Exploring Java Collections
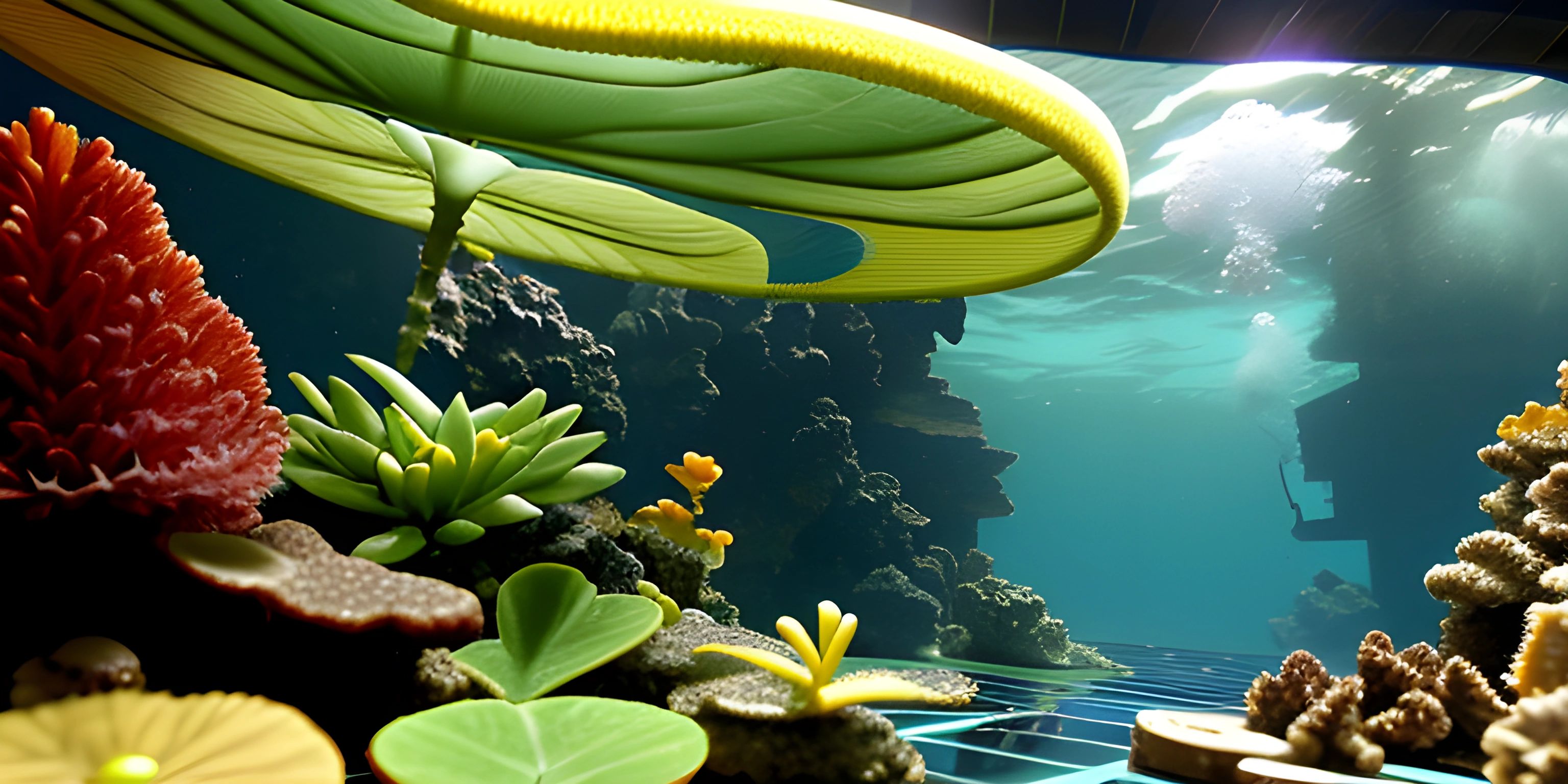
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Java Collections Framework is a powerful set of classes and interfaces designed to help developers manage and manipulate groups of objects with ease. With Collections, you can perform common tasks like searching, sorting, and inserting elements in an efficient and intuitive way.
Java Collections Hierarchy
The Java Collections Framework includes several interfaces and classes, organized into a hierarchy. The main interfaces are Collection
, List
, Set
, Map
, and Queue
. Let's briefly go over each of these interfaces.
Collection Interface
The Collection
interface is the root of the Collections hierarchy and is implemented by all the other interfaces[^1^]. It provides basic methods like adding, removing, and querying elements, along with methods for iterating through the elements.
List Interface
The List
interface extends Collection
and represents an ordered sequence of elements, allowing duplicates. You can access elements by their index, add elements at a specific position, or search for elements. Some popular List
implementations are ArrayList
[^2^] and LinkedList
[^3^].
Set Interface
The Set
interface also extends Collection
but represents an unordered collection of unique elements. There are no duplicate elements in a Set
. Common implementations include HashSet
[^4^] and TreeSet
[^5^].
Map Interface
The Map
interface represents a collection of key-value pairs, where each unique key maps to a single value. It is not a direct descendant of Collection
but is still considered part of the Java Collections Framework. Examples of Map
implementations are HashMap
[^6^] and TreeMap
[^7^].
Queue Interface
The Queue
interface extends Collection
and represents a data structure that holds elements in a specific order, allowing you to add elements at the end and remove elements from the front. This is useful for implementing first-in-first-out (FIFO) data structures. A popular Queue
implementation is LinkedList
[^3^].
Iterators and Iterable
In addition to the main interfaces, the Java Collections Framework includes the Iterator
[^8^] and Iterable
[^9^] interfaces. The Iterator
interface provides a way to traverse collections, while the Iterable
interface represents a collection that can be iterated using an iterator.
Here is an example of using an iterator to traverse an ArrayList
:
List<String> fruits = new ArrayList<>(); fruits.add("apple"); fruits.add("banana"); fruits.add("cherry"); Iterator<String> iterator = fruits.iterator(); while (iterator.hasNext()) { String fruit = iterator.next(); System.out.println(fruit); }
Conclusion
The Java Collections Framework is a versatile and powerful tool for working with groups of objects in Java. By understanding the main interfaces and their implementations, you can choose the right data structure for your specific needs and perform tasks more efficiently. To dive deeper into each interface and implementation, check out their respective Cratecode articles linked above.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the Java Collections Framework?
The Java Collections Framework is a set of classes and interfaces designed to help developers manage and manipulate groups of objects. It includes interfaces like Collection, List, Set, Map, and Queue, along with various implementations for different use cases.
What are the main interfaces in the Java Collections Framework?
The main interfaces in the Java Collections Framework are Collection, List, Set, Map, and Queue. Each interface represents a specific type of data structure, and they offer various methods for working with collections of objects.
What is the difference between a List and a Set?
A List is an ordered sequence of elements that can include duplicates, while a Set is an unordered collection of unique elements (no duplicates). Lists allow you to access elements by their index, while Sets only permit adding, removing, and checking for the presence of elements.
What is an Iterator in Java?
An Iterator is an interface that provides a way to traverse collections. It includes methods like hasNext() and next() to check for the presence of more elements and retrieve the next element in the collection.
How do I choose the right Java Collection for my needs?
To choose the right Java Collection, consider the specific requirements of your use case, such as whether you need an ordered or unordered collection, whether duplicates are allowed, and the performance requirements for adding, removing, and searching elements. You can then choose the appropriate interface and implementation based on these factors.