Understanding Java Methods and Their Significance
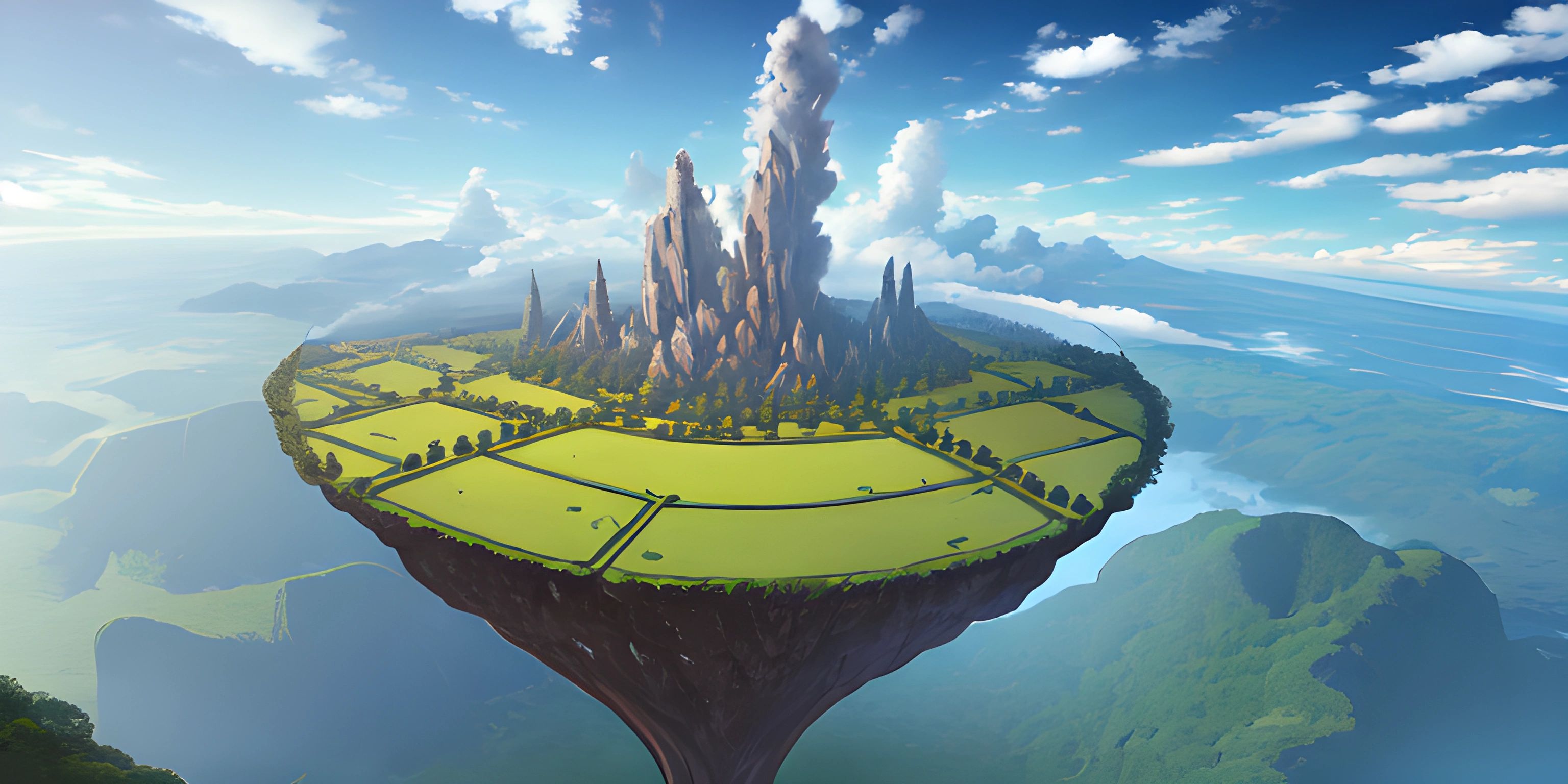
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're diving into the world of Java programming, one of the first and most important concepts you'll encounter is methods. In this article, we'll explore what Java methods are, their syntax, and why they're critical in building modular, readable, and efficient code.
What are Java Methods?
Java methods, sometimes referred to as functions, are blocks of code that perform a specific task. They are used to organize and break down complex problems into smaller, manageable parts. Methods can be called (or invoked) from other parts of your code, allowing you to reuse the same piece of code multiple times without duplicating it.
Here's an example of a simple Java method that adds two numbers and returns the result:
public static int add(int a, int b) { return a + b; }
Java Method Syntax
A Java method consists of several components:
- Access modifier: Determines the visibility of the method (e.g.,
public
,private
,protected
). - Optional modifiers: Additional information about the method, such as
static
,final
, orabstract
. - Return type: The data type of the value that the method returns (e.g.,
int
,double
,String
). Usevoid
if the method doesn't return a value. - Method name: A descriptive name for the method, following Java naming conventions.
- Parameters: A list of variables enclosed in parentheses, which are used to pass values into the method.
- Method body: A block of code enclosed in curly braces
{ }
that contains the instructions to be executed.
Here's a breakdown of the example method:
public static int add(int a, int b) { return a + b; }
- Access modifier:
public
- Optional modifier:
static
- Return type:
int
- Method name:
add
- Parameters:
(int a, int b)
- Method body:
{ return a + b; }
Why are Java Methods Important?
Methods in Java serve several crucial purposes:
- Modularity: Methods help break down complex problems into smaller, more manageable pieces. This makes your code easier to understand and maintain.
- Reusability: Methods allow you to reuse the same piece of code multiple times without duplicating it, making your code more efficient and easier to update.
- Readability: Methods provide a way to give descriptive names to specific tasks, making your code more readable and understandable for both yourself and others.
- Abstraction: Methods allow you to hide the implementation details of a task, exposing only the necessary inputs and outputs. This can make your code more flexible and easier to change.
In Conclusion
Java methods are fundamental building blocks of any Java program. They provide modularity, reusability, readability, and abstraction, allowing you to create efficient, maintainable, and user-friendly code. By understanding Java methods and their significance, you'll be well on your way to becoming a skilled Java programmer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Java methods?
Java methods, also known as functions, are blocks of code that perform a specific task. They help organize and break down complex problems into smaller, more manageable parts and can be called from other parts of the code, allowing for code reusability.
What are the components of a Java method?
A Java method consists of an access modifier, optional modifiers, a return type, a method name, parameters, and a method body.
Why are Java methods important?
Java methods are important because they provide modularity, reusability, readability, and abstraction, making your code more efficient, maintainable, and user-friendly. They also help break down complex problems into smaller, more manageable parts.