Java ArrayList
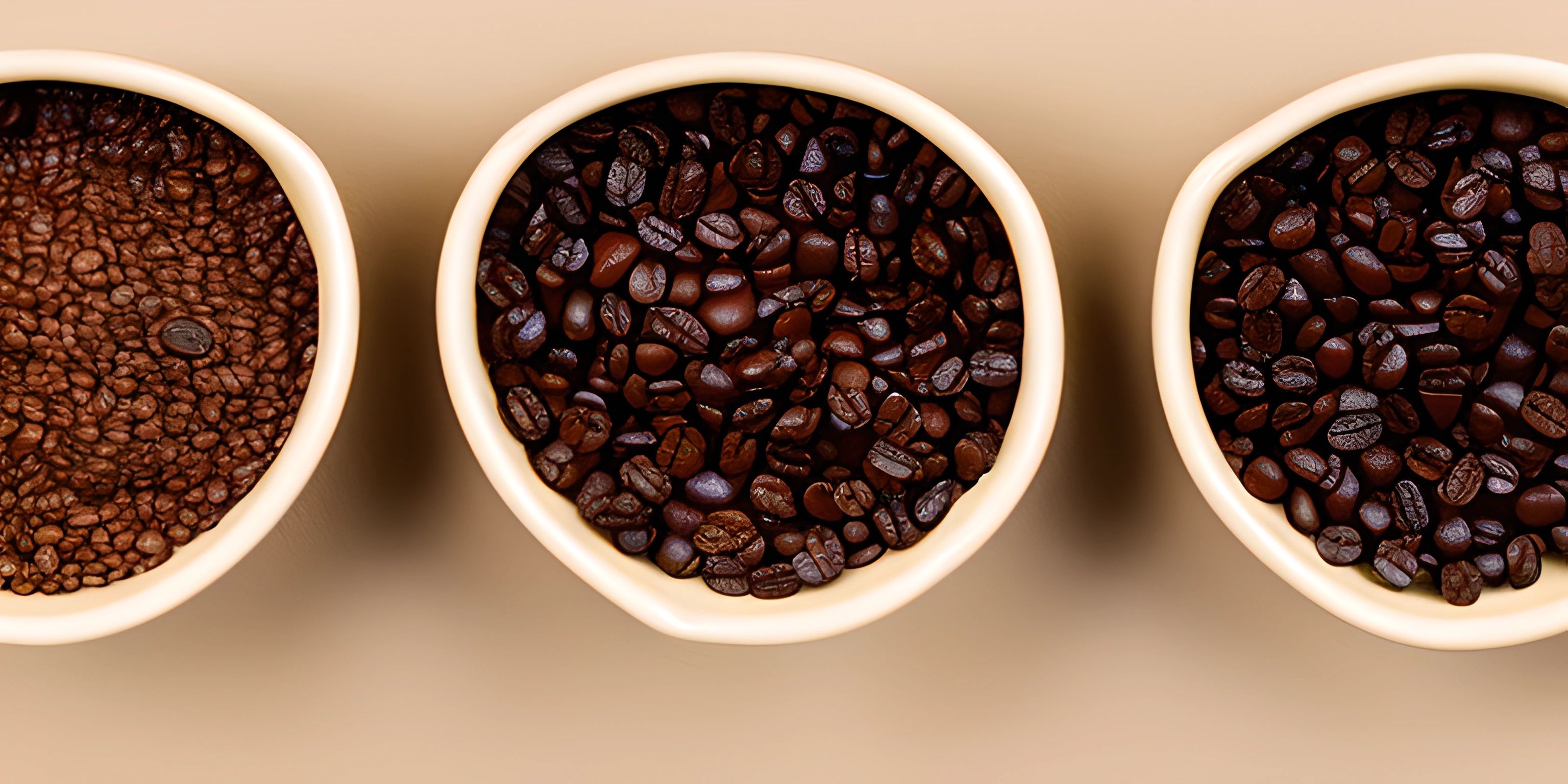
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java's ArrayList
is a part of the Collections Framework and is a dynamic array that can grow or shrink based on the elements we add or remove. Implemented as a resizable array, ArrayList
is one of the most versatile and widely used collection classes.
Creating an ArrayList
To create an ArrayList, you'll first need to import the java.util.ArrayList
package. Then, declare an ArrayList
object, specifying the data type of its elements using Generics.
