Advanced Java Inheritance Concepts
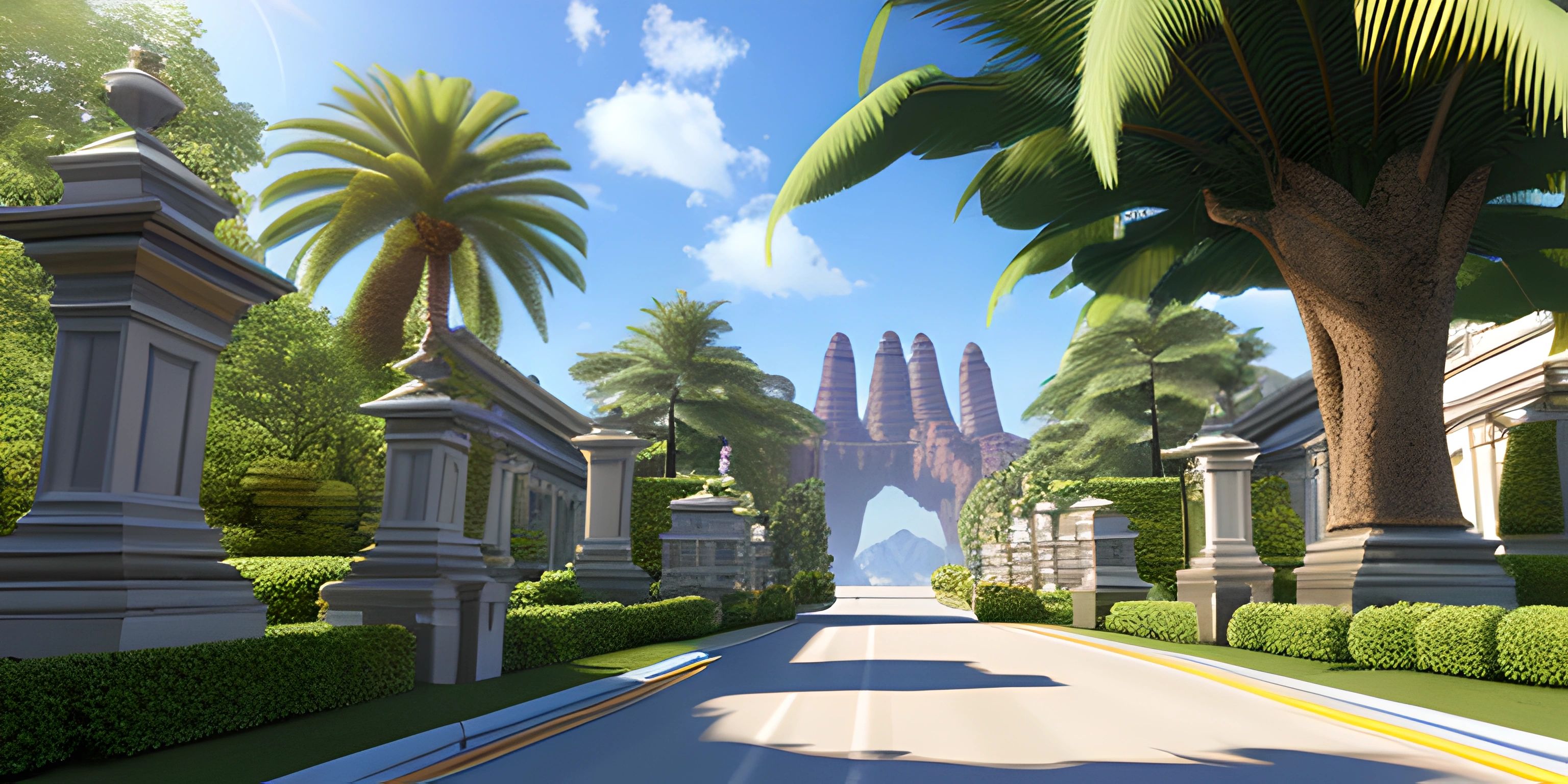
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In Java, inheritance is the core mechanism that allows a class to inherit properties and methods from another class. This helps us reuse and organize code efficiently. In this article, we'll look at some advanced concepts in Java inheritance, including the use of super
keyword and constructors.
