Java Generics and Methods
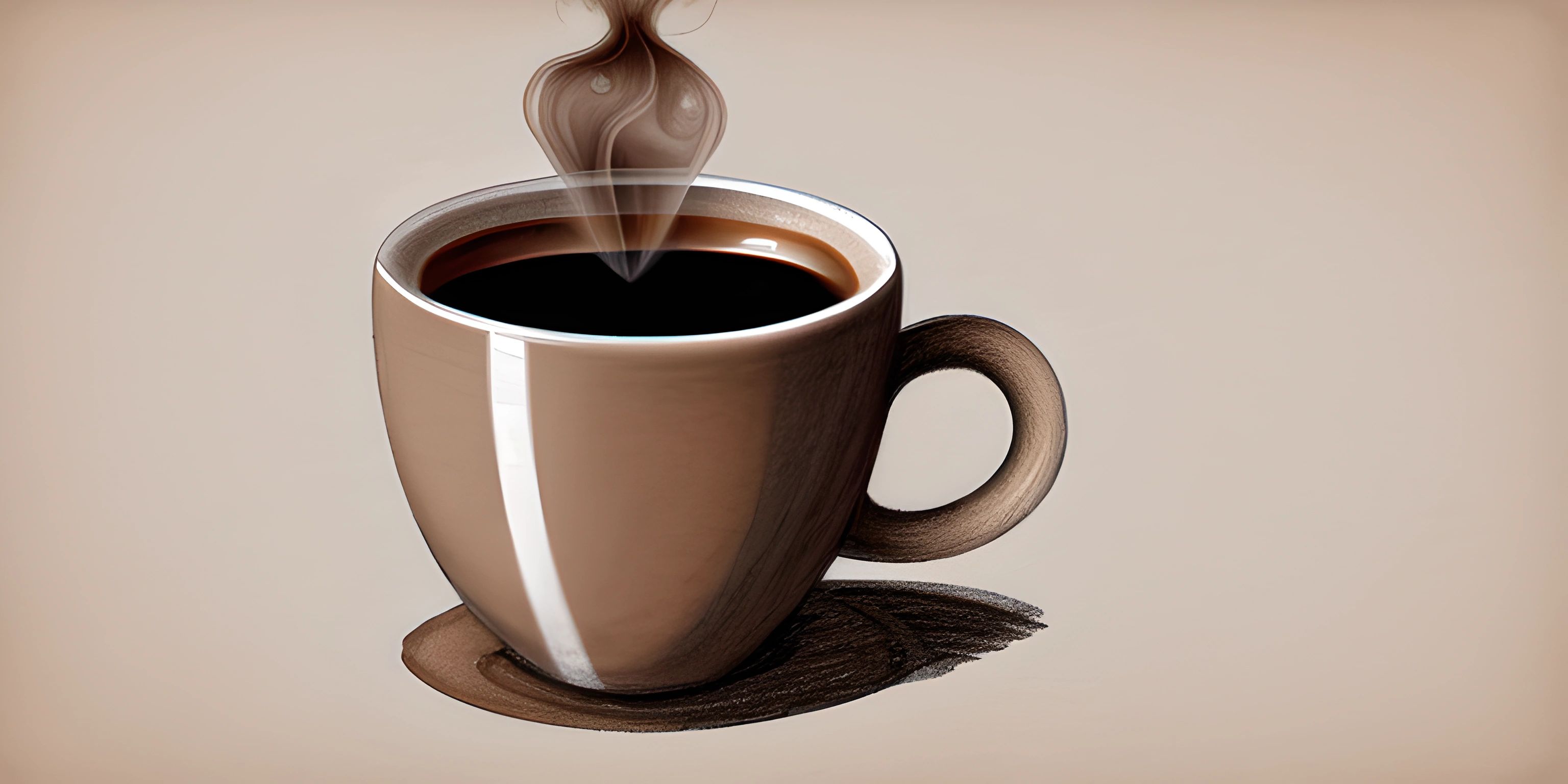
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java generics, like a Swiss Army knife, allow for greater flexibility and code reusability. With generics, you can write methods that work with different types without having to create multiple overloaded methods. Let's dive into the world of generic methods and see how they can enhance your Java programming skills.
What are Generics?
Generics are a powerful feature in Java that allows you to abstract type information from your classes and methods, making them more reusable and type-safe. Introduced in Java 5, generics enable you to create classes, interfaces, and methods that work with different data types while maintaining type safety.
Generic Methods
A generic method is a method that includes type parameters. These type parameters are placeholders for the actual data types that will be used when calling the method. To declare a generic method, you must specify a type variable within angle brackets <T>
before the method's return type.
