower-bounded wildcard restricts the unknown type to a specific class or any of its superclasses. It is declared using the ? super T
syntax. Here's an example of how to use a lower-bounded wildcard:
public static void addIntegersToList(List<? super Integer> list, int numIntegers) { for (int i = 1; i <= numIntegers; i++) { list.add(i); } }
This addIntegersToList
method accepts a list of any type that is a superclass of Integer
(e.g., Number
, Object
). You can use this method with different types of lists, as long as they can store integers.
Wildcard Usage Tips
When working with wildcards in Java generics, keep the following guidelines in mind:
- PECS principle: "Producer Extends, Consumer Super." Use
? extends T
when your method consumes data (reads) from a generic type, and use? super T
when your method produces data (writes) to a generic type. - Favor upper-bounded wildcards: When in doubt, prefer upper-bounded wildcards over lower-bounded wildcards, as they provide more type safety and are often more useful.
- Use wildcards for flexibility: Wildcards are meant to increase the flexibility of your code. Use them when you want your methods or data structures to work with multiple types, but still maintain type safety.
By understanding and using wildcards in Java generics, you'll be able to create more flexible and reusable code that can adapt to a variety of different data types, all while maintaining compile-time type safety. Wildcards are an invaluable tool to enhance your Java programming skills and write more powerful, adaptable code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Java generics wildcards?
Java generics wildcards are a way to create more flexible and reusable code when working with generic classes and methods. They are represented by the question mark symbol (?
) and allow you to work with unknown types, making it easier to write code that can be reused for different types.
How do I use the `? extends` wildcard in Java generics?
The ? extends
wildcard is used to represent an unknown type that is a subclass of a specific class or interface. For example, if you have a generic class Box<T>
, you can use the ? extends Number
wildcard to accept any Box object holding a subclass of the Number
class, like Integer
, Double
, or Float
. Here's an example:
public static double sum(Box<? extends Number> box) { Number number = box.get(); return number.doubleValue(); }
What is the purpose of the `? super` wildcard in Java generics?
The ? super
wildcard is used to represent an unknown type that is a superclass of a specific class. This is useful when you need to work with objects that are of a certain class or any of its superclasses. For example, if you have a generic class Box<T>
, you can use the ? super Integer
wildcard to accept any Box object holding an Integer
or its superclasses, such as Number
or Object
. Here's an example:
public static void addInt(Box<? super Integer> box, int value) { box.set(value); }
Can I use both upper and lower bounded wildcards in the same method?
Yes, you can use both upper and lower bounded wildcards in the same method. However, keep in mind that using both in the same method can make the code more difficult to understand and maintain. It's important to use wildcards judiciously, ensuring that your code remains flexible, reusable, and easy to read.
How can I use wildcards with generic methods in Java?
To use wildcards with generic methods in Java, you can declare the method with a wildcard type parameter. This allows the method to accept arguments of various types without knowing their exact type. Here's an example:
public static <T> void copy(Box<? super T> dest, Box<? extends T> src) { T value = src.get(); dest.set(value); }
In this example, the copy
method accepts two Box
objects with unknown types, but ensures that the source object's type is a subclass of the destination object's type.