Java Generics Introduction
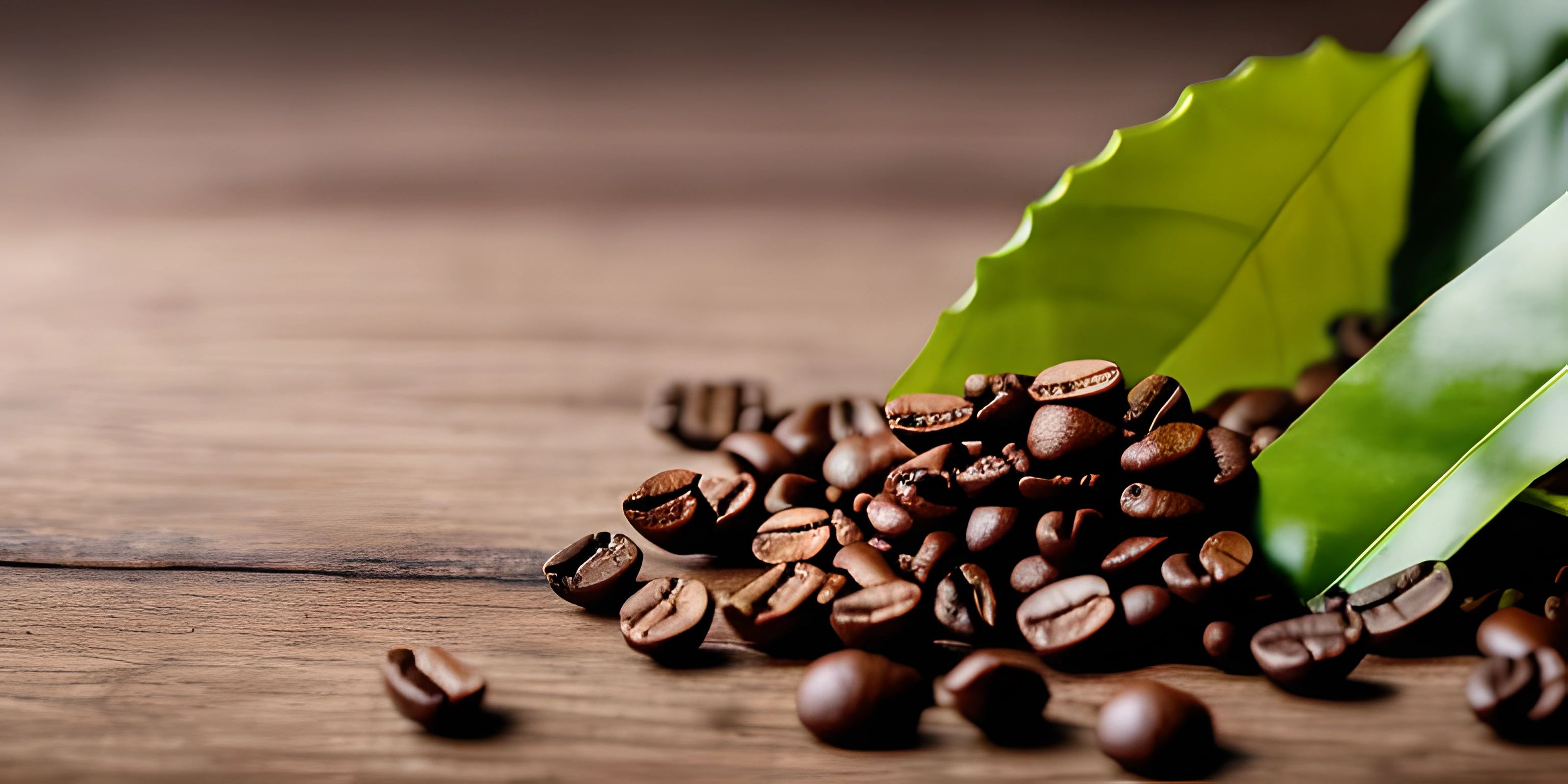
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the realm of Java programming, one concept stands out as a powerful tool for promoting type safety and code reusability: Generics. Put on your explorer's hat and let's venture into the world of Java Generics!