Let's look at an example of a generic method:
public static <T> void printArray(T[] inputArray) { for (T element : inputArray) { System.out.printf("%s ", element); } System.out.println(); }
In this example, <T>
is the type parameter that represents a generic data type. The printArray
method accepts an array of any type and prints out its elements.
Using Generic Methods
To use a generic method, you simply call the method with the appropriate arguments. Java's compiler will automatically infer the type of the type parameter based on the arguments that you pass to the method. Here's an example of how to use the printArray
method from earlier:
public static void main(String[] args) { Integer[] intArray = {1, 2, 3, 4, 5}; Double[] doubleArray = {1.1, 2.2, 3.3, 4.4}; Character[] charArray = {'H', 'E', 'L', 'L', 'O'}; System.out.println("Array of integers:"); printArray(intArray); System.out.println("\nArray of doubles:"); printArray(doubleArray); System.out.println("\nArray of characters:"); printArray(charArray); }
In this example, we pass arrays of different types (Integer
, Double
, and Character
) to the printArray
method. Java's compiler automatically determines the type of <T>
based on the argument, and the method prints out the elements of each array.
Bounded Type Parameters
In some cases, you may want to restrict the types that can be used as arguments for a generic method. This can be achieved using bounded type parameters. To declare a bounded type parameter, you use the extends
keyword followed by the upper bound.
Here's an example of a generic method with a bounded type parameter:
public static <T extends Comparable<T>> T findMax(T x, T y) { return (x.compareTo(y) > 0) ? x : y; }
In this example, the findMax
method has a bounded type parameter, <T extends Comparable<T>>
. This means that the type T
must implement the Comparable
interface. The method compares two elements and returns the maximum of the two.
Using Bounded Type Parameters
To use a generic method with a bounded type parameter, you must pass arguments that satisfy the bound. Here's an example of using the findMax
method:
public static void main(String[] args) { System.out.println("Max of 3 and 4: " + findMax(3, 4)); System.out.println("Max of 'a' and 'b': " + findMax('a', 'b')); System.out.println("Max of \"hello\" and \"world\": " + findMax("hello", "world")); }
In this example, we pass arguments of different types (Integer
, Character
, and String
) to the findMax
method. All of these types implement the Comparable
interface, so they satisfy the bound, and the method returns the maximum value.
Conclusion
Java generics provide a powerful way to create flexible and reusable methods. Generic methods allow you to write a single method that works with different data types, simplifying your code and making it more maintainable. Bounded type parameters enable you to impose restrictions on the types that can be used with your generic methods, ensuring type safety and proper behavior. Embrace the versatility of generics and watch your Java programming prowess flourish!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Java generics and why are they important for methods?
Java generics are a language feature in Java that allows you to define and work with generic types, making your code more flexible and reusable. Generics are particularly important for methods because they enable you to create methods that can work with different types, without the need for excessive code duplication or casting.
How do I create a generic method in Java?
To create a generic method in Java, you need to add a type parameter enclosed in angle brackets < >
before the method's return type. The type parameter can then be used within the method's parameters and return type. Here's an example:
public static <T> void printArray(T[] array) { for (T item : array) { System.out.println(item); } }
In this example, the generic method printArray
accepts an array of any type T
and prints its elements.
Can I use multiple type parameters in a generic method?
Yes, you can use multiple type parameters in a generic method by separating them with commas inside the angle brackets < >
. Here's an example of a generic method with two type parameters:
public static <T, U> void printKeyValuePairs(Map<T, U> map) { for (Map.Entry<T, U> entry : map.entrySet()) { System.out.println(entry.getKey() + ": " + entry.getValue()); } }
In this example, the generic method printKeyValuePairs
accepts a Map
with key-value pairs of types T
and U
, respectively.
How can I restrict the types that can be used with my generic method?
You can restrict the types that can be used with your generic method by using bounded type parameters. Bounded type parameters are defined using the extends
keyword followed by the class or interface that the type must extend or implement. Here's an example:
public static <T extends Number> double sum(T[] numbers) { double sum = 0; for (T number : numbers) { sum += number.doubleValue(); } return sum; }
In this example, the generic method sum
accepts an array of T
objects, but the type T
must extend the Number
class.
Can I use wildcards in my generic method parameters?
Yes, you can use wildcards in your generic method parameters to make your method more flexible. Wildcards are represented with the ?
character and can be used in conjunction with the extends
or super
keywords to define upper or lower bounds. Here's an example:
public static void printNumbers(List<? extends Number> numbers) { for (Number number : numbers) { System.out.println(number); } }
In this example, the printNumbers
method accepts a List
of any type that extends Number
. This allows it to be called with a List<Integer>
, List<Double>
, or any other subclass of Number
.