Here's how to create an ArrayList
that stores integers:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<Integer> numbers = new ArrayList<>(); } }
Adding Elements
Adding elements to an ArrayList
is simple with the add()
method. Here's an example:
numbers.add(42); numbers.add(13); numbers.add(7);
Our ArrayList
now contains the integers 42
, 13
, and 7
.
Accessing Elements
To access elements in an ArrayList
, use the get()
method, providing the index of the desired element:
int firstNumber = numbers.get(0); // 42 int secondNumber = numbers.get(1); // 13
Keep in mind that ArrayList
indices are zero-based.
Modifying Elements
To update an element in an ArrayList
, use the set()
method, specifying both the index and the new value:
numbers.set(1, 99); // Replace 13 with 99
Our ArrayList
now contains 42
, 99
, and 7
.
Removing Elements
To remove an element from an ArrayList
, use the remove()
method, providing the index of the element to be removed:
numbers.remove(0); // Remove 42
Our ArrayList
now contains 99
and 7
.
Looping Through an ArrayList
To loop through an ArrayList
, use a standard for
loop or a for-each
loop:
// Standard for loop for (int i = 0; i < numbers.size(); i++) { System.out.println(numbers.get(i)); } // For-each loop for (int number : numbers) { System.out.println(number); }
Some Useful Methods
ArrayList
offers many useful methods. Here are a few examples:
size()
: Returns the number of elements in theArrayList
.isEmpty()
: Returnstrue
if theArrayList
is empty, andfalse
otherwise.contains(Object o)
: Returnstrue
if theArrayList
contains the specified element, andfalse
otherwise.indexOf(Object o)
: Returns the index of the first occurrence of the specified element, or-1
if the element is not present.clear()
: Removes all elements from theArrayList
.
ArrayList vs. Array
One might wonder when to use an ArrayList
over a normal array. In general, use ArrayList
when you need a dynamic, resizable data structure and use arrays when you have a fixed-size requirement. Arrays have a fixed length, while ArrayList
can grow or shrink as needed.
With ArrayList
, adding and removing elements is more convenient, and you can take advantage of built-in methods, making your code more readable and efficient.
Now that you're familiar with ArrayList
in Java, go ahead and experiment with various methods and use cases. Soon, you'll be an ArrayList
master!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is an ArrayList in Java?
An ArrayList in Java is a dynamic, resizable array implementation in the Java Collections Framework. Unlike regular arrays, ArrayLists can grow or shrink in size as elements are added or removed, making them highly flexible and efficient for storing and manipulating data.
How do I create an ArrayList in Java?
To create an ArrayList in Java, you need to import the java.util.ArrayList
package and declare an instance of it. Here's an example:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<>(); } }
How do I add elements to an ArrayList?
You can add elements to an ArrayList using the add()
method. Here's an example:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<>(); myList.add("Apple"); myList.add("Banana"); myList.add("Cherry"); System.out.println(myList); // Output: [Apple, Banana, Cherry] } }
How do I remove elements from an ArrayList?
To remove elements from an ArrayList, you can use the remove()
method with either the index or the object as a parameter. Here's an example:
import java.util.ArrayList; public class Main { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<>(); myList.add("Apple"); myList.add("Banana"); myList.add("Cherry"); myList.remove(1); // Removes the element at index 1 (Banana) System.out.println(myList); // Output: [Apple, Cherry] myList.remove("Cherry"); // Removes the object "Cherry" System.out.println(myList); // Output: [Apple] } }
How can I iterate through an ArrayList?
You can iterate through an ArrayList using a standard for
loop, an enhanced for
loop (also known as a "for-each" loop), or an iterator. Here are examples for each method:
import java.util.ArrayList; import java.util.Iterator; public class Main { public static void main(String[] args) { ArrayList<String> myList = new ArrayList<>(); myList.add("Apple"); myList.add("Banana"); myList.add("Cherry"); // Standard for loop for (int i = 0; i < myList.size(); i++) { System.out.println(myList.get(i)); } // Enhanced for loop (for-each) for (String fruit : myList) { System.out.println(fruit); } // Using iterator Iterator<String> iterator = myList.iterator(); while (iterator.hasNext()) { System.out.println(iterator.next()); } } }