What are Java Generics?
Generics, introduced in Java 5, enable you to create classes, interfaces, and methods that work with different types while maintaining type safety. In other words, they allow you to write a single piece of code that can handle various data types without casting or potential type mismatches.
Generics are also known as parameterized types, because they use type parameters (enclosed in angle brackets <>
) to represent the type(s) you want to work with. Let's dive into an example to see how generics can improve our code.
Without Generics
Consider a simple Box
class without generics:
public class Box { private Object content; public void setContent(Object content) { this.content = content; } public Object getContent() { return content; } }
Here, the Box
class stores its content as an Object
. This means you can put any type of object in the box, but when you retrieve the content, you need to cast it back to its original type:
Box box = new Box(); box.setContent("Hello, Cratecode!"); String content = (String) box.getContent(); // Requires casting System.out.println(content);
The casting can be error-prone, as it is susceptible to ClassCastException
if the type is incorrect.
With Generics
Now let's rewrite the Box
class using generics:
public class Box<T> { private T content; public void setContent(T content) { this.content = content; } public T getContent() { return content; } }
Notice the <T>
after the class name? That's a type parameter, and it represents the type of content the box will hold. Now, when we use the Box
class, we specify the type we want to store:
Box<String> box = new Box<>(); box.setContent("Hello, Cratecode!"); String content = box.getContent(); // No casting required System.out.println(content);
No more casting, and if you try to put an object of the wrong type into the box, you'll get a compile-time error, increasing type safety.
Benefits of Java Generics
Java Generics bring several benefits to the table, including:
- Type safety: With generics, you catch type mismatch errors during compilation rather than at runtime, reducing the risk of runtime exceptions.
- Code reusability: Generics enable you to write a single piece of code that works with different types, reducing code duplication and increasing maintainability.
- Easier-to-read code: By specifying the types upfront, your code becomes clearer and more self-documenting.
Wrapping Up
Java Generics are a powerful feature that enhances type safety, code reusability, and readability. They are widely used in the Java standard library, especially in collections, which benefit greatly from the type safety and flexibility that generics provide. As you continue your Java journey, you'll undoubtedly encounter and appreciate the power of generics. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Java Generics and why should I use them?
Java Generics is a powerful feature that allows you to define and use generic classes, interfaces, and methods, enabling you to create type-safe and reusable code. By using Generics, you can ensure that the objects you work with have the correct types, reducing the risk of runtime errors and making your code more efficient.
How do I define a simple generic class in Java?
To define a generic class in Java, you use angle brackets <T>
following the class name, where T
represents a type parameter. Here's an example of a simple generic class:
public class Box<T> { private T content; public void setContent(T content) { this.content = content; } public T getContent() { return content; } }
In this example, T
stands for "Type" and can be replaced with any Java type when creating an instance of the Box
class.
How do I create an instance of a generic class with a specific type?
To create an instance of a generic class with a specific type, you replace the type parameter with the desired type when declaring the object. For example, to create a Box
object that can hold an Integer
value, you'd do the following:
Box<Integer> integerBox = new Box<>();
Can I use multiple type parameters in a generic class or method?
Yes, you can use multiple type parameters in a generic class or method by separating them with commas inside the angle brackets. Here's an example of a Pair
class with two type parameters, K
and V
:
public class Pair<K, V> { private K key; private V value; public Pair(K key, V value) { this.key = key; this.value = value; } // getters and setters }
How do I restrict the types allowed in a generic class or method?
You can restrict the types allowed in a generic class or method by using the extends
keyword followed by the class or interface that the type parameter must extend or implement. This is called a "bounded type parameter." For example, to restrict a generic class to only accept types that implement the Comparable
interface, you would do the following:
public class SortedPair<T extends Comparable<T>> { // class implementation }