The 'super' Keyword
The super
keyword in Java refers to the immediate parent class. It is commonly used when we want to call the parent class's constructor or methods. Using super
can help us avoid code duplication and make our code more maintainable.
Using 'super' to Call Parent Class Methods
When you override a method in a subclass, you're essentially replacing the parent class's implementation with your own. But what if you want to extend the functionality of the parent class method instead of completely replacing it? Here's where super
comes in. You can use super
to call the parent class method within the overridden method.
Here's an example:
class Animal { void makeSound() { System.out.println("The animal makes a sound"); } } class Dog extends Animal { void makeSound() { super.makeSound(); // Calling the parent class method System.out.println("The dog barks"); } } public class Main { public static void main(String[] args) { Dog dog = new Dog(); dog.makeSound(); // Output: The animal makes a sound \n The dog barks } }
In this example, we use super.makeSound()
within the makeSound
method of the Dog
class. This allows us to execute the parent class's makeSound
method before adding the additional functionality of printing "The dog barks".
Using 'super' with Constructors
Just like methods, you can use super
to call a parent class's constructor. By default, Java will call the no-argument constructor of the parent class if you don't explicitly call it using super
. However, if you want to call a specific constructor from the parent class, you can use super
to do so.
Here's an example:
class Animal { String name; Animal(String name) { this.name = name; } } class Dog extends Animal { String breed; Dog(String name, String breed) { super(name); // Calling the parent class constructor this.breed = breed; } } public class Main { public static void main(String[] args) { Dog dog = new Dog("Buddy", "Golden Retriever"); System.out.println("Dog's name: " + dog.name + ", breed: " + dog.breed); // Output: Dog's name: Buddy, breed: Golden Retriever } }
In this example, we use super(name)
within the Dog
class constructor to call the Animal
class constructor that takes a String
as an argument.
This advanced understanding of Java inheritance and the use of super
keyword can help you write more efficient and organized code. Keep experimenting and mastering these concepts to become a better Java programmer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
How do I use the 'super' keyword in Java inheritance?
The 'super' keyword in Java is used to refer to the parent class of a derived class. It can be used to access the methods and attributes of the parent class or to call the parent class's constructor. Here's an example:
class Parent { int num; Parent(int n) { num = n; } } class Child extends Parent { int num; Child(int n1, int n2) { super(n1); // Calls the constructor of the parent class num = n2; } void display() { System.out.println("Parent class num: " + super.num); System.out.println("Child class num: " + num); } } public class Main { public static void main(String[] args) { Child obj = new Child(10, 20); obj.display(); } }
In this example, the 'super' keyword is used to call the parent class's constructor and access its 'num' attribute.
What happens if I don't explicitly call the parent class constructor in a derived class?
If you don't explicitly call the parent class constructor in a derived class, Java will automatically call the no-argument constructor of the parent class. Note that this will only work if the parent class has a no-argument constructor. If the parent class doesn't have a no-argument constructor, you must call one of the parent class's constructors explicitly using the 'super' keyword.
Can I use 'super' to call a method from the parent class that has been overridden in the child class?
Yes, you can use 'super' to call a method from the parent class that has been overridden in the child class. This is useful when you want to extend the functionality of a method in the parent class rather than completely replacing it. Here's an example:
class Parent { void display() { System.out.println("Parent class method"); } } class Child extends Parent { @Override void display() { super.display(); // Calls the display method of the parent class System.out.println("Child class method"); } } public class Main { public static void main(String[] args) { Child obj = new Child(); obj.display(); } }
In this example, the 'display' method in the child class calls the 'display' method from the parent class using 'super', before adding its own additional functionality.