Java Encapsulation
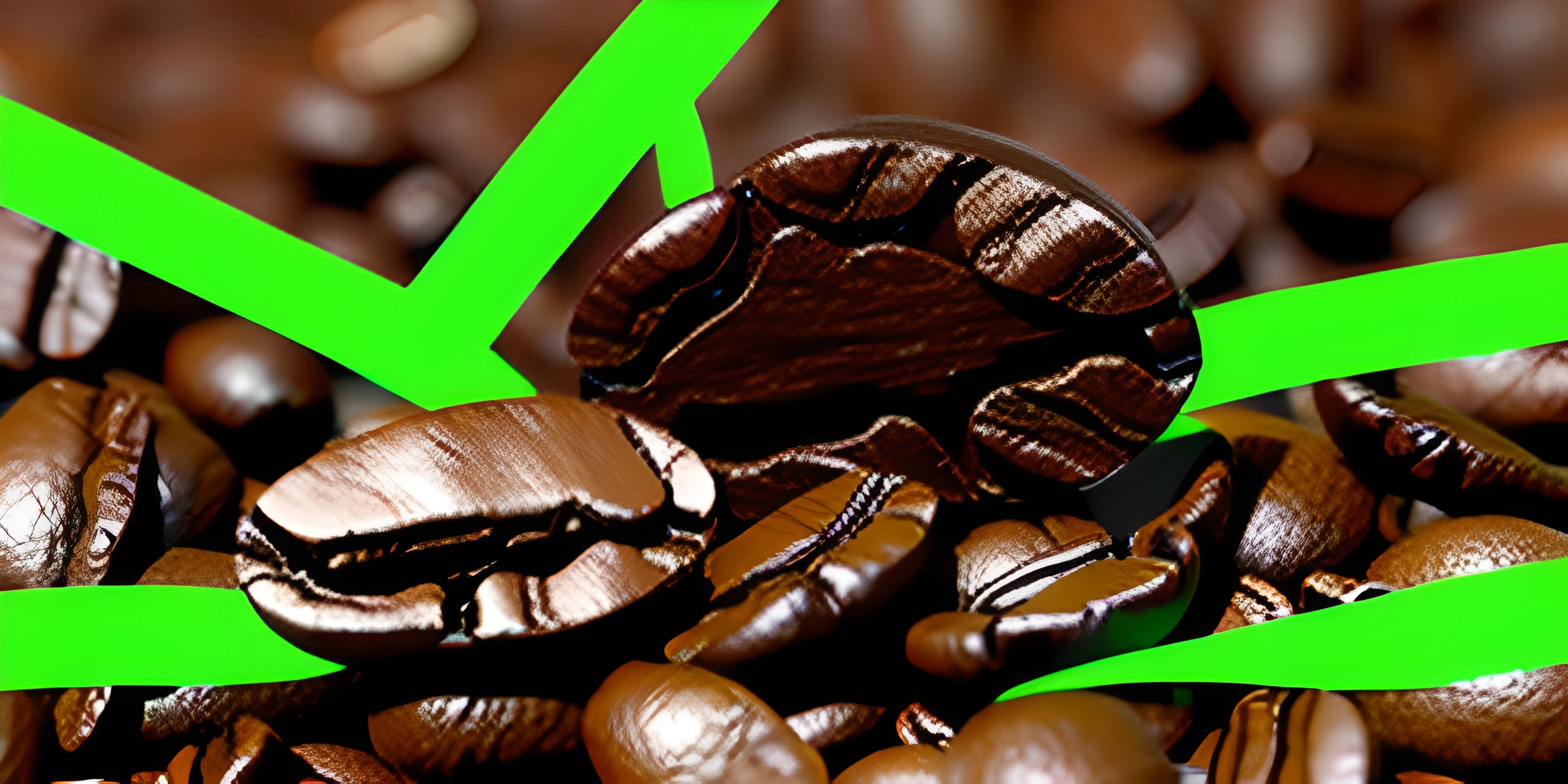
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Encapsulation is one of the four fundamental principles of object-oriented programming (OOP). It's all about wrapping up data (variables) and code (methods) together into a single unit known as a class. This not only helps to keep your code organized but also provides a layer of security by controlling access to data and methods.
Access Modifiers: Public, Private, and Protected
In Java, encapsulation is achieved using access modifiers. These are keywords that determine the visibility and accessibility of class members (variables and methods). Java provides three main access modifiers:
- Public: Members declared as public are accessible from any class within the project.
- Private: Members declared as private are only accessible within the class they are declared in.
- Protected: Members declared as protected are accessible within the same package and also by subclasses in other packages.
By setting the right access modifier for your class members, you can control how they can be accessed from other classes, thus encapsulating the data and methods.
Getters and Setters
The most common way of implementing encapsulation in Java is through the use of getters and setters. Getters are methods that return the value of a private variable, while setters are methods that set or modify the value of a private variable.
Here's a simple Java class that demonstrates encapsulation using getters and setters:
public class Employee { private String name; private int age; // Getter for the name variable public String getName() { return name; } // Setter for the name variable public void setName(String newName) { name = newName; } // Getter for the age variable public int getAge() { return age; } // Setter for the age variable public void setAge(int newAge) { age = newAge; } }
In this example, the name
and age
variables are encapsulated using the private access modifier. This means they cannot be accessed directly from outside the Employee
class. Instead, we provide public getters and setters to allow controlled access to these variables from other classes.
Benefits of Encapsulation
Encapsulation has several benefits, which include:
- Controlled access: By encapsulating data and methods, you can control how they are accessed and modified from other classes, ensuring the integrity of your code.
- Flexibility: By using getters and setters, you can easily change the underlying implementation of a class without affecting any code that uses the class.
- Maintainability: Encapsulation promotes a clear separation of concerns, making it easier to understand, maintain, and extend your code.
Conclusion
Encapsulation is an essential concept in Java and object-oriented programming. By understanding and implementing encapsulation using access modifiers and getters and setters, you can create more robust, flexible, and maintainable code. So, go ahead and wrap up your code like a precious gift, and enjoy the benefits it brings!